FSMSADF::SDF3Flow Class Reference
#include <flow.h>
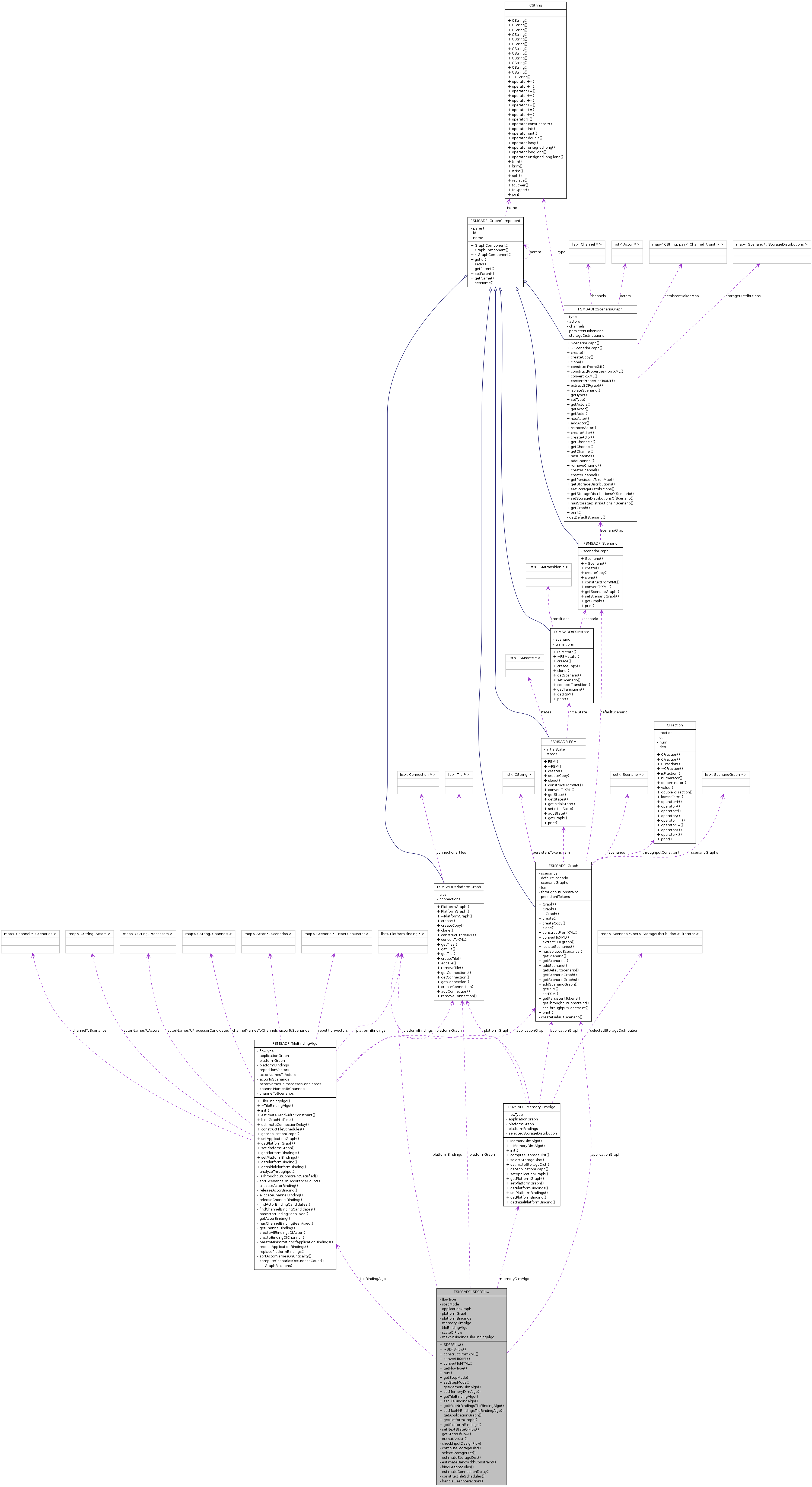
Detailed Description
Graph mapping to MP-SoC flow
Member Enumeration Documentation
Constructor & Destructor Documentation
SDF3Flow::~SDF3Flow | ( | ) |
~SDF3Flow () Destructor.
References applicationGraph, platformBindings, and platformGraph.
Member Function Documentation
void SDF3Flow::bindGraphtoTiles | ( | ) | [private] |
bindGraphtoTiles () Bind graph to the tile resources.
References FSMSADF::TileBindingAlgo::bindGraphtoTiles(), FlowEstimateConnectionDelay, FlowFailed, getMaxNrBindingsTileBindingAlgo(), logInfo(), setNextStateOfFlow(), and tileBindingAlgo.
Referenced by run().
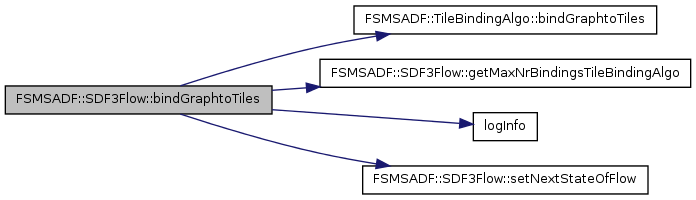
void SDF3Flow::checkInputDesignFlow | ( | ) | [private] |
checkInputDesignFlow () The function checks that all required inputs are present and it initializes the used data-structures.
References FlowComputeStorageDist, getApplicationGraph(), getPlatformGraph(), FSMSADF::TileBindingAlgo::init(), FSMSADF::MemoryDimAlgo::init(), FSMSADF::Graph::isolateScenarios(), logInfo(), memoryDimAlgo, platformBindings, FSMSADF::TileBindingAlgo::setApplicationGraph(), FSMSADF::MemoryDimAlgo::setApplicationGraph(), setNextStateOfFlow(), FSMSADF::TileBindingAlgo::setPlatformBindings(), FSMSADF::MemoryDimAlgo::setPlatformBindings(), FSMSADF::TileBindingAlgo::setPlatformGraph(), FSMSADF::MemoryDimAlgo::setPlatformGraph(), and tileBindingAlgo.
Referenced by run().
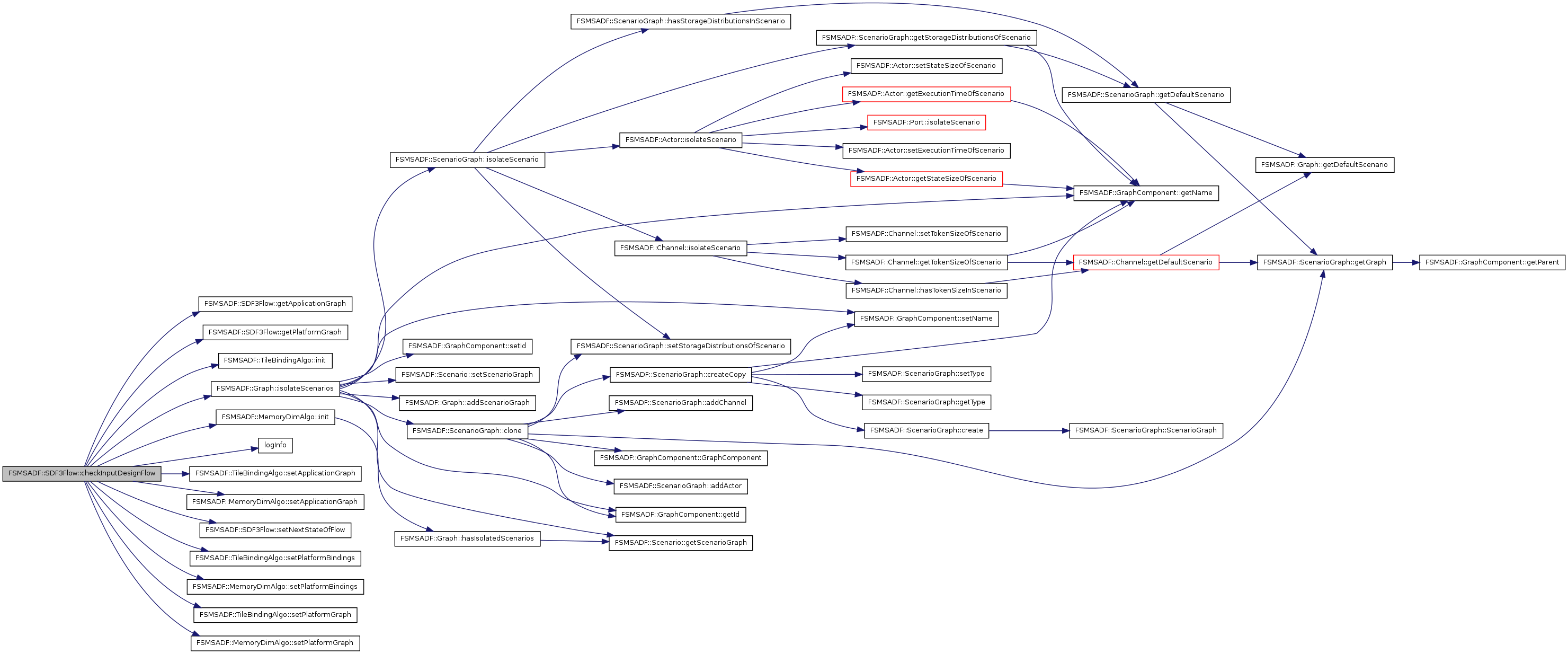
void SDF3Flow::computeStorageDist | ( | ) | [private] |
computeStorageDist () Compute storage distributions for the application graph.
References FSMSADF::MemoryDimAlgo::computeStorageDist(), FlowFailed, FlowSelectStorageDist, logInfo(), memoryDimAlgo, and setNextStateOfFlow().
Referenced by run().
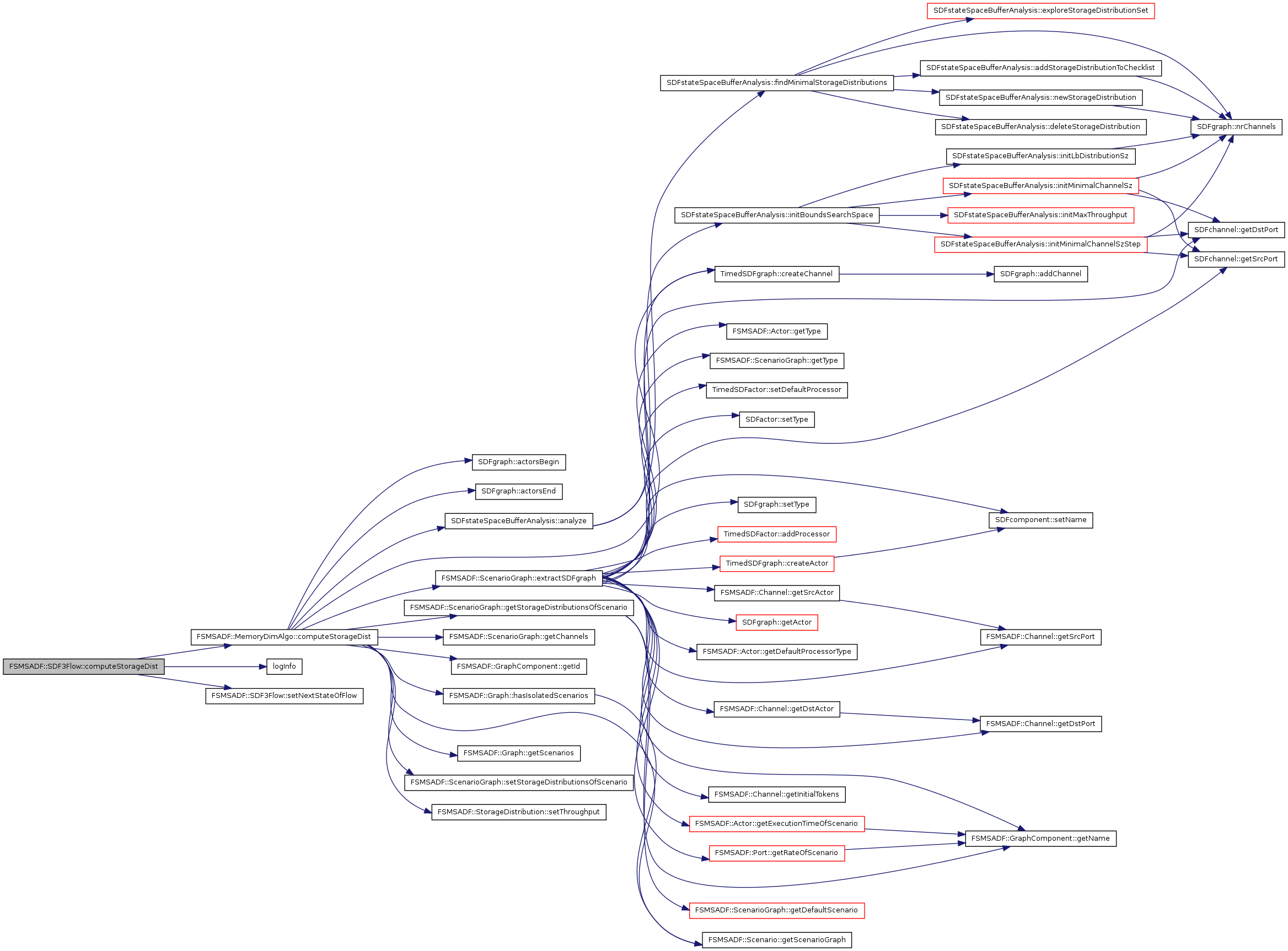
void SDF3Flow::constructFromXML | ( | const CNodePtr | sdf3Node | ) |
References applicationGraph, CGetChildNode(), CHasChildNode(), CNextNode(), FSMSADF::PlatformBinding::constructFromXML(), FSMSADF::PlatformGraph::constructFromXML(), FSMSADF::Graph::constructFromXML(), platformBindings, and platformGraph.
Referenced by FSMSADF::mapApplicationGraphToArchitectureGraph().
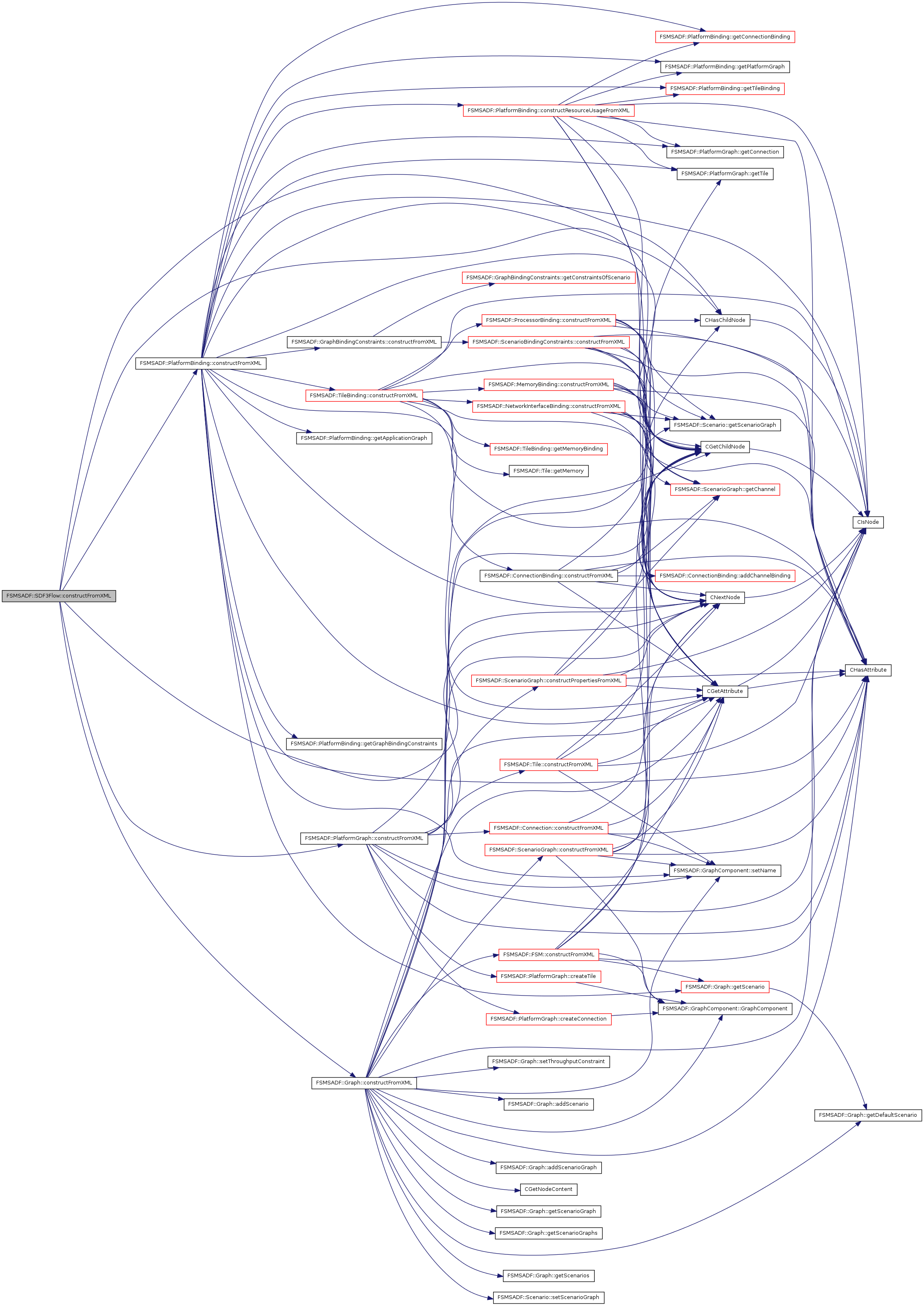
void SDF3Flow::constructTileSchedules | ( | ) | [private] |
constructTileSchedules () Construct a static-order and TDMA schedule for every tile to which actors are bound.
References FSMSADF::TileBindingAlgo::constructTileSchedules(), FlowCompleted, FlowSelectStorageDist, getMaxNrBindingsTileBindingAlgo(), logInfo(), setNextStateOfFlow(), and tileBindingAlgo.
Referenced by run().
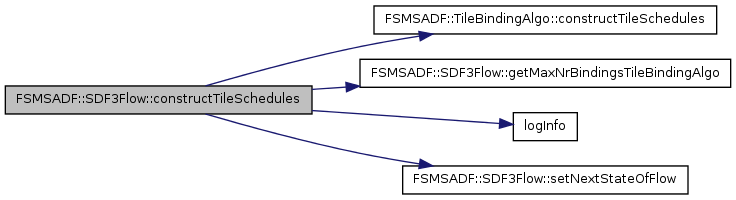
void SDF3Flow::convertToHTML | ( | const CString & | dirname | ) |
convertToHTML () The function outputs the application graph, platform graph, mapping, and resource usage to a set of HTML files. These files are stored in the directory with the supplied dirname.
References FSMSADF::OutputHTML::outputAsHTML(), and FSMSADF::OutputHTML::setDirname().
Referenced by FSMSADF::mapApplicationGraphToArchitectureGraph().
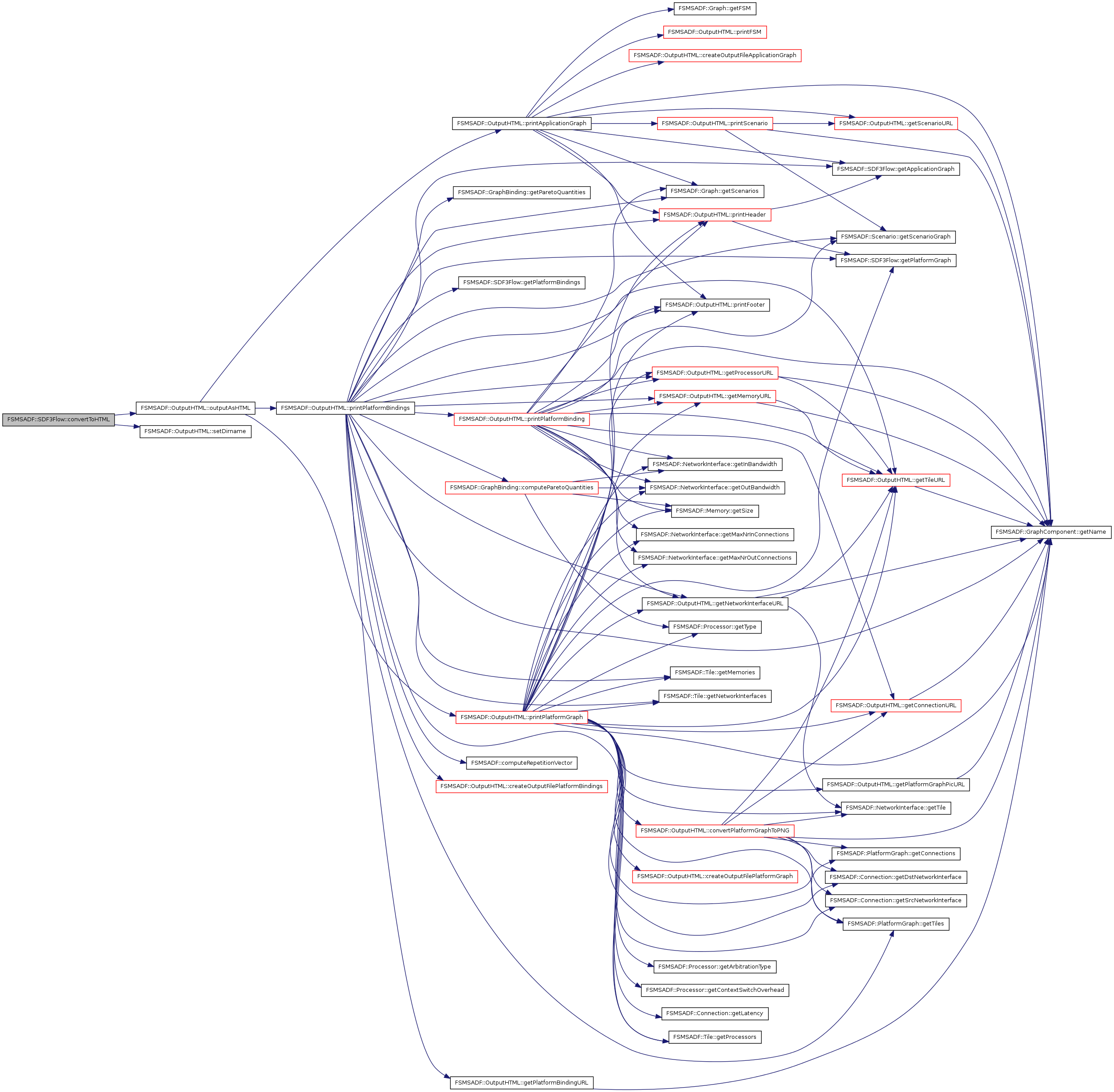
void SDF3Flow::convertToXML | ( | const CNodePtr | sdf3Node | ) |
convertToXML () The function add the application graph, platform graph, mapping, and resource usage to the sdf3Node..
References applicationGraph, CAddAttribute(), CAddNode(), FSMSADF::PlatformBinding::convertToXML(), FSMSADF::PlatformGraph::convertToXML(), FSMSADF::Graph::convertToXML(), platformBindings, and platformGraph.
Referenced by FSMSADF::mapApplicationGraphToArchitectureGraph(), and outputAsXML().
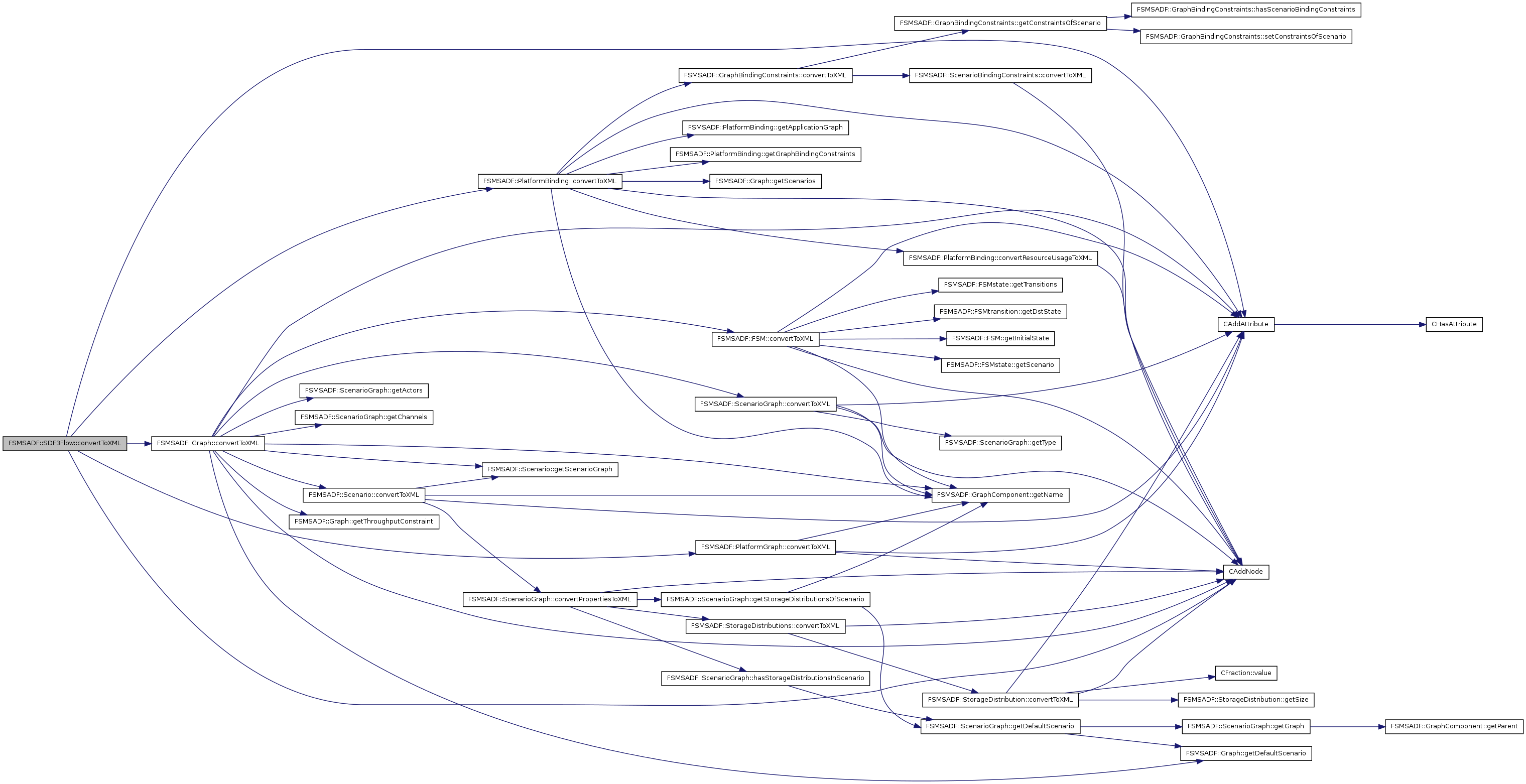
void SDF3Flow::estimateBandwidthConstraint | ( | ) | [private] |
estimateBandwidthConstraint () Estimate bandwidth requirement of the channels when mapped to a connection.
References FSMSADF::TileBindingAlgo::estimateBandwidthConstraint(), FlowBindGraphtoTile, FlowFailed, logInfo(), setNextStateOfFlow(), and tileBindingAlgo.
Referenced by run().

void SDF3Flow::estimateConnectionDelay | ( | ) | [private] |
estimateConnectionDelay () Estimaet delay constraints of channels when bound to a connection.
References FSMSADF::TileBindingAlgo::estimateConnectionDelay(), FlowConstructTileSchedules, FlowFailed, logInfo(), setNextStateOfFlow(), and tileBindingAlgo.
Referenced by run().

void SDF3Flow::estimateStorageDist | ( | ) | [private] |
estimateStorageDist () Estimate storage distibution
References FSMSADF::MemoryDimAlgo::estimateStorageDist(), FlowEstimateBandwidthConstraint, FlowFailed, logInfo(), memoryDimAlgo, and setNextStateOfFlow().
Referenced by run().
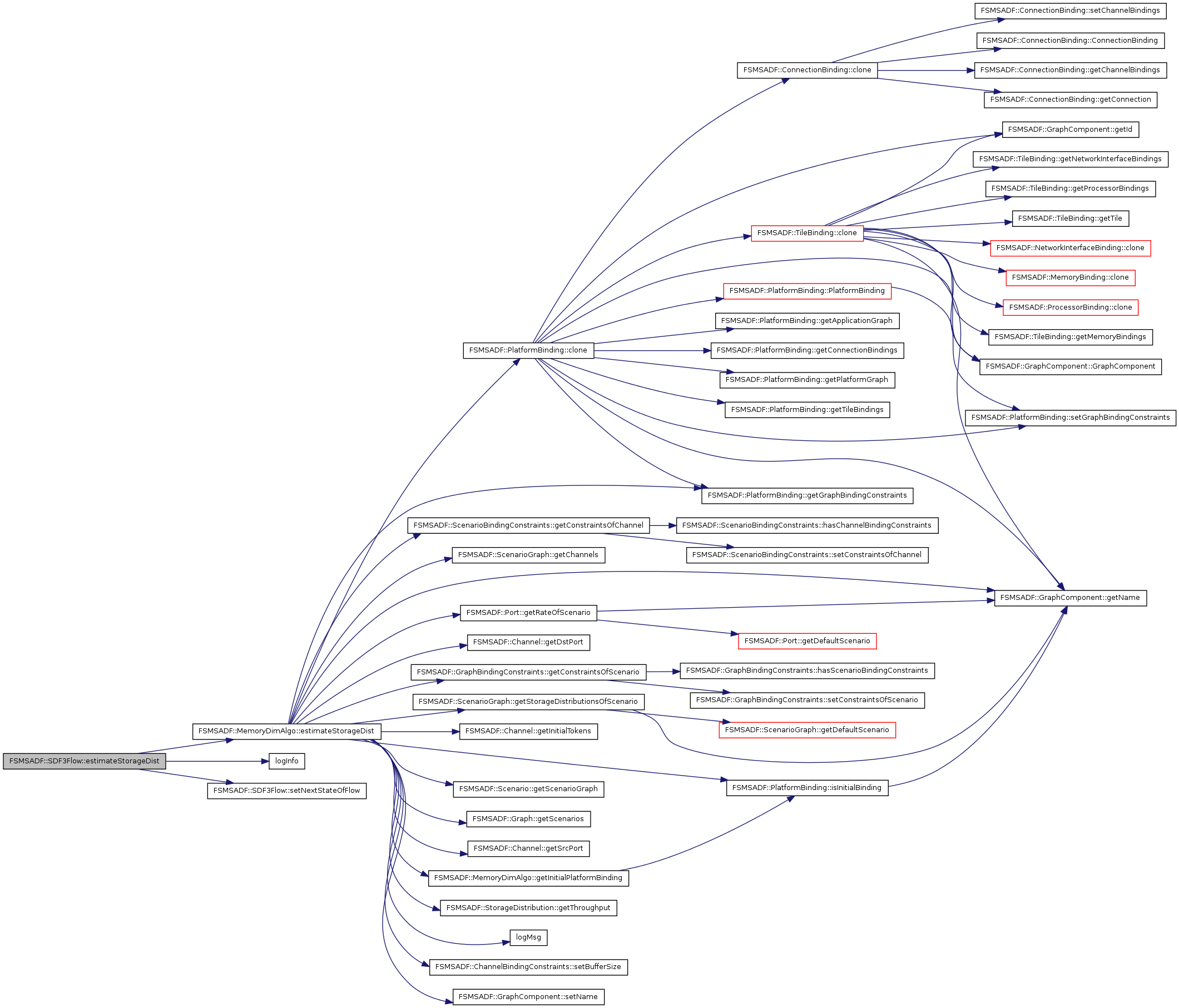
Graph* FSMSADF::SDF3Flow::getApplicationGraph | ( | ) | [inline] |
uint FSMSADF::SDF3Flow::getMaxNrBindingsTileBindingAlgo | ( | ) | const [inline] |
References maxNrBindingsTileBindingAlgo.
Referenced by bindGraphtoTiles(), and constructTileSchedules().
MemoryDimAlgo* FSMSADF::SDF3Flow::getMemoryDimAlgo | ( | ) | const [inline] |
References memoryDimAlgo.
PlatformBindings& FSMSADF::SDF3Flow::getPlatformBindings | ( | ) | [inline] |
References platformBindings.
Referenced by FSMSADF::OutputHTML::printPlatformBindings().
PlatformGraph* FSMSADF::SDF3Flow::getPlatformGraph | ( | ) | [inline] |
FlowState FSMSADF::SDF3Flow::getStateOfFlow | ( | ) | [inline, private] |
References stateOfFlow.
Referenced by run().
TileBindingAlgo* FSMSADF::SDF3Flow::getTileBindingAlgo | ( | ) | const [inline] |
References tileBindingAlgo.
void SDF3Flow::handleUserInteraction | ( | ) | [private] |
handleUserInteraction () The function request the user for the next action to perform. Possible actions include continuing with the next step of the flow, completing the remaining flow or printing the current result of the flow to the terminal.
References c, outputAsXML(), and setStepMode().
Referenced by run().
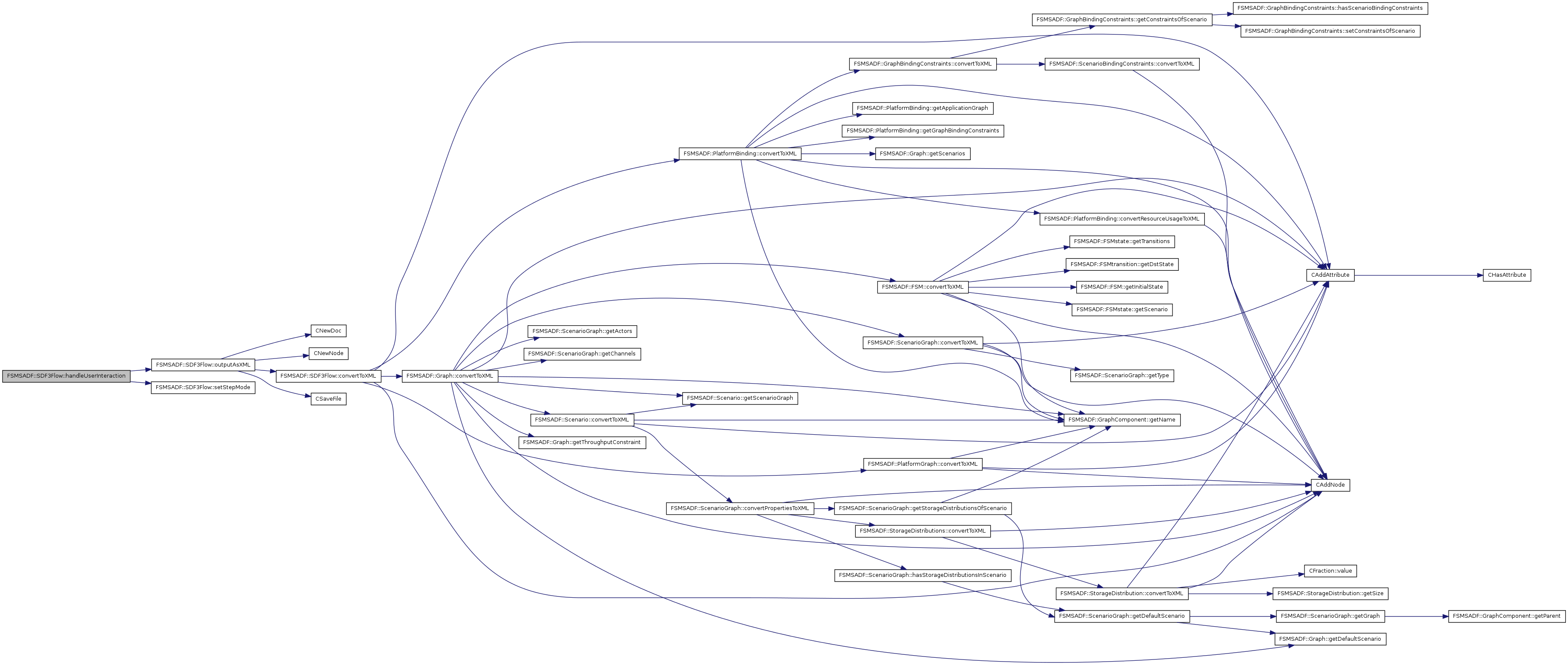
void SDF3Flow::outputAsXML | ( | ostream & | out | ) | [private] |
outputAsXML () The function ouputs an sdf3 node to the supplied stream.
References CNewDoc(), CNewNode(), convertToXML(), and CSaveFile().
Referenced by handleUserInteraction().
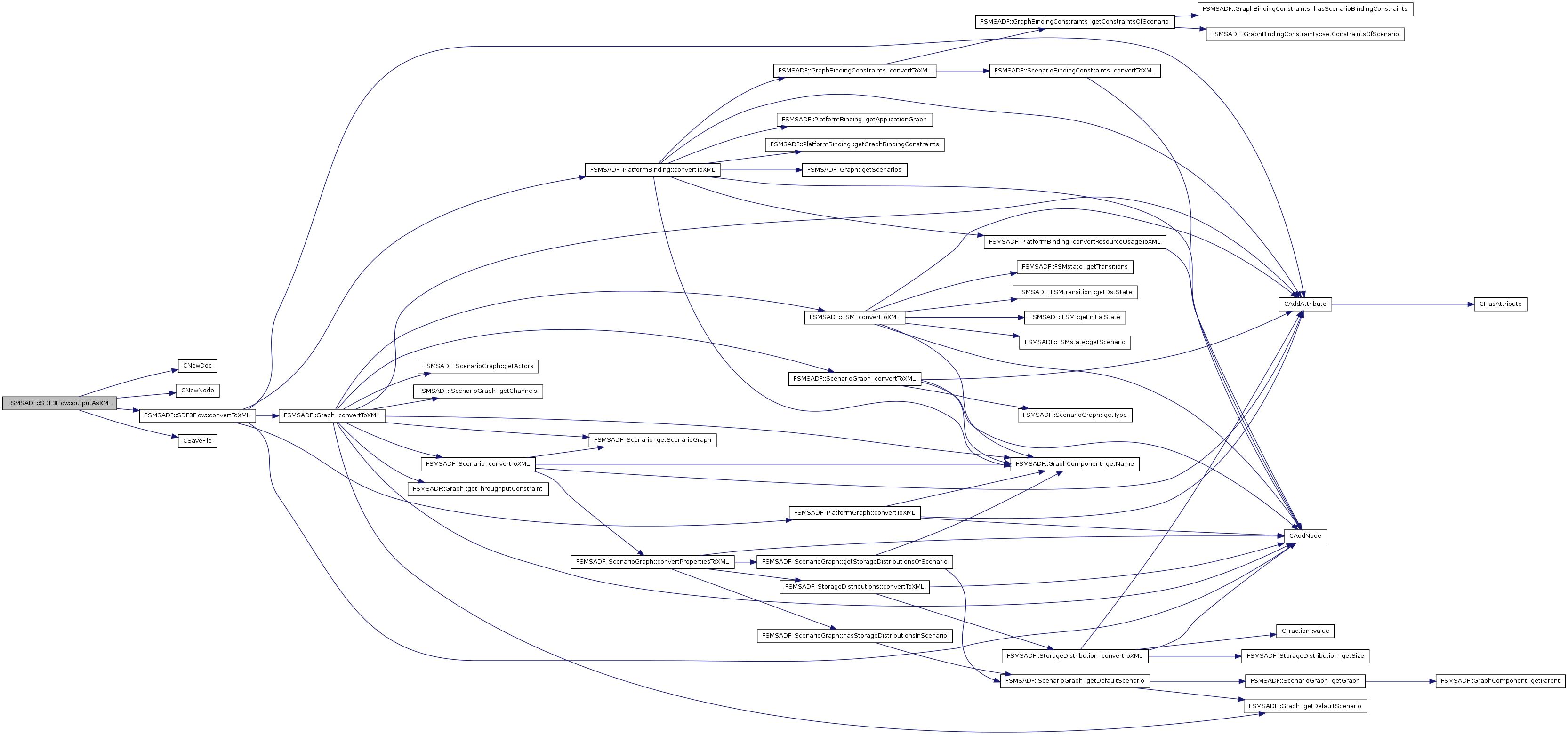
SDF3Flow::FlowState SDF3Flow::run | ( | ) |
run () Execute the design flow. The function returns the last state reached by the design flow. Its value is equal to 'FlowCompleted' when all phases have been executed succesfully or else it is 'FlowFailed' which indicates that a step of the flow could not be completed succesfully.
References bindGraphtoTiles(), checkInputDesignFlow(), computeStorageDist(), constructTileSchedules(), estimateBandwidthConstraint(), estimateConnectionDelay(), estimateStorageDist(), FlowBindGraphtoTile, FlowCompleted, FlowComputeStorageDist, FlowConstructTileSchedules, FlowEstimateBandwidthConstraint, FlowEstimateConnectionDelay, FlowEstimateStorageDist, FlowFailed, FlowSelectStorageDist, FlowStart, getStateOfFlow(), getStepMode(), handleUserInteraction(), printTimer(), selectStorageDist(), startTimer(), and stopTimer().
Referenced by FSMSADF::mapApplicationGraphToArchitectureGraph().
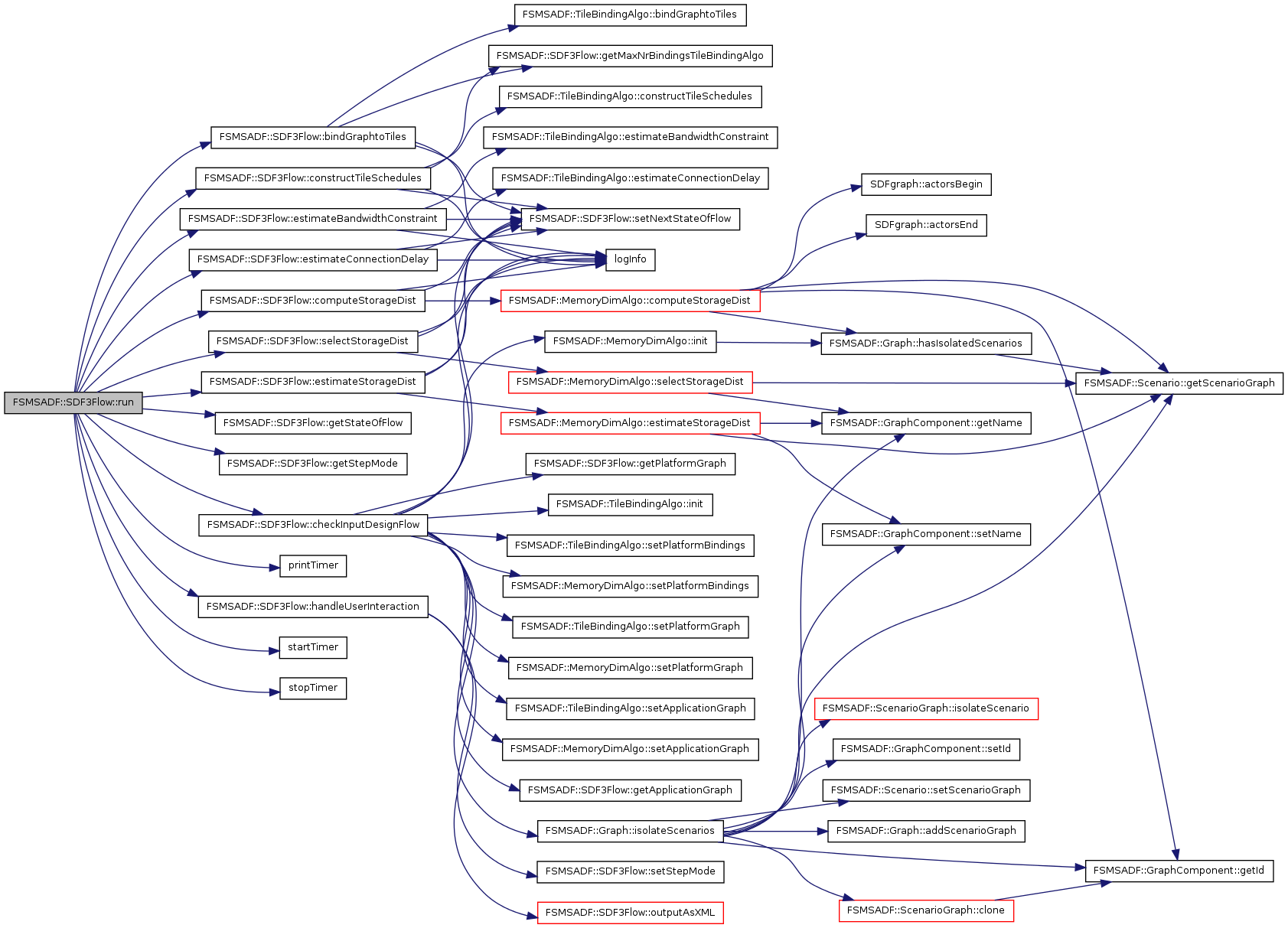
void SDF3Flow::selectStorageDist | ( | ) | [private] |
selectStorageDist () Select storage distribution.
References FlowEstimateStorageDist, FlowFailed, logInfo(), memoryDimAlgo, FSMSADF::MemoryDimAlgo::selectStorageDist(), and setNextStateOfFlow().
Referenced by run().

void FSMSADF::SDF3Flow::setMaxNrBindingsTileBindingAlgo | ( | const uint | n | ) | [inline] |
References maxNrBindingsTileBindingAlgo.
Referenced by FSMSADF::mapApplicationGraphToArchitectureGraph().
void FSMSADF::SDF3Flow::setMemoryDimAlgo | ( | MemoryDimAlgo * | a | ) | [inline] |
References memoryDimAlgo.
Referenced by FSMSADF::mapApplicationGraphToArchitectureGraph().
void FSMSADF::SDF3Flow::setNextStateOfFlow | ( | FlowState | s | ) | [inline, private] |
void FSMSADF::SDF3Flow::setStepMode | ( | bool | flag | ) | [inline] |
References stepMode.
Referenced by handleUserInteraction(), and FSMSADF::mapApplicationGraphToArchitectureGraph().
void FSMSADF::SDF3Flow::setTileBindingAlgo | ( | TileBindingAlgo * | a | ) | [inline] |
References tileBindingAlgo.
Referenced by FSMSADF::mapApplicationGraphToArchitectureGraph().
Member Data Documentation
Graph* FSMSADF::SDF3Flow::applicationGraph [private] |
Referenced by constructFromXML(), convertToXML(), getApplicationGraph(), and ~SDF3Flow().
FlowType FSMSADF::SDF3Flow::flowType [private] |
Referenced by getFlowType().
Referenced by getMaxNrBindingsTileBindingAlgo(), and setMaxNrBindingsTileBindingAlgo().
MemoryDimAlgo* FSMSADF::SDF3Flow::memoryDimAlgo [private] |
Referenced by checkInputDesignFlow(), constructFromXML(), convertToXML(), getPlatformBindings(), and ~SDF3Flow().
PlatformGraph* FSMSADF::SDF3Flow::platformGraph [private] |
Referenced by constructFromXML(), convertToXML(), getPlatformGraph(), and ~SDF3Flow().
FlowState FSMSADF::SDF3Flow::stateOfFlow [private] |
Referenced by getStateOfFlow(), and setNextStateOfFlow().
bool FSMSADF::SDF3Flow::stepMode [private] |
Referenced by getStepMode(), and setStepMode().
The documentation for this class was generated from the following files: