FSMSADF::TileBindingAlgo Class Reference
#include <binding.h>
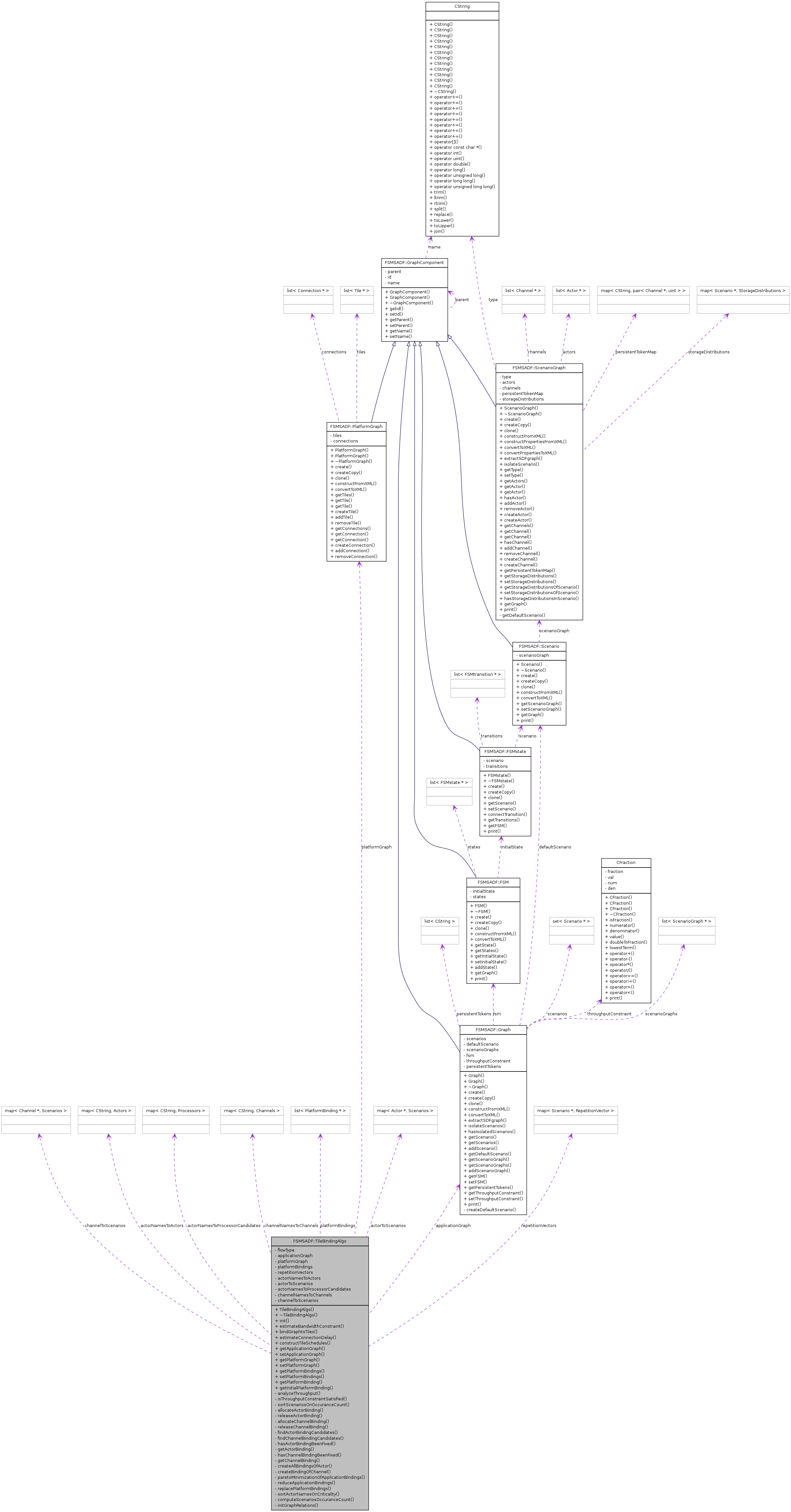
Detailed Description
Tile binding and scheduling
Constructor & Destructor Documentation
FSMSADF::TileBindingAlgo::TileBindingAlgo | ( | FlowType & | t | ) |
TileBindingAlgo() Constructor.
FSMSADF::TileBindingAlgo::~TileBindingAlgo | ( | ) |
~TileBindingAlgo() Destructor.
Member Function Documentation
bool FSMSADF::TileBindingAlgo::allocateActorBinding | ( | GraphBinding * | applicationBinding, | |
CString & | name, | |||
ActorBinding & | actorBinding | |||
) | [private] |
allocateActorBinding() The function creates an actor bindings for the actor with the supplied name.
bool FSMSADF::TileBindingAlgo::allocateChannelBinding | ( | GraphBinding * | applicationBinding, | |
CString & | name, | |||
ChannelBinding & | channelBinding | |||
) | [private] |
allocateChannelBinding() The function creates a channel binding for all channels which match the supplied name.
Throughput FSMSADF::TileBindingAlgo::analyzeThroughput | ( | PlatformBinding * | pb | ) | [private] |
analyzeThroughput () The function returns the throughput of the application graph mapped onto the platform graph.
bool FSMSADF::TileBindingAlgo::bindGraphtoTiles | ( | const uint | maxNrAppBindings | ) |
bindGraphtoTiles() Bind the application graph to the platform tiles. The function produces Pareto trade-off space with different platform bindings. The number of bindings are outputted by the function does not exceed the maxNrAppBindings.
Referenced by FSMSADF::SDF3Flow::bindGraphtoTiles().
map< Scenario *, uint > FSMSADF::TileBindingAlgo::computeScenariosOccuranceCount | ( | Graph * | g | ) | const [private] |
computeScenariosOccuranceCount() The function returns the occurance frequency of the scenarios in the FSM.
bool FSMSADF::TileBindingAlgo::constructTileSchedules | ( | const uint | maxNrAppBindings | ) |
constructTileSchedules() Construct static-order and TDMA schedules for the application on all platform tiles.
Referenced by FSMSADF::SDF3Flow::constructTileSchedules().
void FSMSADF::TileBindingAlgo::createAllBindingsOfActor | ( | CString & | name, | |
GraphBindings & | bindings | |||
) | [private] |
createAllBindingsOfActor() The function returns all possible bindings of the actors with the supplied name on the platform using the (partial) bindings given in the list bindings.
bool FSMSADF::TileBindingAlgo::createBindingOfChannel | ( | CString & | name, | |
GraphBinding * | b | |||
) | [private] |
createBindingOfChannel() The function tries to bind the channels with the supplied name to the platform using the binding b. A binding can only be made when the source and destination actor of the channel are already bound to a tile. The function will try all possible bindings till a valid binding is found. When no valid binding exists (due to resource constraints), the function returns false. Otherwise the function returns true. So, the function also returns true when no binding can be made because the source or destination actor is not bound.
bool FSMSADF::TileBindingAlgo::estimateBandwidthConstraint | ( | ) |
estimateBandwidthConstraint() Estimate the required bandwidth for each channel in the application graph.
Referenced by FSMSADF::SDF3Flow::estimateBandwidthConstraint().
bool FSMSADF::TileBindingAlgo::estimateConnectionDelay | ( | ) |
estimateConnectionDelay() Estimate the channel delay of channels which have been mapped to a connection.
Referenced by FSMSADF::SDF3Flow::estimateConnectionDelay().
ActorBindings FSMSADF::TileBindingAlgo::findActorBindingCandidates | ( | GraphBinding * | applicationBinding, | |
Actor * | a | |||
) | [private] |
findActorBindingCandidates() The function returns a list with all possible actor bindings. Note that it is not guaranteed that sufficient resources are available to actually create a binding.
ChannelBindings FSMSADF::TileBindingAlgo::findChannelBindingCandidates | ( | GraphBinding * | applicationBinding, | |
Channel * | c | |||
) | [private] |
findChannelBindingCandidates() The function returns a list with all possible channel bindings. Note that it is not guaranteed that sufficient resources are available to actually create a binding. Channel binding candidates can only be created when the source and destination actor of the channel are already mapped to a processor.
ActorBinding FSMSADF::TileBindingAlgo::getActorBinding | ( | GraphBinding * | applicationBinding, | |
const CString & | name | |||
) | const [private] |
getActorBinding() The function returns an actor binding of an actor with the supplied name. When no binding of an actor with this name exists, an exception is thrown.
Graph* FSMSADF::TileBindingAlgo::getApplicationGraph | ( | ) | const [inline] |
References applicationGraph.
ChannelBinding FSMSADF::TileBindingAlgo::getChannelBinding | ( | GraphBinding * | applicationBinding, | |
const CString & | name | |||
) | const [private] |
getChannelBinding() The function returns a chanenl binding of a channel with the supplied name. When no binding of a channel with this name exists, an exception is thrown.
PlatformBinding * FSMSADF::TileBindingAlgo::getInitialPlatformBinding | ( | ) | const |
getInitialPlatformBinding() The function returns a pointer to the initial platform binding object. An exception is thrown when no such object exists.
PlatformBinding * FSMSADF::TileBindingAlgo::getPlatformBinding | ( | const CString & | name | ) | const |
getPlatformBinding() The function returns a pointer to a platform binding object with the specified name. An exception is thrown when no such object exists.
PlatformBindings* FSMSADF::TileBindingAlgo::getPlatformBindings | ( | ) | const [inline] |
References platformBindings.
PlatformGraph* FSMSADF::TileBindingAlgo::getPlatformGraph | ( | ) | const [inline] |
References platformGraph.
bool FSMSADF::TileBindingAlgo::hasActorBindingBeenFixed | ( | GraphBinding * | applicationBinding, | |
const CString & | name | |||
) | const [private] |
hasActorBindingBeenFixed() The function returns true when an actor with the supplied name has been bound to the platform in at least one scenario. Otherwise the function return false.
bool FSMSADF::TileBindingAlgo::hasChannelBindingBeenFixed | ( | GraphBinding * | applicationBinding, | |
const CString & | name | |||
) | const [private] |
hasChannelBindingBeenFixed() The function returns true when a channel with the supplied name has been bound to the platform in at least one scenario. Otherwise the function return false.
void FSMSADF::TileBindingAlgo::init | ( | ) |
initialize() The function initializes the tile binding and scheduling algorithm.
Referenced by FSMSADF::SDF3Flow::checkInputDesignFlow().
void FSMSADF::TileBindingAlgo::initGraphRelations | ( | ) | [private] |
initGraphRelations() Initialize the relations between the application and platform graph.
bool FSMSADF::TileBindingAlgo::isThroughputConstraintSatisfied | ( | PlatformBinding * | pb | ) | [private] |
isThroughputConstraintSatisfied () Check wether or not the throughput constraint is satisfied.
void FSMSADF::TileBindingAlgo::paretoMinimizationOfApplicationBindings | ( | GraphBindings & | applicationBindings | ) | [private] |
paretoMinimizationOfApplicationBindings() The function removes all non-Pareto application bindings from the list of application bindings. The function implements the Simple Cull algorithm.
void FSMSADF::TileBindingAlgo::reduceApplicationBindings | ( | GraphBindings & | applicationBindings, | |
const uint | maxNrAppBindings | |||
) | [private] |
reduceApplicationBindings() The function reduces the number of application bindings in the list to the specified maximum.
void FSMSADF::TileBindingAlgo::releaseActorBinding | ( | GraphBinding * | applicationBinding, | |
CString & | name | |||
) | [private] |
releaseActorBinding() The function removes the specified actor binding.
void FSMSADF::TileBindingAlgo::releaseChannelBinding | ( | GraphBinding * | applicationBinding, | |
CString & | name | |||
) | [private] |
releaseChannelBinding() The function removes the specified channel binding.
void FSMSADF::TileBindingAlgo::replacePlatformBindings | ( | GraphBindings | newBindings | ) | [private] |
replacePlatformBindings() The function replaces the existing platform bindings with the platform bindings given by the graph bindings. The initial binding (if present) is preserved.
void FSMSADF::TileBindingAlgo::setApplicationGraph | ( | Graph * | g | ) | [inline] |
References applicationGraph.
Referenced by FSMSADF::SDF3Flow::checkInputDesignFlow().
void FSMSADF::TileBindingAlgo::setPlatformBindings | ( | PlatformBindings * | b | ) | [inline] |
References platformBindings.
Referenced by FSMSADF::SDF3Flow::checkInputDesignFlow().
void FSMSADF::TileBindingAlgo::setPlatformGraph | ( | PlatformGraph * | g | ) | [inline] |
References platformGraph.
Referenced by FSMSADF::SDF3Flow::checkInputDesignFlow().
list< CString > FSMSADF::TileBindingAlgo::sortActorNamesOnCriticality | ( | ) | [private] |
sortActorNamesOnCriticality() The function returns a list of actor names in which those names have been ordered (high-to-low) based on the criticality of the actors.
list< Scenario * > FSMSADF::TileBindingAlgo::sortScenariosOnOccuranceCount | ( | Graph * | g | ) | const [private] |
sortScenariosOnOccuranceCount() The function returns a list where the scenarios in the graph g are sorted on their occurance frequency in the FSM. The ordering is from high to low.
Member Data Documentation
map<CString, Actors > FSMSADF::TileBindingAlgo::actorNamesToActors [private] |
map<CString, Processors > FSMSADF::TileBindingAlgo::actorNamesToProcessorCandidates [private] |
map<Actor*, Scenarios > FSMSADF::TileBindingAlgo::actorToScenarios [private] |
Graph* FSMSADF::TileBindingAlgo::applicationGraph [private] |
Referenced by getApplicationGraph(), and setApplicationGraph().
map<CString, Channels > FSMSADF::TileBindingAlgo::channelNamesToChannels [private] |
map<Channel*, Scenarios > FSMSADF::TileBindingAlgo::channelToScenarios [private] |
FlowType FSMSADF::TileBindingAlgo::flowType [private] |
Referenced by getPlatformBindings(), and setPlatformBindings().
Referenced by getPlatformGraph(), and setPlatformGraph().
map<Scenario*, RepetitionVector > FSMSADF::TileBindingAlgo::repetitionVectors [private] |
The documentation for this class was generated from the following files: