NoCScheduler Class Reference
#include <noc_scheduler.h>
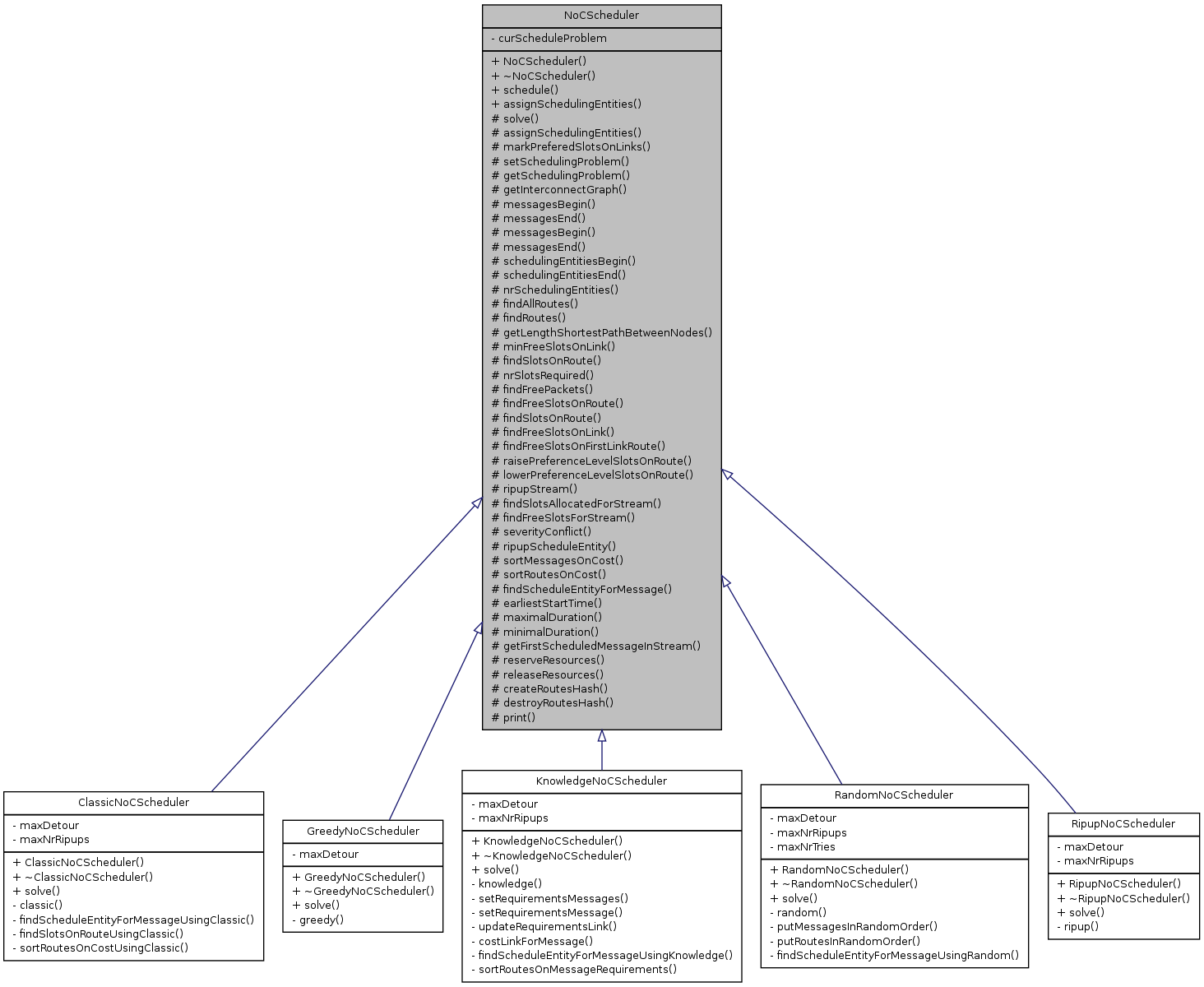
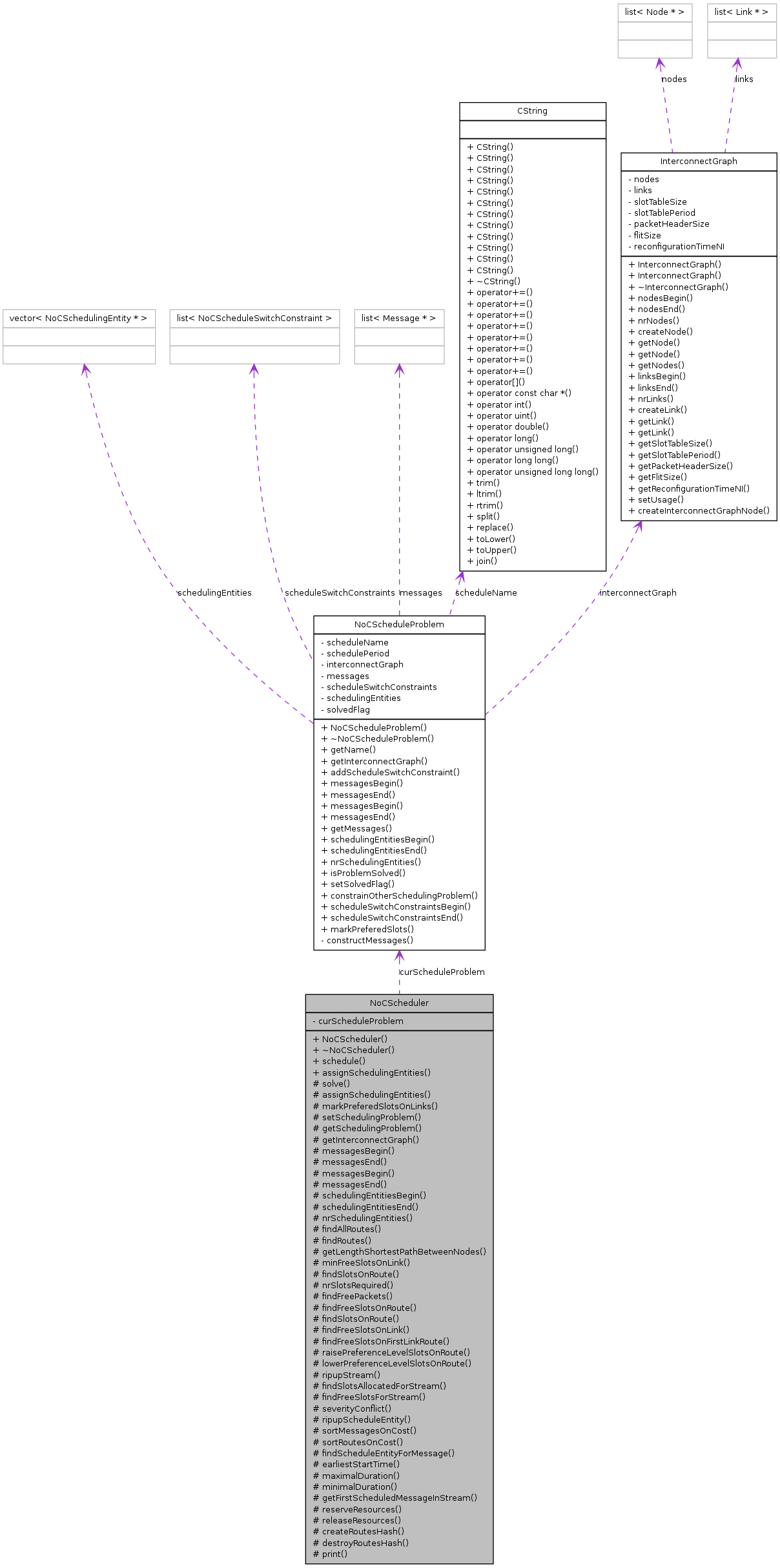
Constructor & Destructor Documentation
NoCScheduler::NoCScheduler | ( | ) | [inline] |
virtual NoCScheduler::~NoCScheduler | ( | ) | [inline, virtual] |
Member Function Documentation
void NoCScheduler::assignSchedulingEntities | ( | SetOfNoCScheduleProblems & | problems, | |
CNode * | networkMappingNode | |||
) |
assignSchedulingEntities () Load scheduling entities for the scheduling problems from XML.
References CGetAttribute(), CGetChildNode(), CNextNode(), NoCScheduleProblem::getName(), SetOfNoCScheduleProblems::scheduleProblemsBegin(), SetOfNoCScheduleProblems::scheduleProblemsEnd(), and setSchedulingProblem().
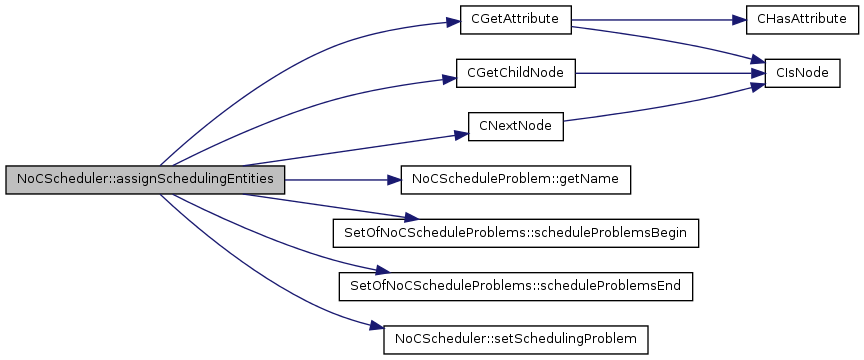
void NoCScheduler::assignSchedulingEntities | ( | CNode * | messagesNode | ) | [protected] |
assignSchedulingEntities () Load scheduling entities for the current scheduling problems from XML.
References Route::appendLink(), CGetAttribute(), CGetChildNode(), CNextNode(), getInterconnectGraph(), InterconnectGraph::getLink(), InterconnectGraph::getSlotTableSize(), messagesBegin(), messagesEnd(), reserveResources(), NoCSchedulingEntity::setDuration(), NoCSchedulingEntity::setRoute(), Message::setSchedulingEntity(), NoCSchedulingEntity::setSlotReservations(), NoCSchedulingEntity::setStartTime(), and CString::split().
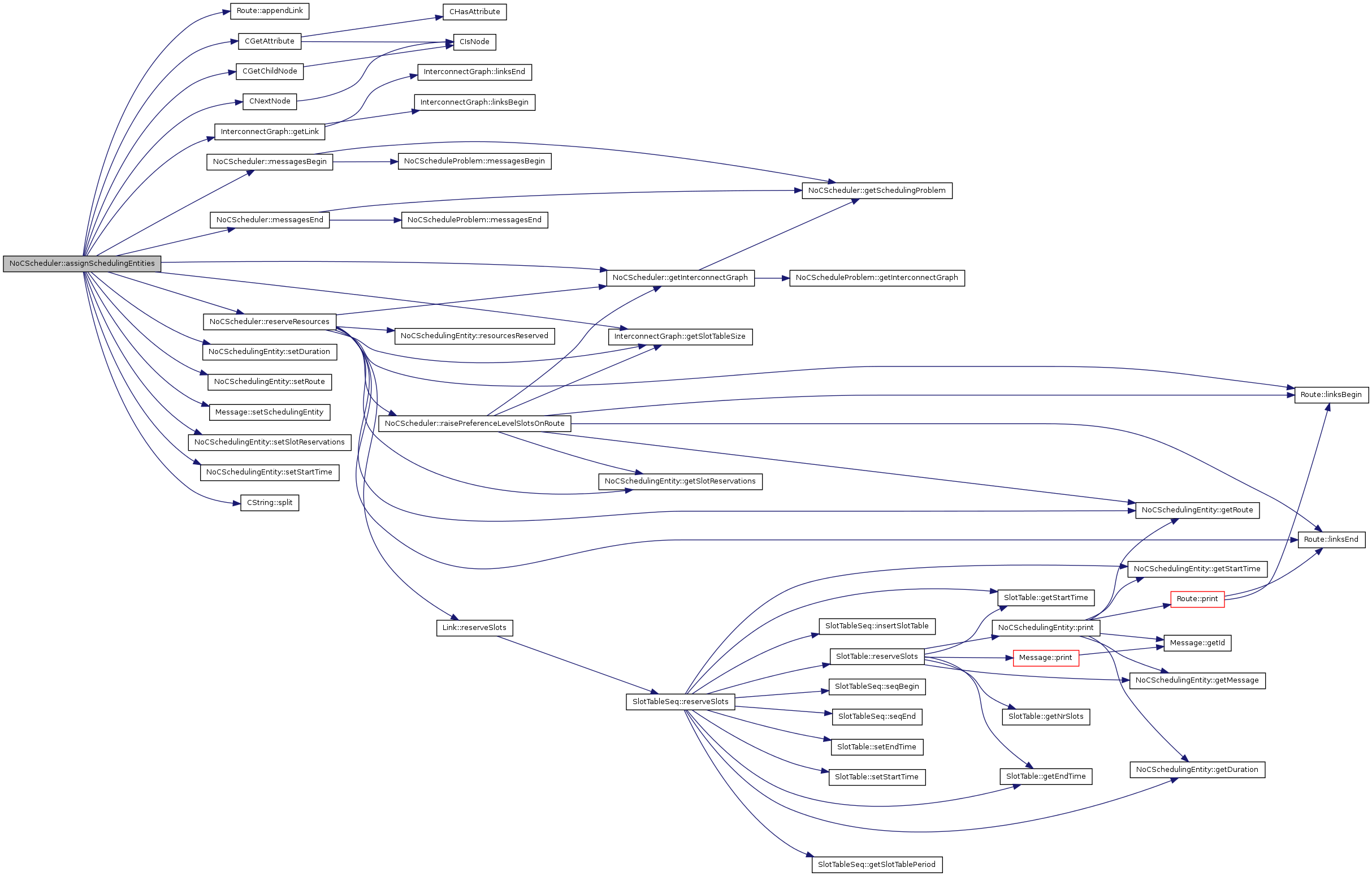
void NoCScheduler::createRoutesHash | ( | const CSize | maxDetour | ) | [protected] |
void NoCScheduler::destroyRoutesHash | ( | ) | [protected] |
earliestStartTime () The function returns the earliest start time for the message m. The earliest start time is always later then the latest end time of messages earlier in the same stream.
References NoCSchedulingEntity::getDuration(), Message::getPreviousMessageInStream(), Message::getSchedulingEntity(), NoCSchedulingEntity::getStartTime(), and Message::getStartTime().
Referenced by findScheduleEntityForMessage(), ClassicNoCScheduler::findScheduleEntityForMessageUsingClassic(), KnowledgeNoCScheduler::findScheduleEntityForMessageUsingKnowledge(), and RandomNoCScheduler::findScheduleEntityForMessageUsingRandom().
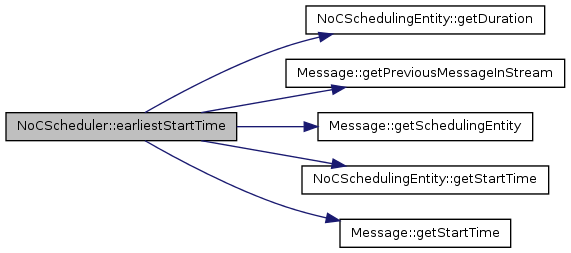
void NoCScheduler::findAllRoutes | ( | const Node * | src, | |
const Node * | dst, | |||
const CSize | maxDetour, | |||
bool | exact, | |||
Routes & | routes | |||
) | [protected] |
findAllRoutes () The function finds all routes between the given source and destination node with the specified maximum detour.
References findRoutes(), and getLengthShortestPathBetweenNodes().
Referenced by findScheduleEntityForMessage(), ClassicNoCScheduler::findScheduleEntityForMessageUsingClassic(), KnowledgeNoCScheduler::findScheduleEntityForMessageUsingKnowledge(), RandomNoCScheduler::findScheduleEntityForMessageUsingRandom(), ripupScheduleEntity(), and KnowledgeNoCScheduler::setRequirementsMessage().
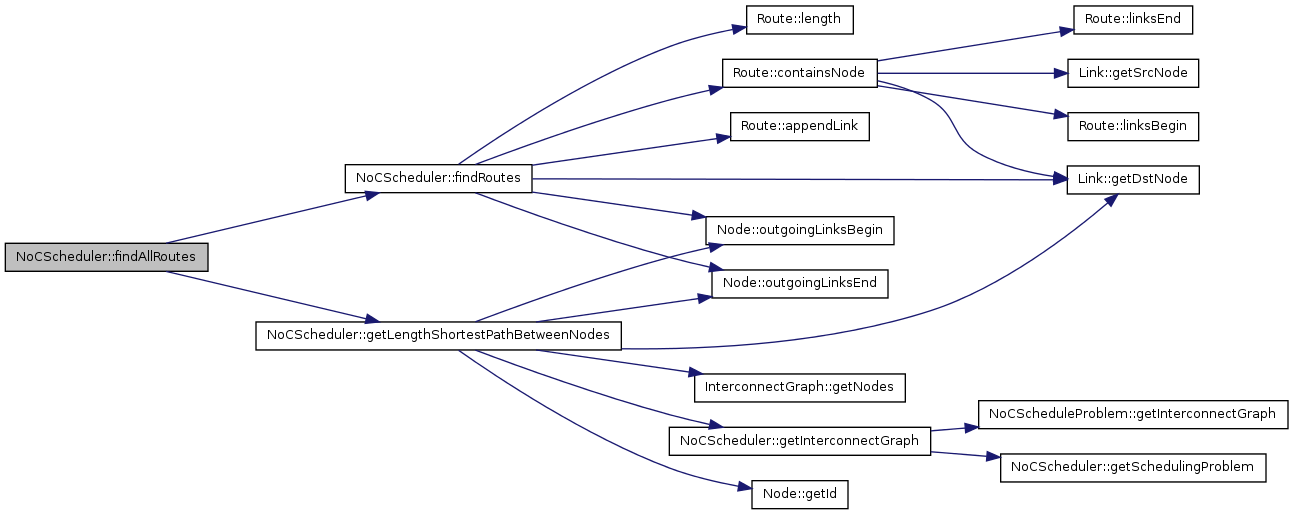
void NoCScheduler::findFreePackets | ( | NoCSchedulingEntity * | e, | |
SlotReservations & | slotsRoute, | |||
Packets & | packets | |||
) | [protected] |
findFreePackets () The function locates all available packets within the timing constraints and available slots on the route (slotsRoute).
References Packet::endTime, NoCSchedulingEntity::getDuration(), getInterconnectGraph(), InterconnectGraph::getSlotTableSize(), NoCSchedulingEntity::getStartTime(), Packet::nrSlots, and Packet::startTime.
Referenced by findSlotsOnRoute(), and ClassicNoCScheduler::findSlotsOnRouteUsingClassic().
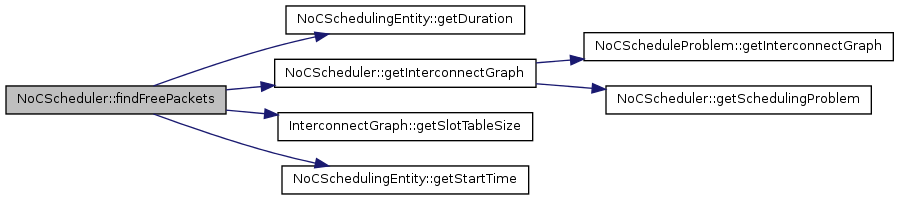
void NoCScheduler::findFreeSlotsForStream | ( | Route * | r, | |
SlotReservations & | slotsRoute | |||
) | [protected] |
findFreeSlotsForStream () The function locates all slots which are not used by any stream at any moment in time along the complete route r.
References getInterconnectGraph(), InterconnectGraph::getSlotTableSize(), SlotTable::isSlotFree(), Route::linksBegin(), Route::linksEnd(), Link::slotTableSeqBegin(), and Link::slotTableSeqEnd().
Referenced by ClassicNoCScheduler::findSlotsOnRouteUsingClassic().
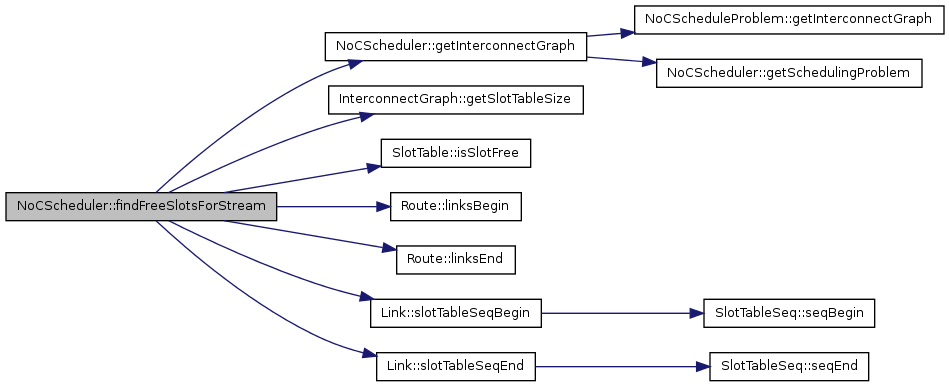
SlotReservations NoCScheduler::findFreeSlotsOnFirstLinkRoute | ( | const Route * | r, | |
const TTime | startTime, | |||
const TTime | duration | |||
) | [protected] |
findFreeSlotsOnFirstLinkRoute () The function computes all slots which are available in the first link of a route within the given time bounds, taking into account the time needed to reconfigure the NI. These slots are marked as true within the slot reservations.
References SlotTable::begin(), SlotTable::end(), SlotTable::getEndTime(), getInterconnectGraph(), InterconnectGraph::getReconfigurationTimeNI(), NoCSchedulingEntity::getRoute(), SlotTable::getSlotReservations(), InterconnectGraph::getSlotTablePeriod(), InterconnectGraph::getSlotTableSize(), SlotTable::getStartTime(), Route::linksBegin(), Link::slotTableSeqBegin(), and Link::slotTableSeqEnd().
Referenced by findFreeSlotsOnRoute().
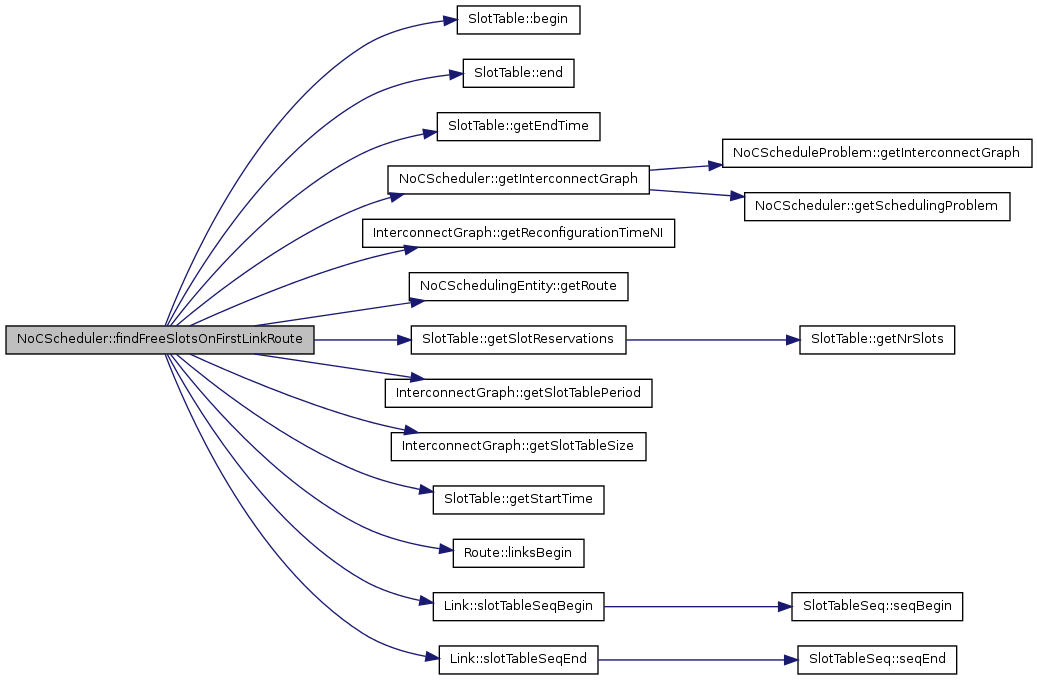
SlotReservations NoCScheduler::findFreeSlotsOnLink | ( | const Link * | l, | |
const TTime | startTime, | |||
const TTime | duration | |||
) | [protected] |
findFreeSlotsOnLink () The function computes all slots which are available in a link within the given time bounds. These slots are marked as true within the slot reservations.
References SlotTable::getEndTime(), getInterconnectGraph(), SlotTable::getSlotReservations(), InterconnectGraph::getSlotTablePeriod(), InterconnectGraph::getSlotTableSize(), SlotTable::getStartTime(), Link::slotTableSeqBegin(), and Link::slotTableSeqEnd().
Referenced by findFreeSlotsOnRoute().
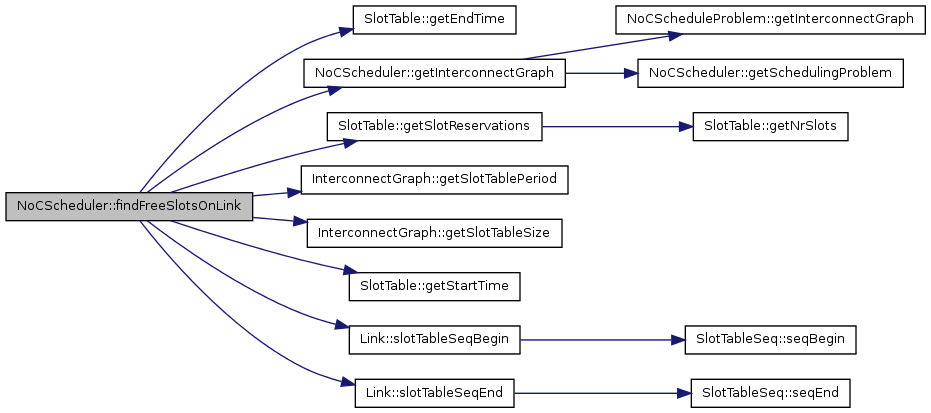
SlotReservations NoCScheduler::findFreeSlotsOnRoute | ( | const Route * | r, | |
const TTime | startTime, | |||
const TTime | duration | |||
) | [protected] |
findFreeSlotsOnRoute () The function computes all slots which are available in consecutive links along a route within the given time bounds. Available slots are marked with true in the slot reservations.
References findFreeSlotsOnFirstLinkRoute(), findFreeSlotsOnLink(), getInterconnectGraph(), InterconnectGraph::getSlotTableSize(), Route::linksBegin(), and Route::linksEnd().
Referenced by findSlotsOnRoute().
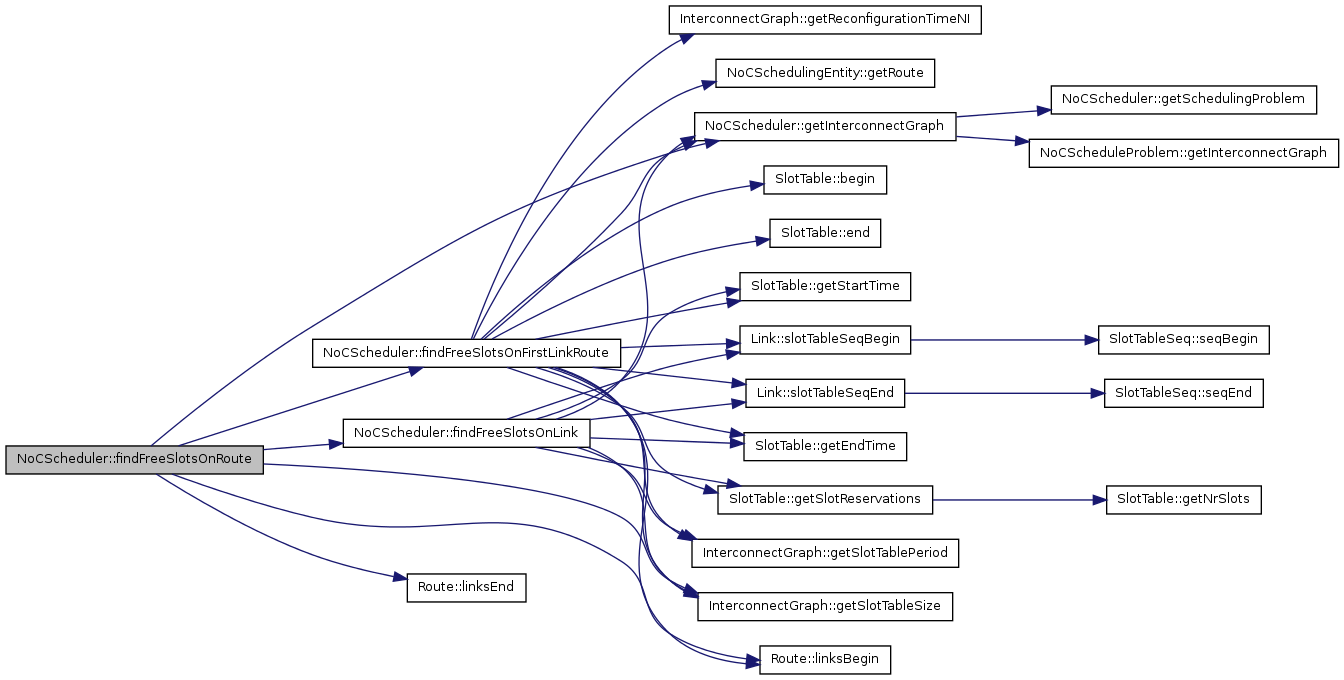
void NoCScheduler::findRoutes | ( | const Node * | src, | |
const Node * | dst, | |||
const CSize | minLength, | |||
const CSize | maxLength, | |||
Route & | route, | |||
Routes & | routes | |||
) | [protected] |
findAllRoutes () The function finds all routes between the given source and destination node within the specified minimum and maximum length.
References Route::appendLink(), Route::containsNode(), Link::getDstNode(), Route::length(), Node::outgoingLinksBegin(), and Node::outgoingLinksEnd().
Referenced by findAllRoutes().
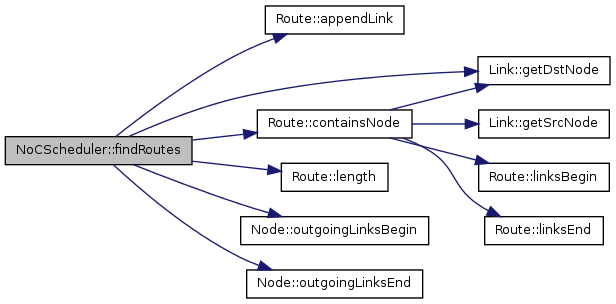
findScheduleEntityForMessage () The function tries to find a schedule entity for the message. On success it returns true and allocates the entity on the NoC. Else it returns false and no resources are claimed.
References earliestStartTime(), findAllRoutes(), findSlotsOnRoute(), Message::getDstNodeId(), NoCSchedulingEntity::getDuration(), getInterconnectGraph(), getLengthShortestPathBetweenNodes(), InterconnectGraph::getNode(), InterconnectGraph::getSlotTableSize(), Message::getSrcNodeId(), NoCSchedulingEntity::getStartTime(), Route::length(), maximalDuration(), minimalDuration(), reserveResources(), NoCSchedulingEntity::setDuration(), NoCSchedulingEntity::setRoute(), Message::setSchedulingEntity(), NoCSchedulingEntity::setSlotReservations(), NoCSchedulingEntity::setStartTime(), and sortRoutesOnCost().
Referenced by GreedyNoCScheduler::greedy(), and RipupNoCScheduler::ripup().
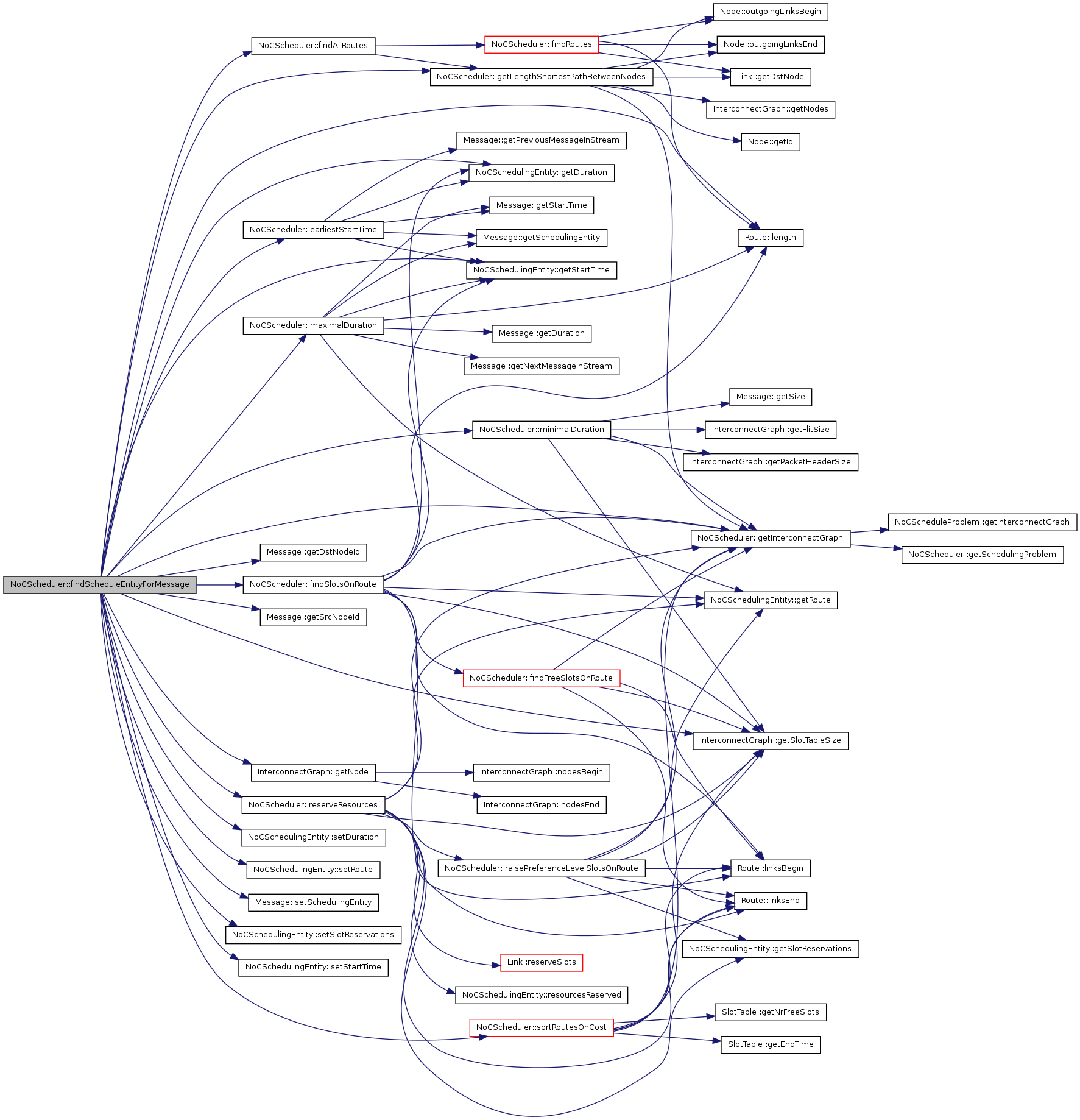
void NoCScheduler::findSlotsAllocatedForStream | ( | NoCSchedulingEntity * | e, | |
SlotReservations & | slotsAllocated | |||
) | [protected] |
findSlotsAllocatedForStream () The function locates all slots which are used to sent messages from the same stream starting at the source link.
References getInterconnectGraph(), NoCSchedulingEntity::getMessage(), NoCSchedulingEntity::getRoute(), InterconnectGraph::getSlotTableSize(), Message::getStreamId(), Route::linksBegin(), Link::slotTableSeqBegin(), and Link::slotTableSeqEnd().
Referenced by ClassicNoCScheduler::findSlotsOnRouteUsingClassic().
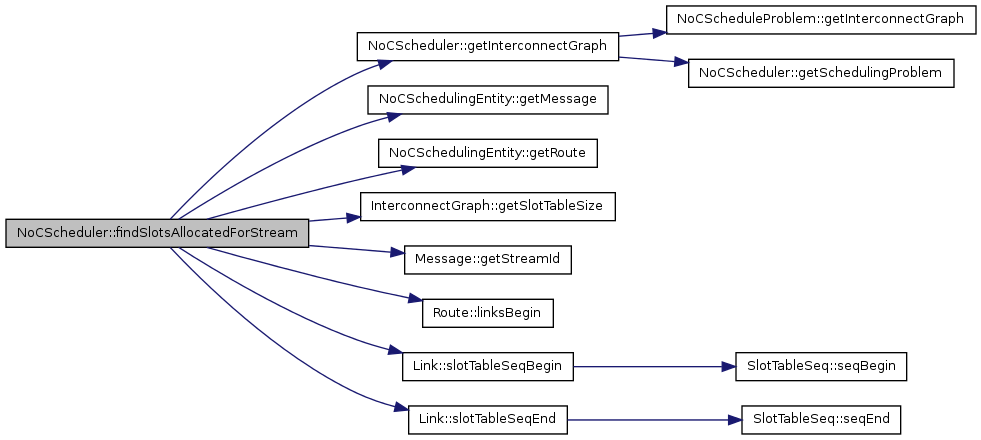
bool NoCScheduler::findSlotsOnRoute | ( | NoCSchedulingEntity * | e, | |
SlotReservations & | s | |||
) | [protected] |
findSlotsOnRoute () The function looks for a set of slots which can be reserved along the route such that flits entering a router leave it in the next slot and there is enough space to transmit both the data and the packatization headers. On success, the slot reservations for the first link of the route are stored in s and the function returns true. On failure, the function returns false. The function tries to minimize the number of packets used.
References findFreeSlotsOnRoute(), NoCSchedulingEntity::getDuration(), getInterconnectGraph(), NoCSchedulingEntity::getRoute(), InterconnectGraph::getSlotTableSize(), NoCSchedulingEntity::getStartTime(), Route::length(), Route::linksBegin(), Route::linksEnd(), and Link::preferredSlots.
Referenced by findScheduleEntityForMessage(), KnowledgeNoCScheduler::findScheduleEntityForMessageUsingKnowledge(), and RandomNoCScheduler::findScheduleEntityForMessageUsingRandom().
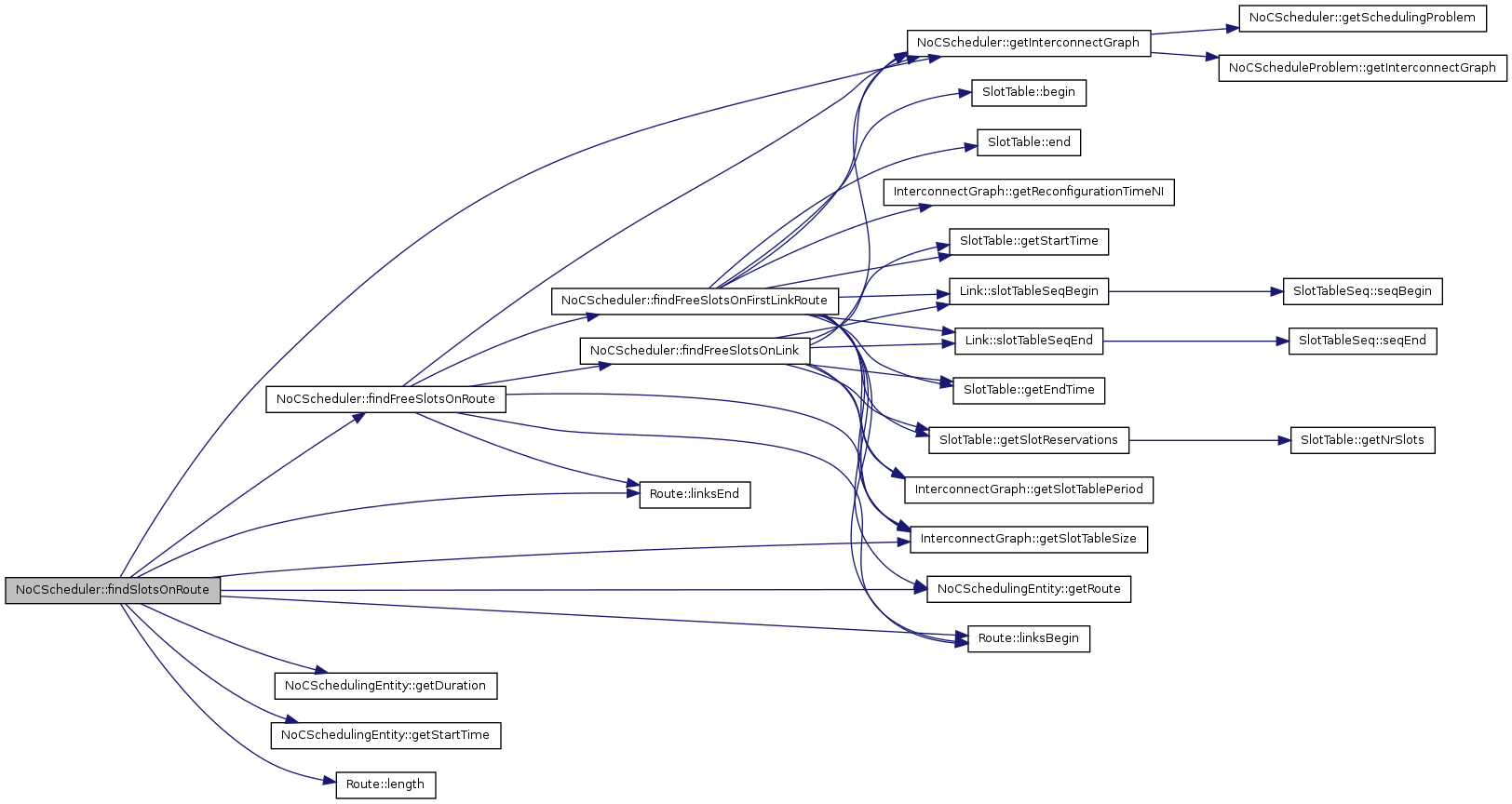
bool NoCScheduler::findSlotsOnRoute | ( | NoCSchedulingEntity * | e, | |
SlotReservations & | slotsForPackets, | |||
SlotReservations & | s | |||
) | [protected] |
findSlotsOnRoute () The function looks for a set of slots which can be reserved along the route such that flits entering a router leave it in the next slot and there is enough space to transmit both the data and the packatization headers. On success, the slot reservations for the first link of the route are stored in s and the function returns true. On failure, the function returns false. The function tries to minimize the number of packets used. It only uses slots from the slots marked in slotsForPackets. The caller of the function must guarantee that those slots are actually available for sending the data.
References Packet::endTime, findFreePackets(), findFreeSlotsOnRoute(), NoCSchedulingEntity::getDuration(), getInterconnectGraph(), NoCSchedulingEntity::getMessage(), NoCSchedulingEntity::getRoute(), Message::getSize(), InterconnectGraph::getSlotTableSize(), NoCSchedulingEntity::getStartTime(), Packet::loop, Packet::nrSlots, nrSlotsRequired(), and Packet::startTime.
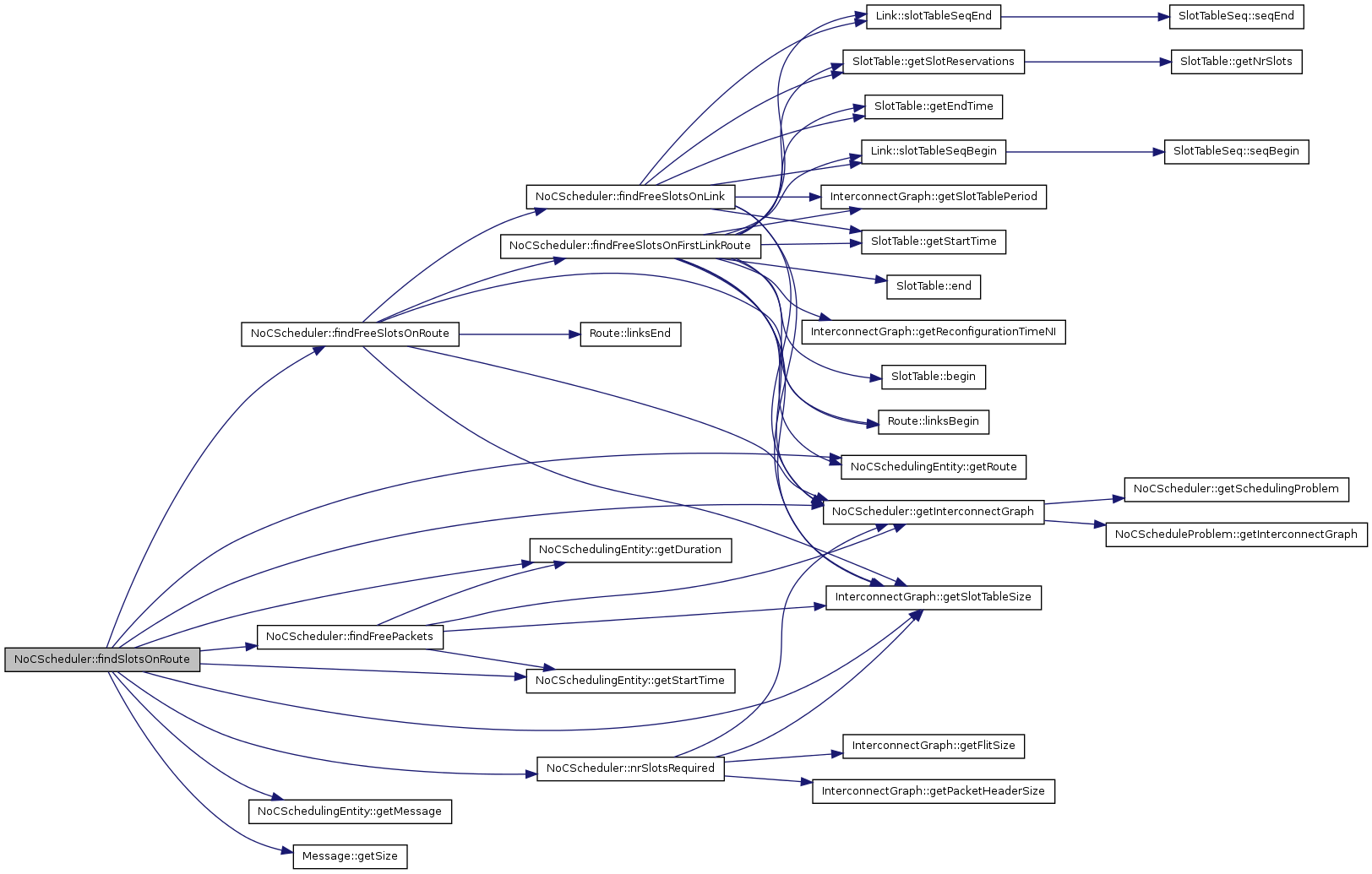
NoCSchedulingEntity * NoCScheduler::getFirstScheduledMessageInStream | ( | Message * | m | ) | [protected] |
getFirstScheduledMessageInStream () The function returns a pointer to the scheduling entity of the first message in the stream which is scheduled. Or NULL if no such message exists.
References Message::getNextMessageInStream(), Message::getPreviousMessageInStream(), and Message::getSchedulingEntity().
Referenced by ClassicNoCScheduler::findScheduleEntityForMessageUsingClassic().
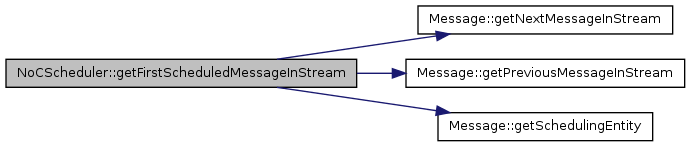
InterconnectGraph* NoCScheduler::getInterconnectGraph | ( | ) | const [inline, protected] |
References NoCScheduleProblem::getInterconnectGraph(), and getSchedulingProblem().
Referenced by assignSchedulingEntities(), KnowledgeNoCScheduler::costLinkForMessage(), findFreePackets(), findFreeSlotsForStream(), findFreeSlotsOnFirstLinkRoute(), findFreeSlotsOnLink(), findFreeSlotsOnRoute(), findScheduleEntityForMessage(), ClassicNoCScheduler::findScheduleEntityForMessageUsingClassic(), KnowledgeNoCScheduler::findScheduleEntityForMessageUsingKnowledge(), RandomNoCScheduler::findScheduleEntityForMessageUsingRandom(), findSlotsAllocatedForStream(), findSlotsOnRoute(), ClassicNoCScheduler::findSlotsOnRouteUsingClassic(), getLengthShortestPathBetweenNodes(), KnowledgeNoCScheduler::knowledge(), lowerPreferenceLevelSlotsOnRoute(), minFreeSlotsOnLink(), minimalDuration(), nrSlotsRequired(), print(), raisePreferenceLevelSlotsOnRoute(), reserveResources(), ripupScheduleEntity(), KnowledgeNoCScheduler::setRequirementsMessage(), KnowledgeNoCScheduler::setRequirementsMessages(), severityConflict(), sortRoutesOnCost(), and ClassicNoCScheduler::sortRoutesOnCostUsingClassic().

CSize NoCScheduler::getLengthShortestPathBetweenNodes | ( | const Node * | src, | |
const Node * | dst | |||
) | [protected] |
getLengthShortestPathBetweenNodes () Compute the shortes path distance between two nodes.
References Link::getDstNode(), Node::getId(), getInterconnectGraph(), InterconnectGraph::getNodes(), Node::outgoingLinksBegin(), and Node::outgoingLinksEnd().
Referenced by findAllRoutes(), findScheduleEntityForMessage(), ClassicNoCScheduler::findScheduleEntityForMessageUsingClassic(), KnowledgeNoCScheduler::findScheduleEntityForMessageUsingKnowledge(), and RandomNoCScheduler::findScheduleEntityForMessageUsingRandom().
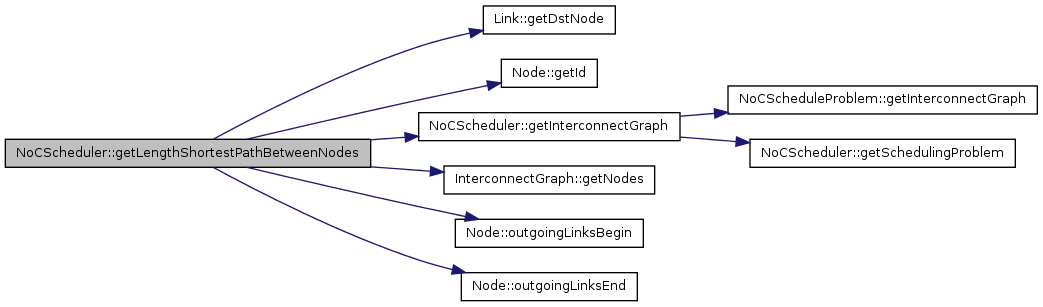
NoCScheduleProblem* NoCScheduler::getSchedulingProblem | ( | ) | const [inline, protected] |
References ASSERT, and curScheduleProblem.
Referenced by getInterconnectGraph(), markPreferedSlotsOnLinks(), messagesBegin(), messagesEnd(), nrSchedulingEntities(), RandomNoCScheduler::putMessagesInRandomOrder(), ripupScheduleEntity(), ripupStream(), schedulingEntitiesBegin(), schedulingEntitiesEnd(), RipupNoCScheduler::solve(), RandomNoCScheduler::solve(), KnowledgeNoCScheduler::solve(), GreedyNoCScheduler::solve(), ClassicNoCScheduler::solve(), and sortMessagesOnCost().
void NoCScheduler::lowerPreferenceLevelSlotsOnRoute | ( | NoCSchedulingEntity * | e | ) | [protected] |
lowerPreferenceLevelSlotsOnRoute () The function decreases the preference level of the slots used by the scheduling entity on its route. This preference level indicates how often a slot in a link is used in the scheduling problem. When its preference level is equal to infinity (UINT_MAX), the slot is also used in another scheduling problem that is scheduled along with this problem.
References getInterconnectGraph(), NoCSchedulingEntity::getRoute(), NoCSchedulingEntity::getSlotReservations(), InterconnectGraph::getSlotTableSize(), Route::linksBegin(), Route::linksEnd(), and Link::preferredSlots.
Referenced by releaseResources().
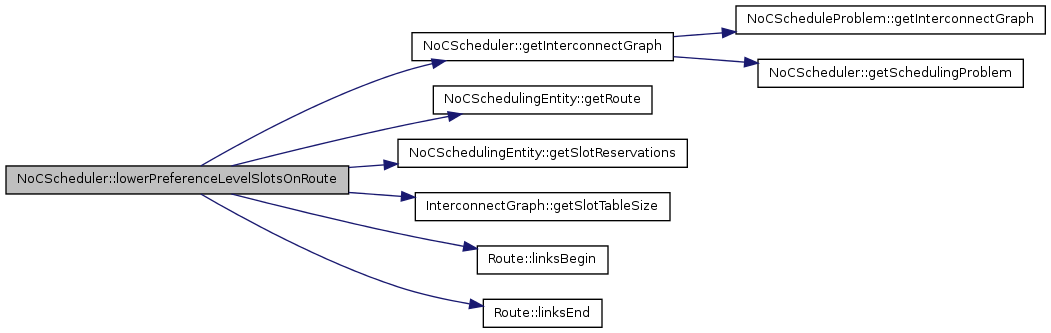
void NoCScheduler::markPreferedSlotsOnLinks | ( | SetOfNoCScheduleProblems & | problems | ) | [protected] |
markPreferedSlotsOnLinks () The function marks every slot in every link of the interconnect graph in problem as prefered (with maximimal level - UINT_MAX) when this slot is used in any of the scheduling problems solved so far. This scheduler uses this information to minimize the number of newly allocated slots. Leaving as many slots free as possible for other applications.
References NoCScheduleProblem::getInterconnectGraph(), getSchedulingProblem(), NoCScheduleProblem::isProblemSolved(), NoCScheduleProblem::markPreferedSlots(), SetOfNoCScheduleProblems::scheduleProblemsBegin(), and SetOfNoCScheduleProblems::scheduleProblemsEnd().
Referenced by schedule().
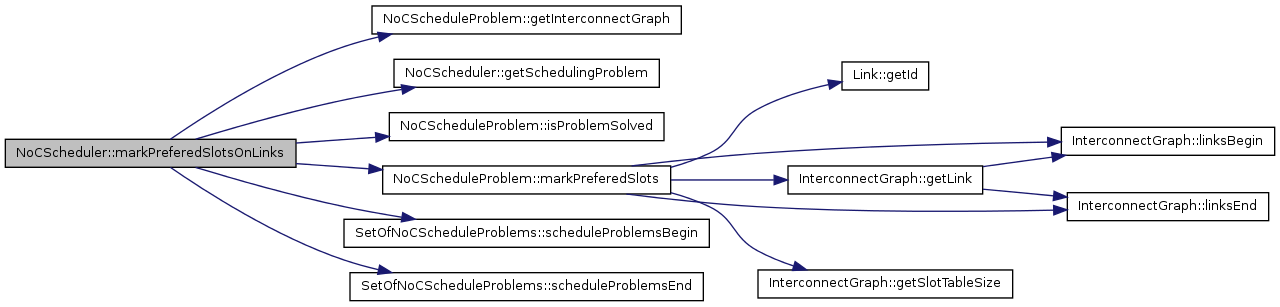
TTime NoCScheduler::maximalDuration | ( | const Message * | m, | |
TTime | startTime, | |||
CSize | lengthRoute | |||
) | const [protected] |
maximalDuration () The function returns the maximal duration for the message m. It considers the start time of messages which arrive later in the stream (i.e. higher sequence number) and the start time of this message.
References Message::getDuration(), Message::getNextMessageInStream(), NoCSchedulingEntity::getRoute(), Message::getSchedulingEntity(), NoCSchedulingEntity::getStartTime(), Message::getStartTime(), and Route::length().
Referenced by findScheduleEntityForMessage(), ClassicNoCScheduler::findScheduleEntityForMessageUsingClassic(), KnowledgeNoCScheduler::findScheduleEntityForMessageUsingKnowledge(), and RandomNoCScheduler::findScheduleEntityForMessageUsingRandom().
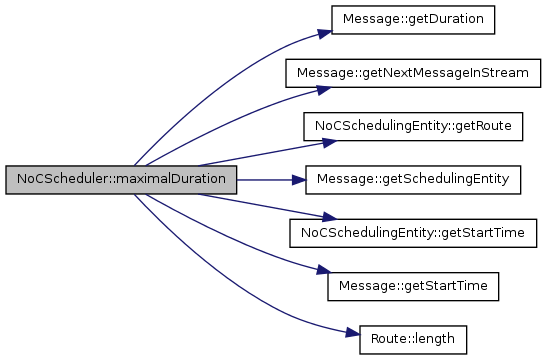
MessagesIter NoCScheduler::messagesBegin | ( | ) | [inline, protected] |
References getSchedulingProblem(), and NoCScheduleProblem::messagesBegin().
Referenced by assignSchedulingEntities(), ClassicNoCScheduler::classic(), GreedyNoCScheduler::greedy(), KnowledgeNoCScheduler::knowledge(), print(), RandomNoCScheduler::putMessagesInRandomOrder(), RandomNoCScheduler::random(), RipupNoCScheduler::ripup(), ripupScheduleEntity(), ripupStream(), KnowledgeNoCScheduler::setRequirementsMessages(), and sortMessagesOnCost().

MessagesCIter NoCScheduler::messagesBegin | ( | ) | const [inline, protected] |
References getSchedulingProblem(), and NoCScheduleProblem::messagesBegin().

MessagesIter NoCScheduler::messagesEnd | ( | ) | [inline, protected] |
References getSchedulingProblem(), and NoCScheduleProblem::messagesEnd().
Referenced by assignSchedulingEntities(), ClassicNoCScheduler::classic(), GreedyNoCScheduler::greedy(), KnowledgeNoCScheduler::knowledge(), print(), RandomNoCScheduler::putMessagesInRandomOrder(), RandomNoCScheduler::random(), RipupNoCScheduler::ripup(), ripupStream(), KnowledgeNoCScheduler::setRequirementsMessages(), and sortMessagesOnCost().

MessagesCIter NoCScheduler::messagesEnd | ( | ) | const [inline, protected] |
References getSchedulingProblem(), and NoCScheduleProblem::messagesEnd().

uint NoCScheduler::minFreeSlotsOnLink | ( | Link * | l, | |
const TTime | startTime, | |||
const TTime | duration | |||
) | [protected] |
minFreeSlotsOnLink () The function returns the minimum number of slots available on the link between the given timing constraints.
References SlotTable::getEndTime(), getInterconnectGraph(), SlotTable::getNrFreeSlots(), InterconnectGraph::getSlotTablePeriod(), InterconnectGraph::getSlotTableSize(), SlotTable::getStartTime(), Link::slotTableSeqBegin(), and Link::slotTableSeqEnd().
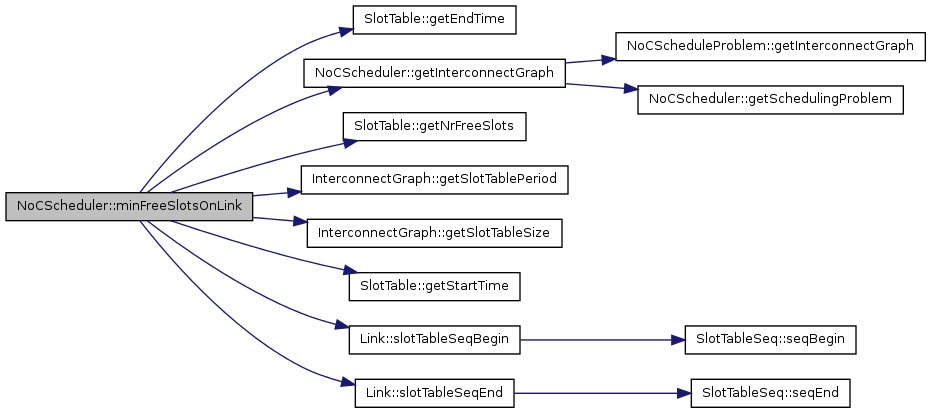
TTime NoCScheduler::minimalDuration | ( | const Message * | m, | |
TTime | startTime, | |||
SlotReservations | slotReservations | |||
) | const [protected] |
minimalDuration () The function returns the minimal duration for the message m. It considers the start time of messages the message given by 'startTime', the length of the route given by 'lengthRoute', and the slot reservations given by 'slotReservations'. The later is used to take the packatization overhead into account.
References InterconnectGraph::getFlitSize(), getInterconnectGraph(), InterconnectGraph::getPacketHeaderSize(), Message::getSize(), and InterconnectGraph::getSlotTableSize().
Referenced by findScheduleEntityForMessage(), ClassicNoCScheduler::findScheduleEntityForMessageUsingClassic(), KnowledgeNoCScheduler::findScheduleEntityForMessageUsingKnowledge(), and RandomNoCScheduler::findScheduleEntityForMessageUsingRandom().
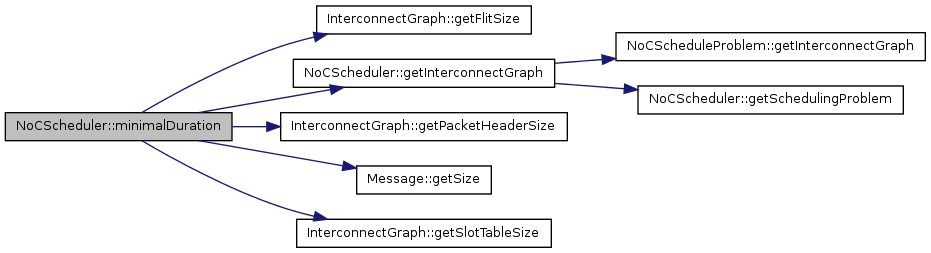
uint NoCScheduler::nrSchedulingEntities | ( | ) | const [inline, protected] |
References getSchedulingProblem(), and NoCScheduleProblem::nrSchedulingEntities().

uint NoCScheduler::nrSlotsRequired | ( | const TTime | duration, | |
const CSize | size, | |||
const uint | nrPacketsPerSlotTable | |||
) | [protected] |
nrSlotsRequired () The function computes the number of slots required to sent a communication event within the given time bounds along a route. It takes the packatization overhead into account.
References InterconnectGraph::getFlitSize(), getInterconnectGraph(), InterconnectGraph::getPacketHeaderSize(), and InterconnectGraph::getSlotTableSize().
Referenced by findSlotsOnRoute(), ClassicNoCScheduler::findSlotsOnRouteUsingClassic(), and KnowledgeNoCScheduler::setRequirementsMessage().
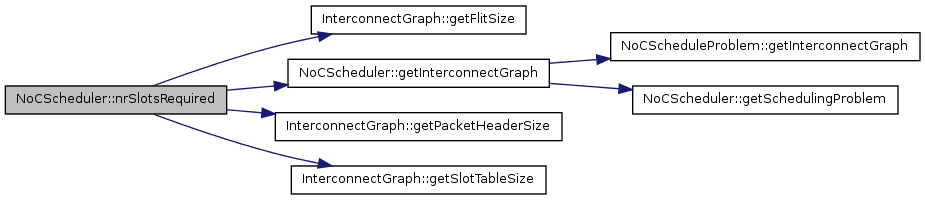
ostream & NoCScheduler::print | ( | ostream & | out | ) | const [protected] |
print () Output the status of the scheduler (overview of all link allocations) and the number of messages scheduled to the supplied output stream.
References getInterconnectGraph(), Message::getSchedulingEntity(), InterconnectGraph::linksEnd(), messagesBegin(), messagesEnd(), and Link::print().
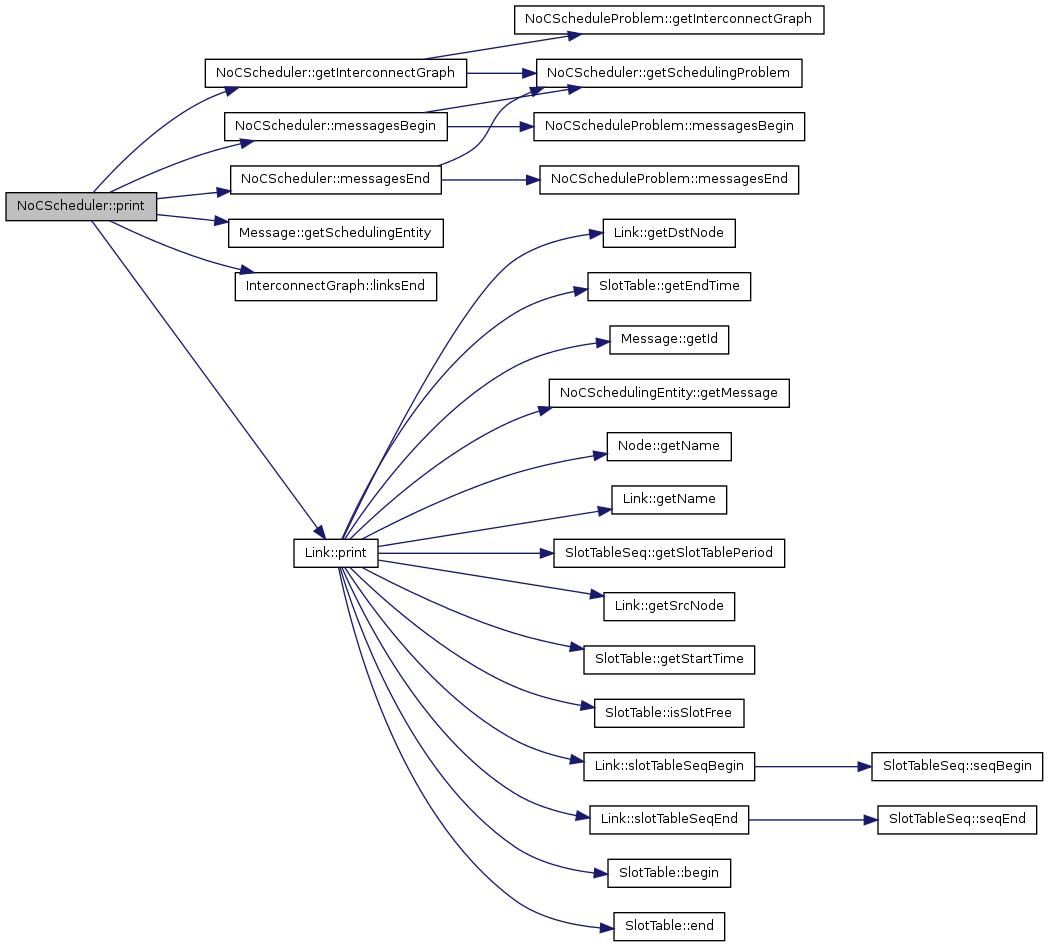
void NoCScheduler::raisePreferenceLevelSlotsOnRoute | ( | NoCSchedulingEntity * | e | ) | [protected] |
raisePreferenceLevelSlotsOnRoute () The function increases the preference level of the slots used by the scheduling entity on its route. This preference level indicates how often a slot in a link is used in the scheduling problem. When its preference level is equal to infinity (UINT_MAX), the slot is also used in another scheduling problem that is scheduled along with this problem.
References getInterconnectGraph(), NoCSchedulingEntity::getRoute(), NoCSchedulingEntity::getSlotReservations(), InterconnectGraph::getSlotTableSize(), Route::linksBegin(), Route::linksEnd(), and Link::preferredSlots.
Referenced by reserveResources().
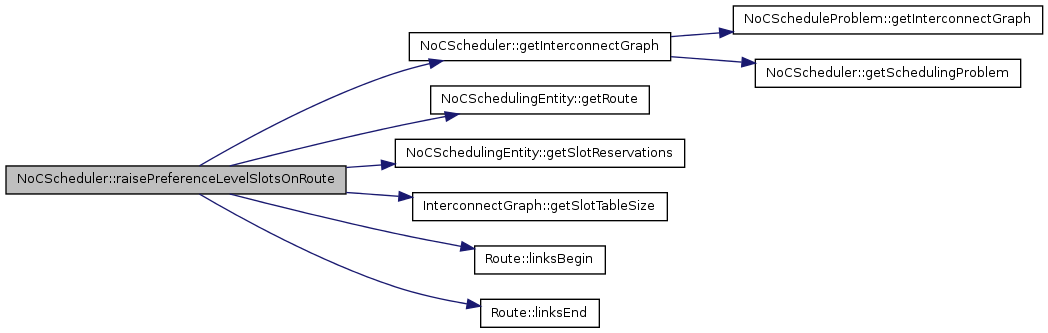
void NoCScheduler::releaseResources | ( | NoCSchedulingEntity * | e | ) | [protected] |
releaseResources () Release resources in the architecture graph claimed by the scheduling entity e.
References NoCSchedulingEntity::getRoute(), Route::linksBegin(), Route::linksEnd(), lowerPreferenceLevelSlotsOnRoute(), Link::releaseSlots(), and NoCSchedulingEntity::resourcesReserved().
Referenced by RandomNoCScheduler::random(), ripupScheduleEntity(), and ripupStream().
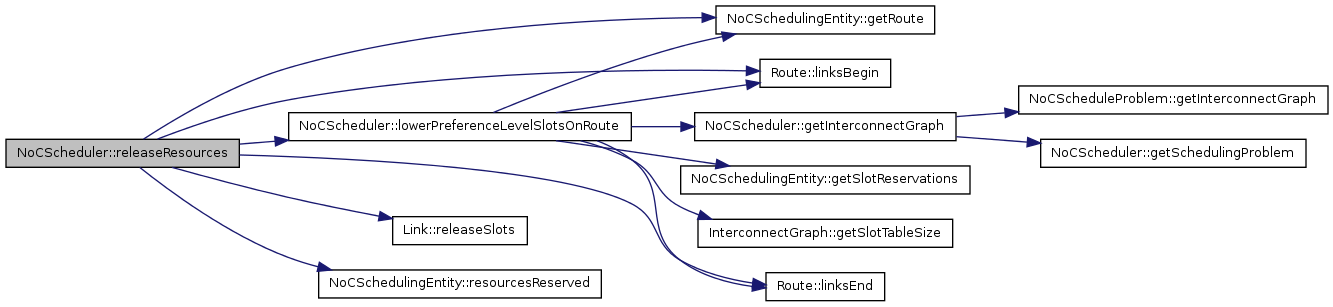
void NoCScheduler::reserveResources | ( | NoCSchedulingEntity * | e | ) | [protected] |
reserveResources () Claim resources in the architecture graph for the scheduling entity e.
References getInterconnectGraph(), NoCSchedulingEntity::getRoute(), NoCSchedulingEntity::getSlotReservations(), InterconnectGraph::getSlotTableSize(), Route::linksBegin(), Route::linksEnd(), raisePreferenceLevelSlotsOnRoute(), Link::reserveSlots(), and NoCSchedulingEntity::resourcesReserved().
Referenced by assignSchedulingEntities(), findScheduleEntityForMessage(), ClassicNoCScheduler::findScheduleEntityForMessageUsingClassic(), KnowledgeNoCScheduler::findScheduleEntityForMessageUsingKnowledge(), and RandomNoCScheduler::findScheduleEntityForMessageUsingRandom().
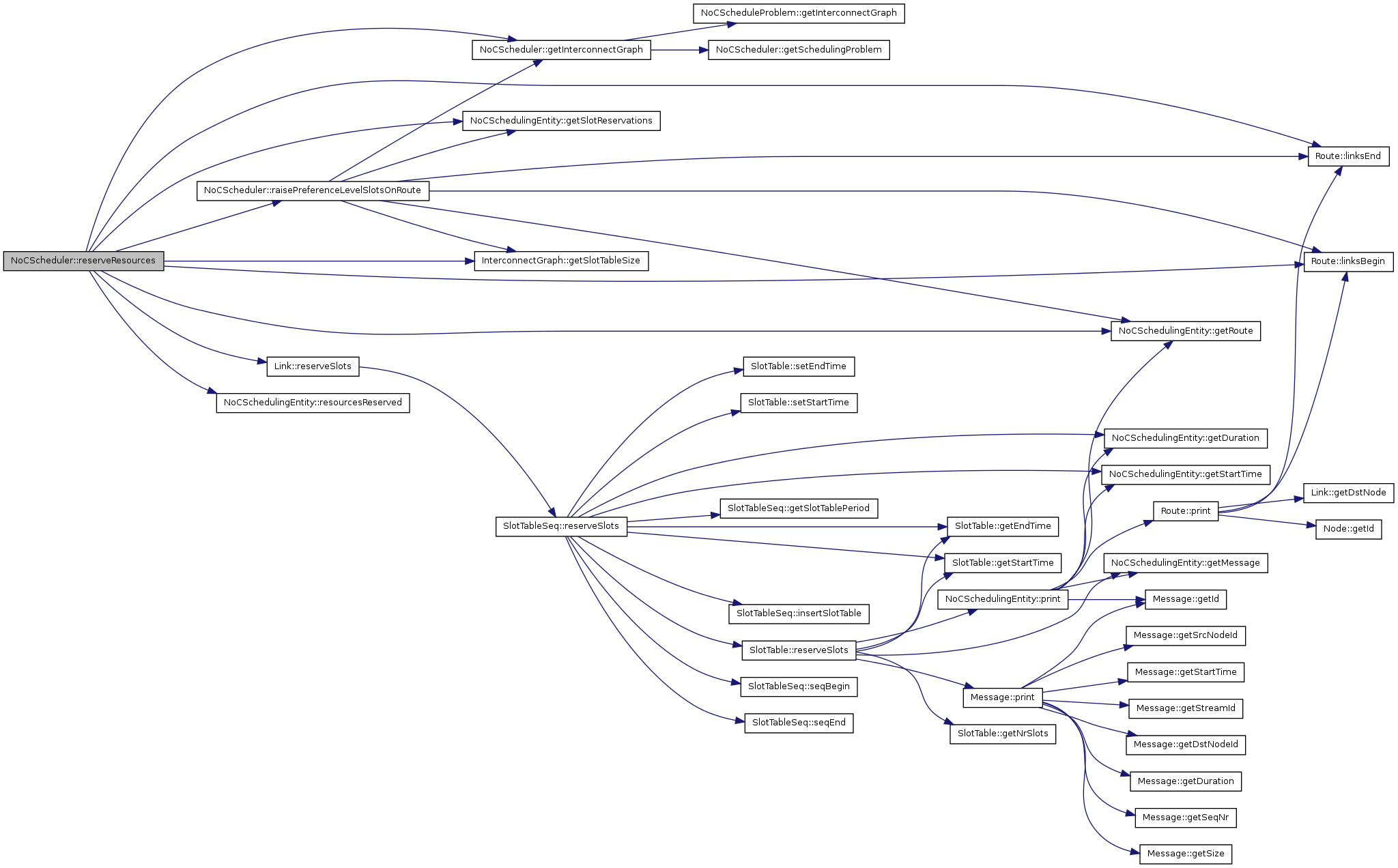
void NoCScheduler::ripupScheduleEntity | ( | MessagesIter | iterMsg | ) | [protected] |
ripupScheduleEntity () Remove the schedule entity which has the largest conflict with the given message.
References findAllRoutes(), Message::getDstNodeId(), Message::getDuration(), getInterconnectGraph(), NoCScheduleProblem::getMessages(), InterconnectGraph::getNode(), getSchedulingProblem(), Message::getSrcNodeId(), Message::getStartTime(), messagesBegin(), releaseResources(), severityConflict(), and sortRoutesOnCost().
Referenced by KnowledgeNoCScheduler::knowledge(), RandomNoCScheduler::random(), and RipupNoCScheduler::ripup().
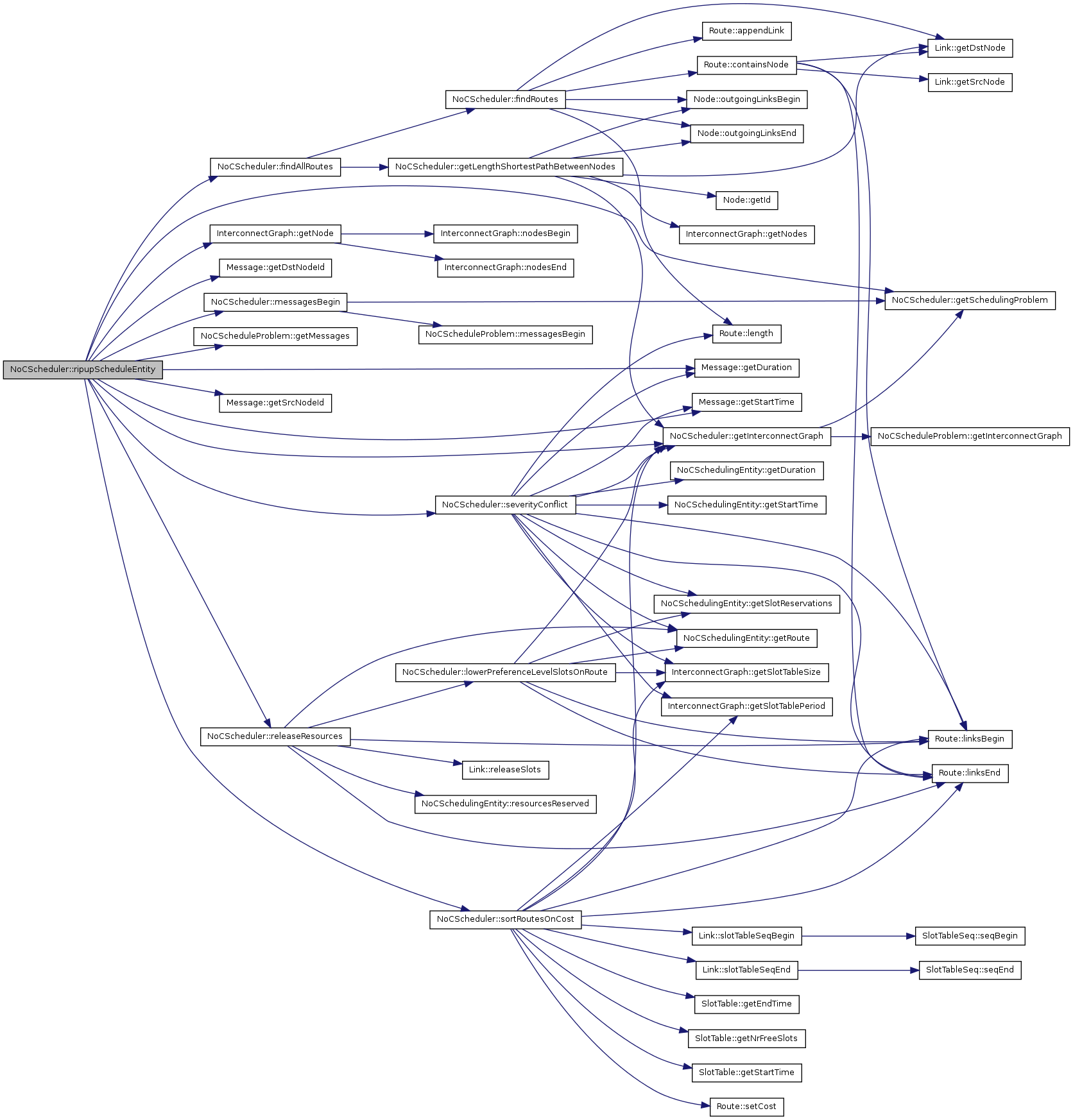
void NoCScheduler::ripupStream | ( | MessagesIter | iterMsg | ) | [protected] |
ripupStream () Remove schedule of all messages which belong to the stream.
References NoCScheduleProblem::getMessages(), Message::getSchedulingEntity(), getSchedulingProblem(), Message::getStreamId(), messagesBegin(), messagesEnd(), releaseResources(), and Message::setSchedulingEntity().
Referenced by ClassicNoCScheduler::classic().
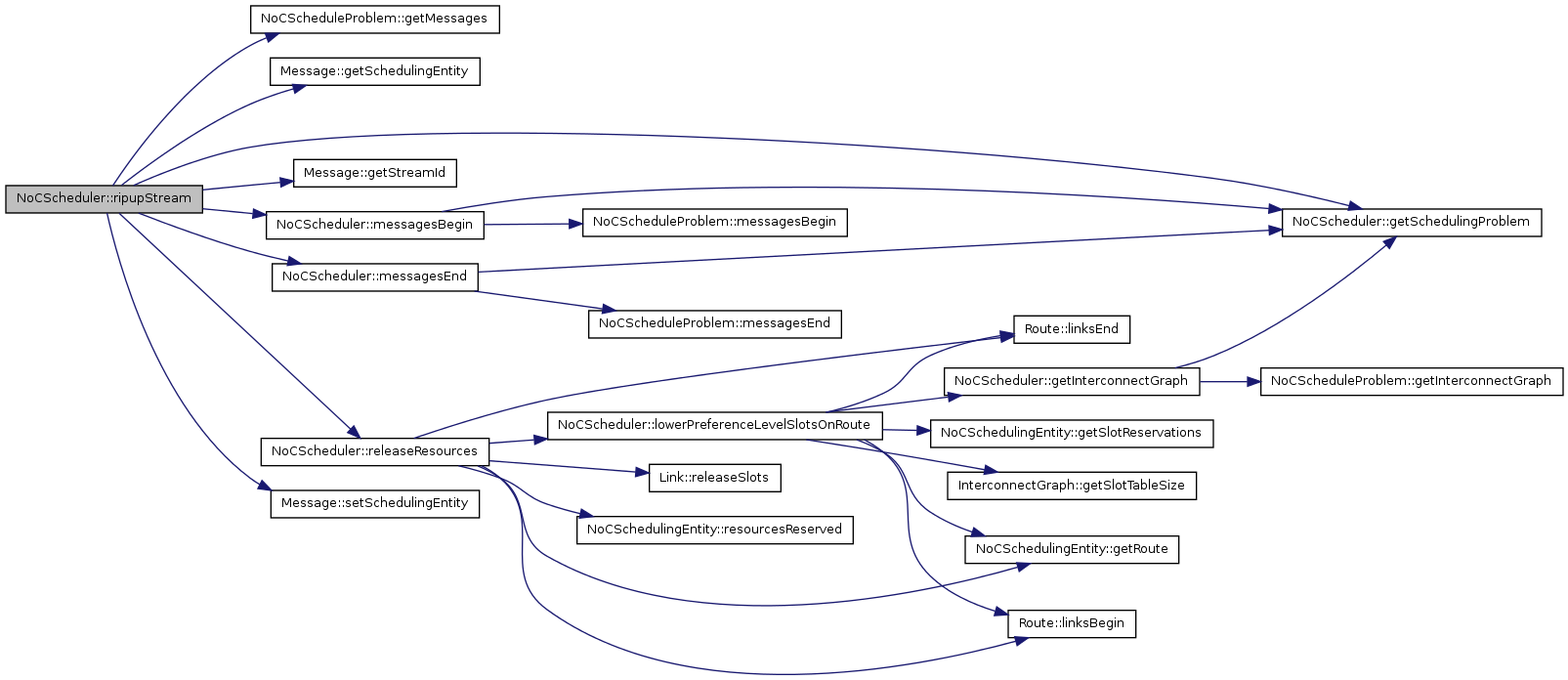
bool NoCScheduler::schedule | ( | SetOfNoCScheduleProblems & | problems | ) |
schedule () The function tries to find a valid schedule for all scheduling problems. On success, the function returns true. Otherwise, it returns false.
References NoCScheduleProblem::getName(), NoCScheduleProblem::isProblemSolved(), logError(), markPreferedSlotsOnLinks(), SetOfNoCScheduleProblems::scheduleProblemsBegin(), SetOfNoCScheduleProblems::scheduleProblemsEnd(), setSchedulingProblem(), NoCScheduleProblem::setSolvedFlag(), and solve().
Referenced by NoCMapping::scheduleCommunication(), and solveSchedulingProblems().
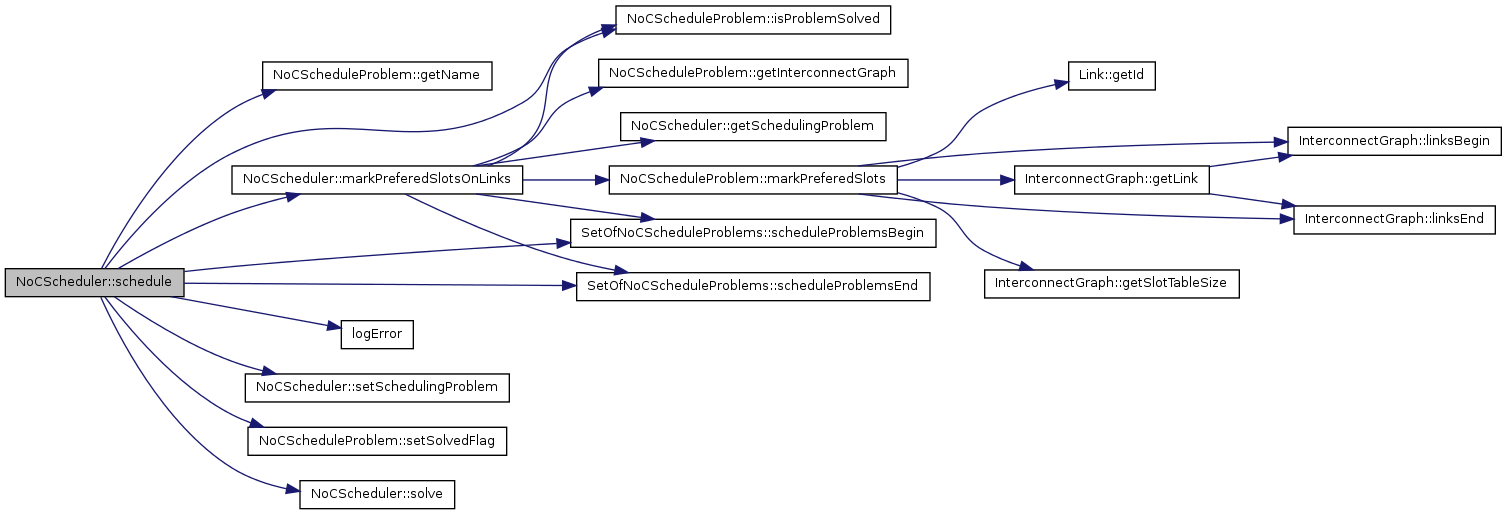
NoCSchedulingEntitiesIter NoCScheduler::schedulingEntitiesBegin | ( | ) | [inline, protected] |
References getSchedulingProblem(), and NoCScheduleProblem::schedulingEntitiesBegin().

NoCSchedulingEntitiesIter NoCScheduler::schedulingEntitiesEnd | ( | ) | [inline, protected] |
References getSchedulingProblem(), and NoCScheduleProblem::schedulingEntitiesEnd().

void NoCScheduler::setSchedulingProblem | ( | NoCScheduleProblem * | p | ) | [inline, protected] |
References curScheduleProblem.
Referenced by assignSchedulingEntities(), and schedule().
double NoCScheduler::severityConflict | ( | NoCSchedulingEntity * | e, | |
Message * | m, | |||
Route * | r | |||
) | [protected] |
severityConflictSchedulingEntities () The function computes the severity of a conflict between a scheduling entity 'e' and a message 'm' which must be scheduled over a route 'r'. The severity is defined as the number of slots used by 'e' on the route 'r'. Only slots within the time bounds of 'm' are counted.
References Message::getDuration(), NoCSchedulingEntity::getDuration(), getInterconnectGraph(), NoCSchedulingEntity::getRoute(), NoCSchedulingEntity::getSlotReservations(), InterconnectGraph::getSlotTablePeriod(), InterconnectGraph::getSlotTableSize(), Message::getStartTime(), NoCSchedulingEntity::getStartTime(), Route::length(), Route::linksBegin(), and Route::linksEnd().
Referenced by ripupScheduleEntity().
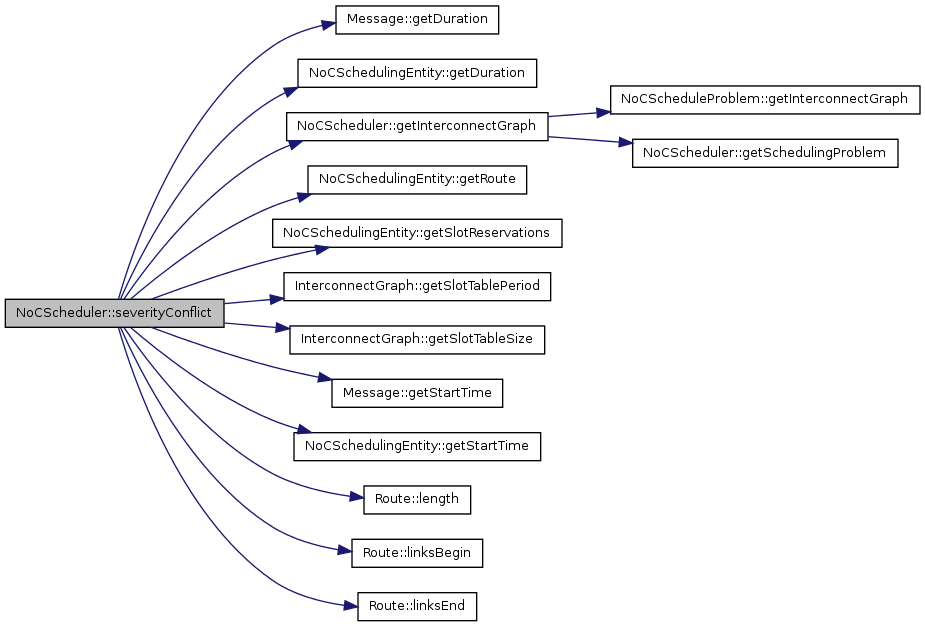
virtual bool NoCScheduler::solve | ( | ) | [protected, pure virtual] |
Implemented in ClassicNoCScheduler, GreedyNoCScheduler, KnowledgeNoCScheduler, RandomNoCScheduler, and RipupNoCScheduler.
Referenced by schedule().
void NoCScheduler::sortMessagesOnCost | ( | ) | [protected] |
sortMessagesOnCost () The function sorts all messages using a cost function with cost from high to low. The cost of a communication event is determined by its size and time bounds.
References Message::getDuration(), NoCScheduleProblem::getMessages(), getSchedulingProblem(), Message::getSize(), messagesBegin(), messagesEnd(), and Message::setCost().
Referenced by ClassicNoCScheduler::classic(), GreedyNoCScheduler::greedy(), KnowledgeNoCScheduler::knowledge(), and RipupNoCScheduler::ripup().
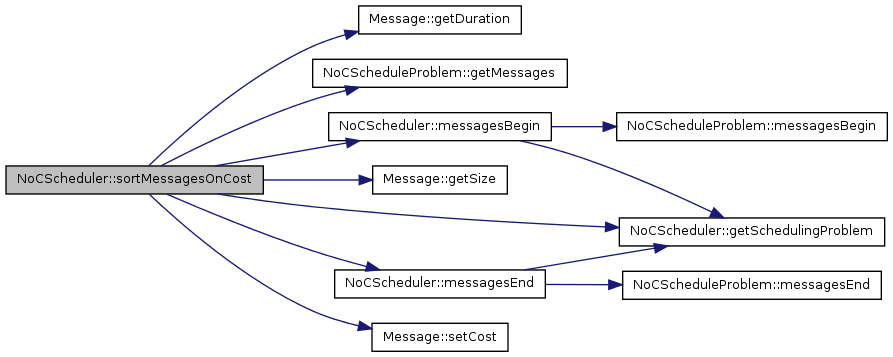
void NoCScheduler::sortRoutesOnCost | ( | Routes & | routes, | |
const TTime | startTime, | |||
const TTime | duration | |||
) | [protected] |
sortRoutesOnCost () The function sorts all routes using a cost function with cost from high to low. The cost is determined by the minimum number of free slots available overall links within the given time bounds.
References SlotTable::getEndTime(), getInterconnectGraph(), SlotTable::getNrFreeSlots(), InterconnectGraph::getSlotTablePeriod(), InterconnectGraph::getSlotTableSize(), SlotTable::getStartTime(), Route::linksBegin(), Route::linksEnd(), Route::setCost(), Link::slotTableSeqBegin(), and Link::slotTableSeqEnd().
Referenced by findScheduleEntityForMessage(), and ripupScheduleEntity().
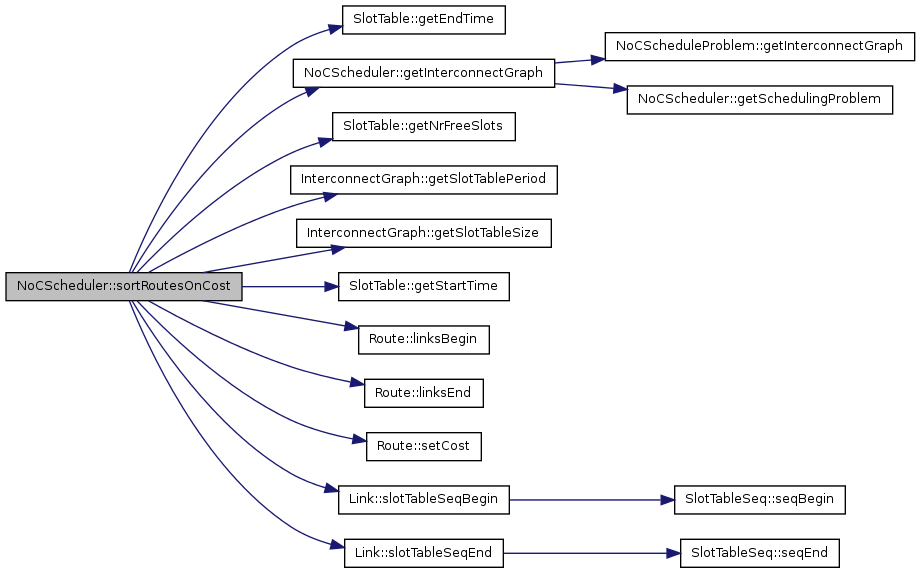
Member Data Documentation
Referenced by getSchedulingProblem(), and setSchedulingProblem().
The documentation for this class was generated from the following files: