LoadBalanceBinding Class Reference
#include <loadbalance.h>
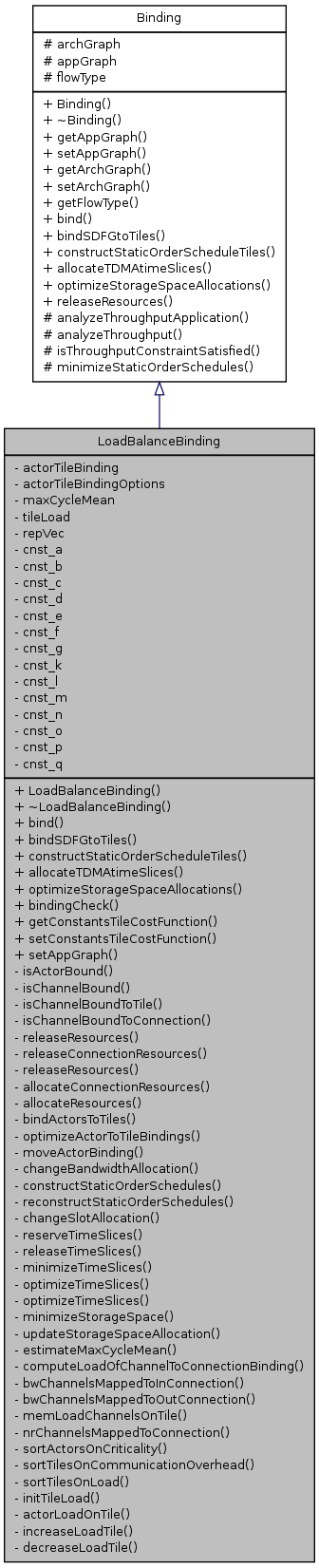
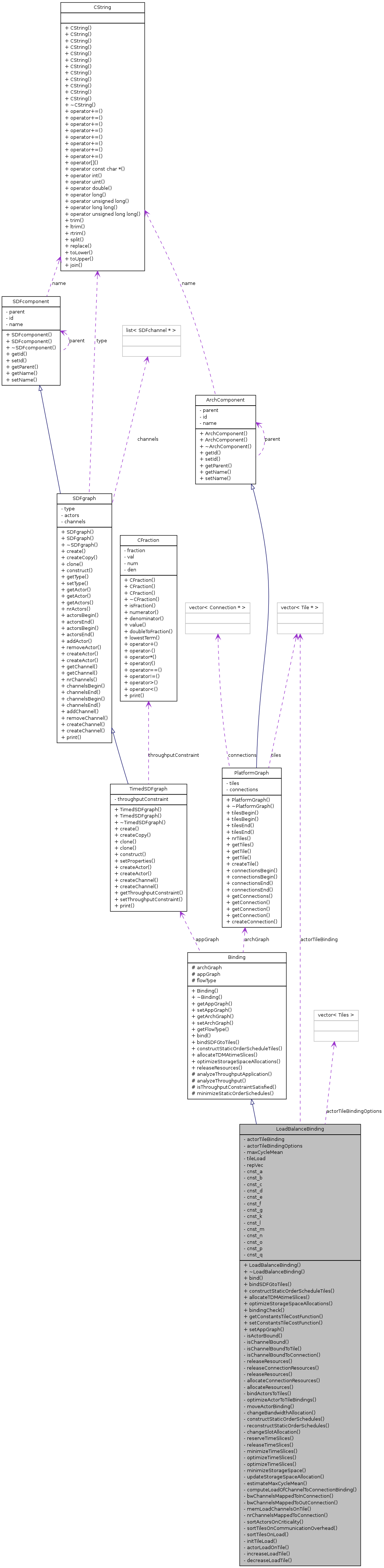
Detailed Description
LoadBalanceBinding () Load balance resource assignment algorithm.
Constructor & Destructor Documentation
LoadBalanceBinding::LoadBalanceBinding | ( | SDFflowType | flowType | ) |
LoadBalanceBinding () Constructor.
References maxCycleMean, setConstantsTileCostFunction(), and tileLoad.

LoadBalanceBinding::~LoadBalanceBinding | ( | ) |
~LoadBalanceBinding () Destructor.
References maxCycleMean, and tileLoad.
Member Function Documentation
double LoadBalanceBinding::actorLoadOnTile | ( | TimedSDFactor * | a, | |
Tile * | t | |||
) | [private] |
actorLoadOnTile () The function returns the load of an actor on a tile. The load is defined as the execution time of the actor per firing times the number of firings required per period.
References SDFcomponent::getId(), TimedSDFactor::getProcessor(), Tile::getProcessor(), Processor::getType(), and repVec.
Referenced by decreaseLoadTile(), increaseLoadTile(), and sortTilesOnLoad().
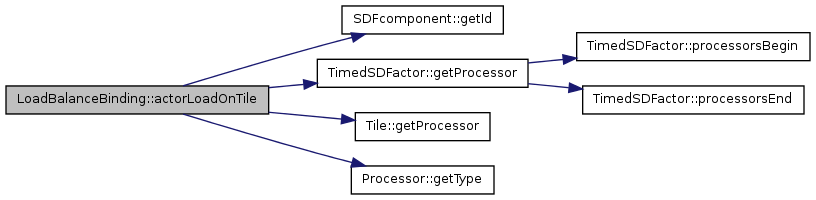
bool LoadBalanceBinding::allocateConnectionResources | ( | TimedSDFchannel * | c | ) | [private] |
allocateConnectionResources () The function allocates all connection resources needed by a channel.
References actorTileBinding, Binding::archGraph, Connection::bindChannel(), TimedSDFchannel::getBufferSize(), PlatformGraph::getConnections(), SDFchannel::getDstActor(), SDFcomponent::getId(), Tile::getMemory(), TimedSDFchannel::getMinBandwidth(), ArchComponent::getName(), SDFcomponent::getName(), Tile::getNetworkInterface(), SDFchannel::getSrcActor(), TimedSDFchannel::getTokenSize(), NetworkInterface::reserveConnection(), and Memory::reserveMemory().
Referenced by allocateResources().
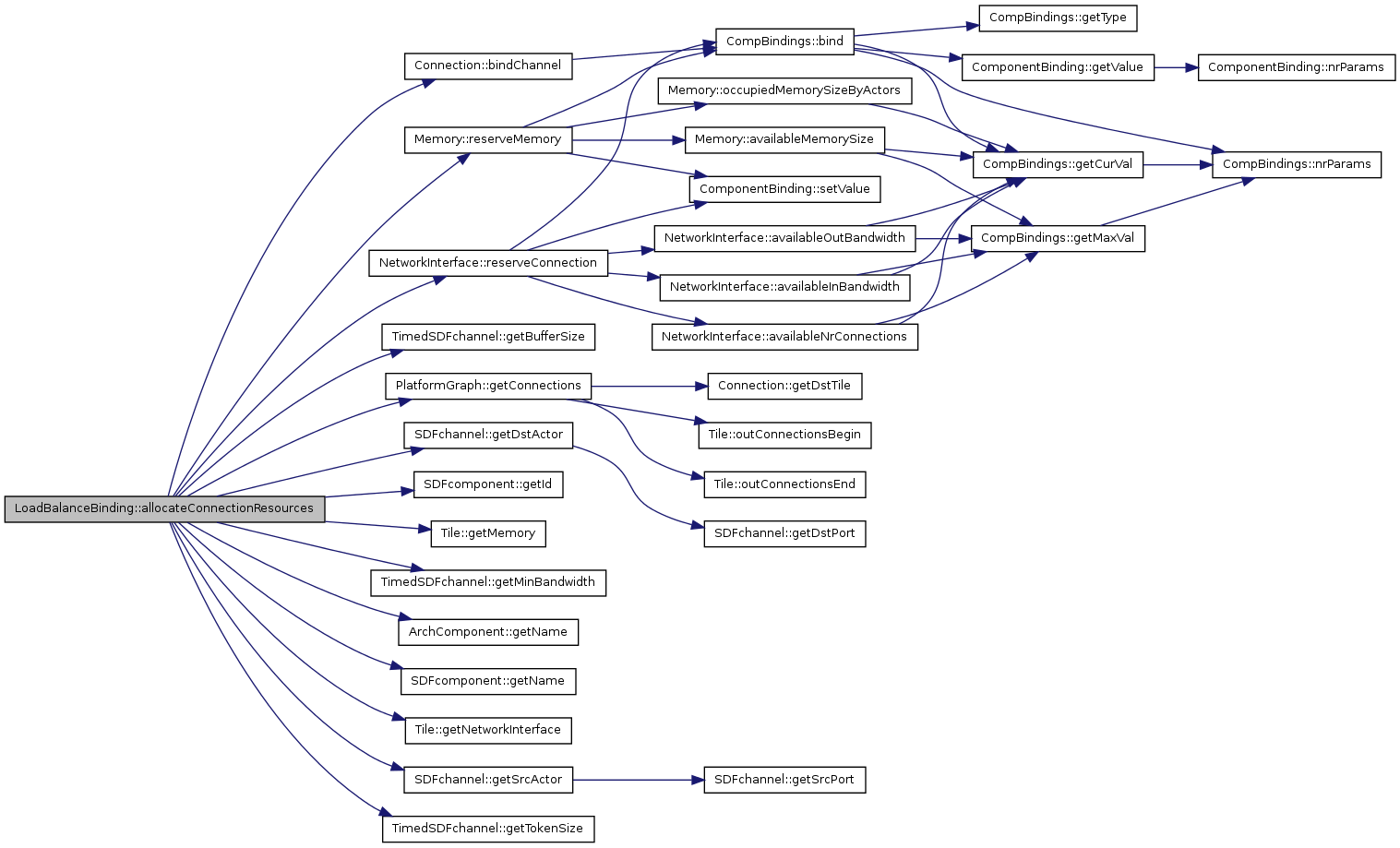
bool LoadBalanceBinding::allocateResources | ( | TimedSDFactor * | a, | |
Tile * | t | |||
) | [private] |
allocateResources () The function attempts to allocate resources for actor a on the tile t. The allocated time slice is equal to the actors execution time.
References actorTileBinding, allocateConnectionResources(), Processor::availableTimewheelSize(), Processor::bindActor(), c, TimedSDFchannel::getBufferSize(), SDFcomponent::getId(), Tile::getMemory(), ArchComponent::getName(), SDFcomponent::getName(), Tile::getNetworkInterface(), Tile::getProcessor(), TimedSDFactor::getStateSize(), TimedSDFchannel::getTokenSize(), increaseLoadTile(), isChannelBoundToConnection(), isChannelBoundToTile(), SDFactor::portsBegin(), SDFactor::portsEnd(), releaseResources(), and Memory::reserveMemory().
Referenced by bindActorsToTiles(), and moveActorBinding().
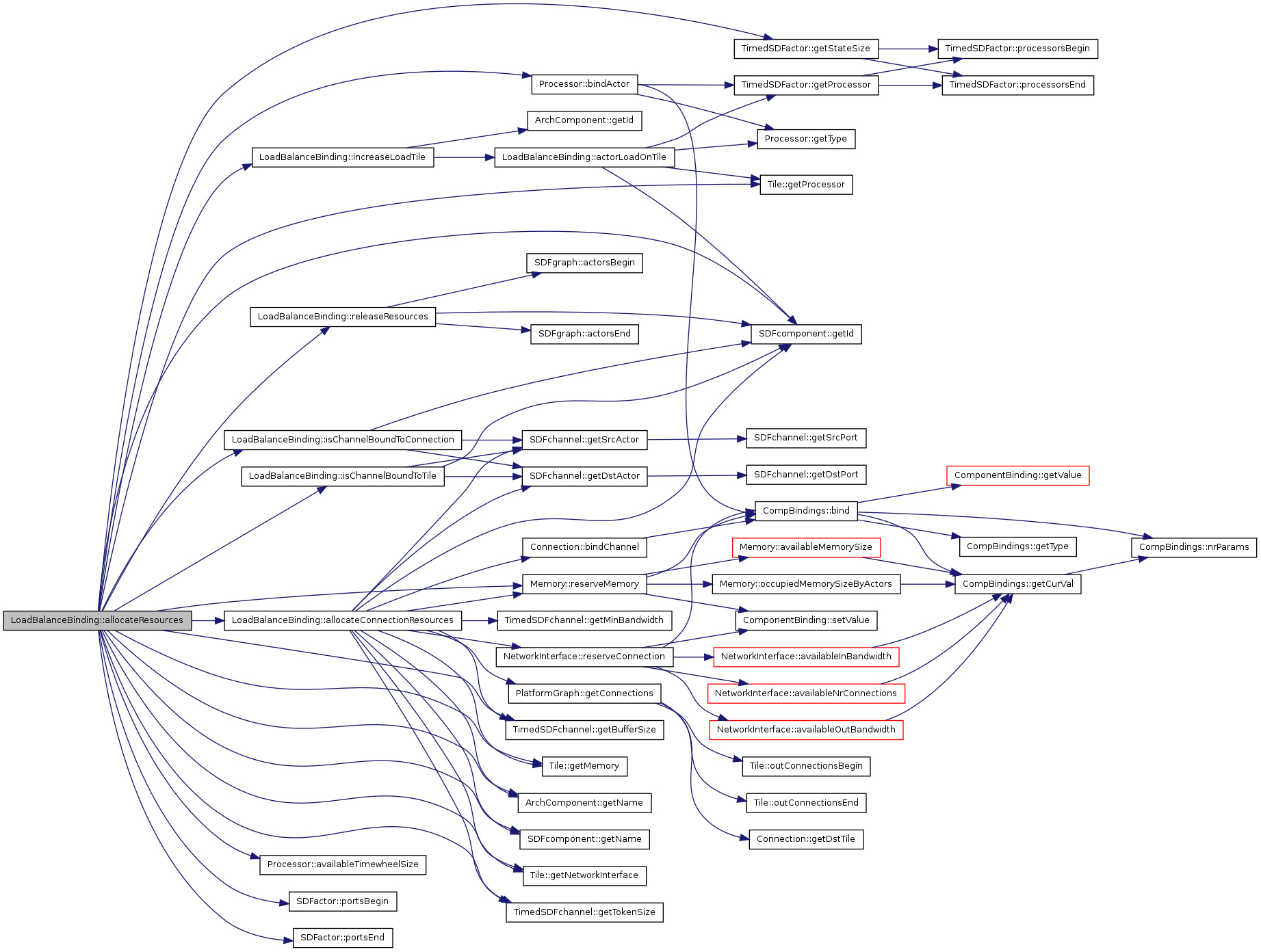
bool LoadBalanceBinding::allocateTDMAtimeSlices | ( | ) | [virtual] |
allocateTDMAtimeSlices ()
Implements Binding.
References a, SDFgraph::actorsBegin(), SDFgraph::actorsEnd(), actorTileBinding, Binding::analyzeThroughput(), Binding::appGraph, Binding::archGraph, SDFcomponent::getId(), ArchComponent::getId(), ArchComponent::getName(), Binding::isThroughputConstraintSatisfied(), minimizeTimeSlices(), optimizeTimeSlices(), releaseResources(), reserveTimeSlices(), PlatformGraph::tilesBegin(), and PlatformGraph::tilesEnd().
Referenced by bind(), and bindApplicationGraphsToArchitectureGraph().
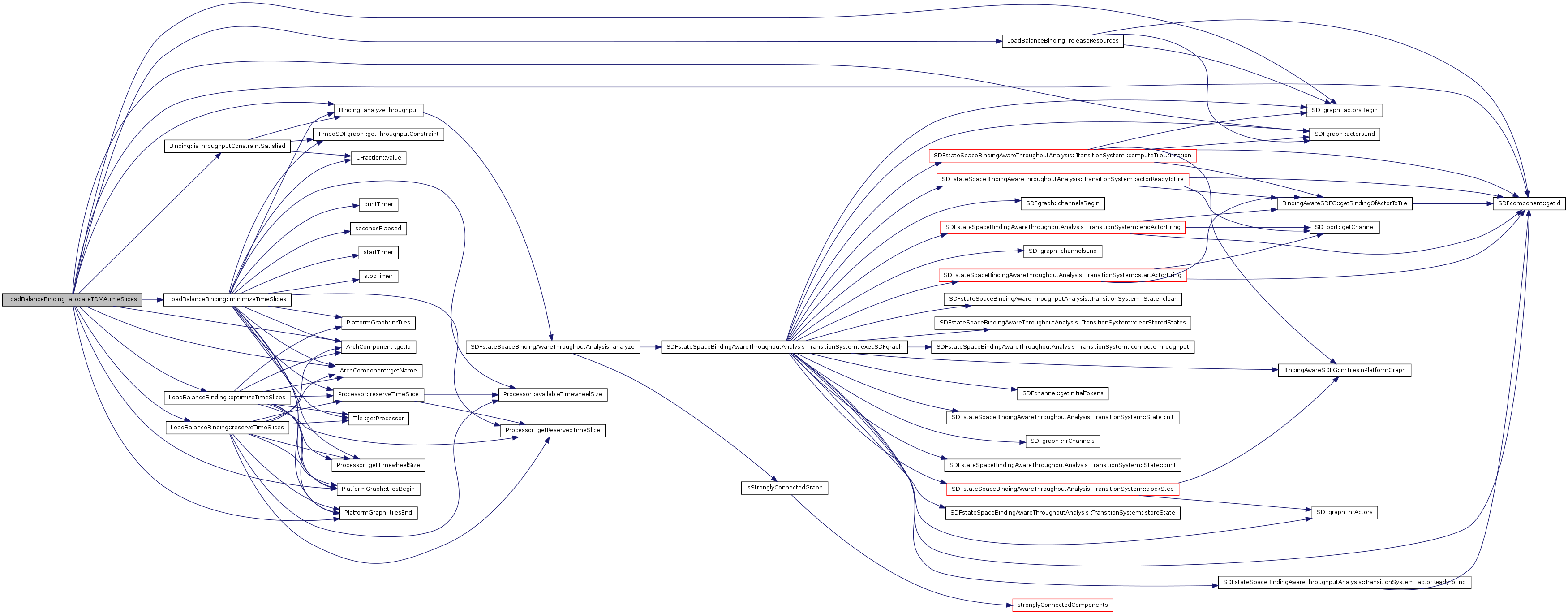
bool LoadBalanceBinding::bind | ( | ) | [virtual] |
bind () Load balance binding algorithm.
Implements Binding.
References allocateTDMAtimeSlices(), bindSDFGtoTiles(), constructStaticOrderScheduleTiles(), and optimizeStorageSpaceAllocations().
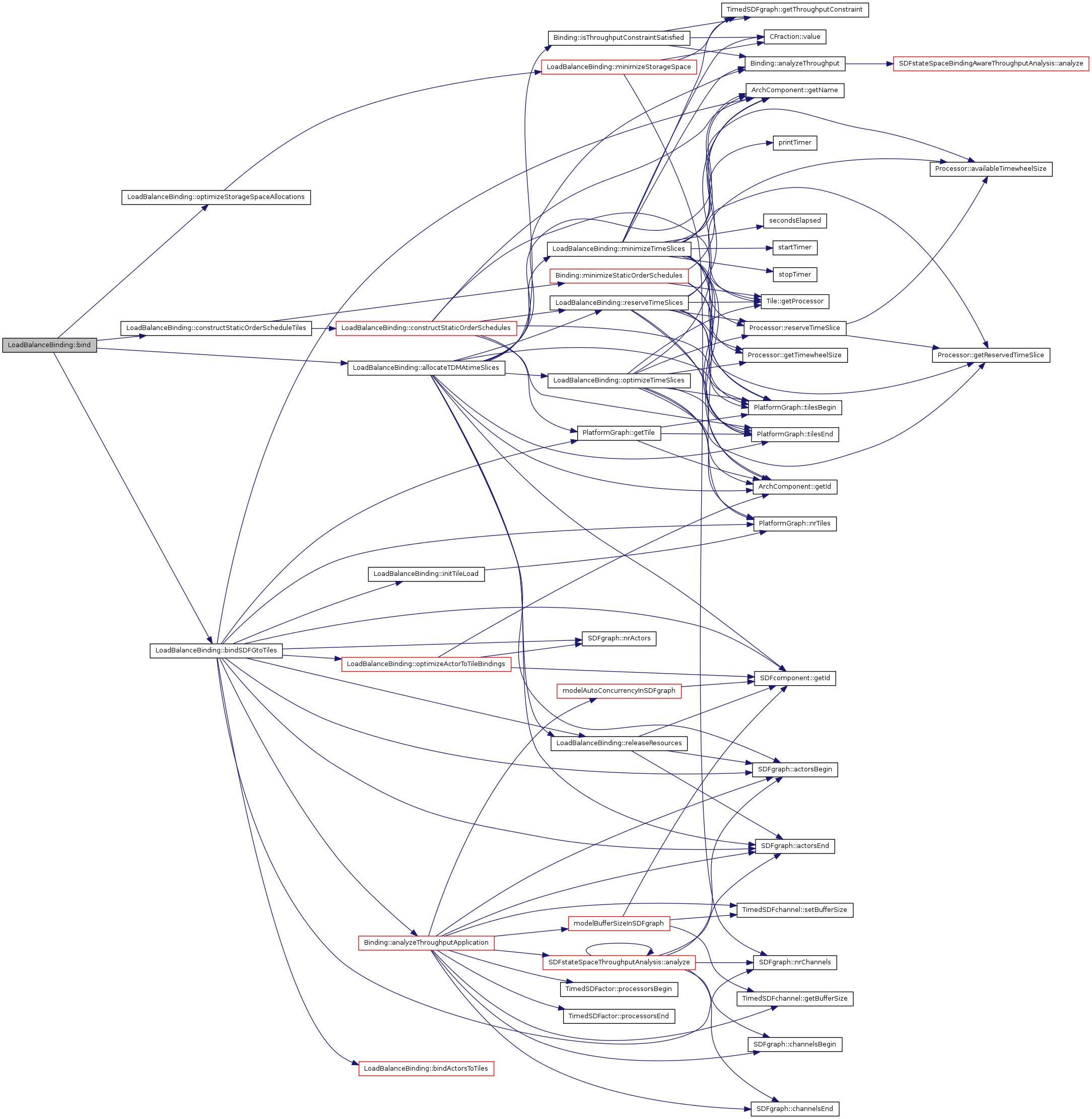
bool LoadBalanceBinding::bindActorsToTiles | ( | ) | [private] |
bindActorsToTiles () Bind each actor to a tile. Actors are handled in order of their criticality and tiles are tried in order of their load. The objective is to spread the load evenly over all tiles.
References a, allocateResources(), Binding::archGraph, cnst_a, cnst_b, cnst_c, cnst_d, cnst_e, cnst_f, cnst_g, cnst_k, cnst_l, cnst_m, cnst_n, cnst_o, cnst_p, cnst_q, SDFcomponent::getName(), PlatformGraph::getTiles(), sortActorsOnCriticality(), and sortTilesOnLoad().
Referenced by bindingCheck(), and bindSDFGtoTiles().
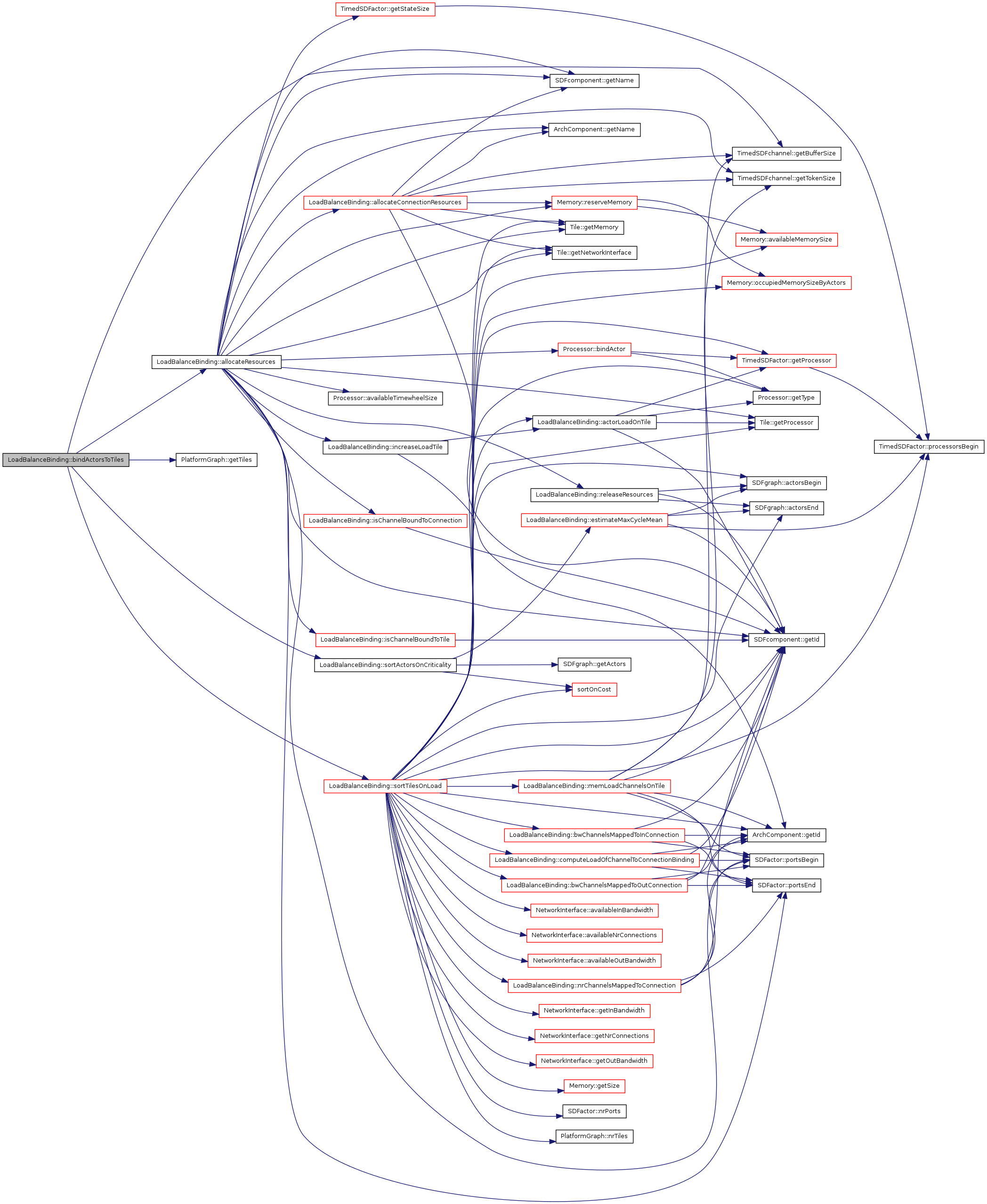
bool LoadBalanceBinding::bindingCheck | ( | ) |
bindingCheck () Run only binding step of the load balance binding algorithm.
References actorTileBinding, Binding::appGraph, bindActorsToTiles(), cnst_a, cnst_b, cnst_c, cnst_d, cnst_e, cnst_f, cnst_g, cnst_k, cnst_l, cnst_m, cnst_n, cnst_o, cnst_p, cnst_q, initTileLoad(), Binding::isThroughputConstraintSatisfied(), SDFgraph::nrActors(), and reserveTimeSlices().
Referenced by bindApplicationGraphsToArchitectureGraph().
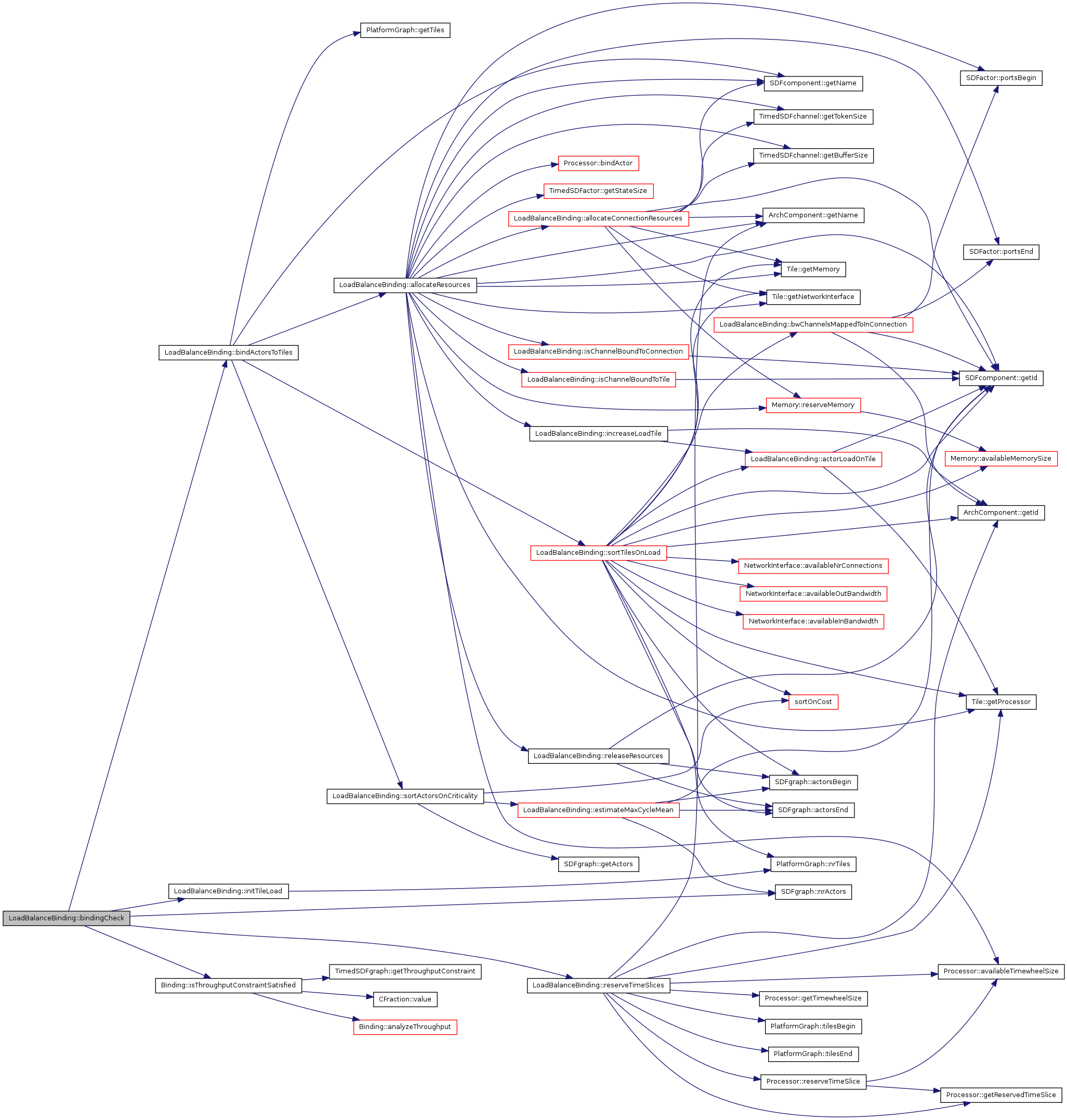
bool LoadBalanceBinding::bindSDFGtoTiles | ( | ) | [virtual] |
bindSDFGtoTiles ()
Implements Binding.
References a, SDFgraph::actorsBegin(), SDFgraph::actorsEnd(), actorTileBinding, Binding::analyzeThroughputApplication(), Binding::appGraph, Binding::archGraph, bindActorsToTiles(), cnst_a, cnst_b, cnst_c, cnst_d, cnst_e, cnst_f, cnst_g, cnst_k, cnst_l, cnst_m, cnst_n, cnst_o, cnst_p, cnst_q, SDFcomponent::getId(), ArchComponent::getName(), PlatformGraph::getTile(), initTileLoad(), SDFgraph::nrActors(), SDFgraph::nrChannels(), PlatformGraph::nrTiles(), optimizeActorToTileBindings(), releaseResources(), repVec, and tileLoad.
Referenced by bind(), and bindApplicationGraphsToArchitectureGraph().
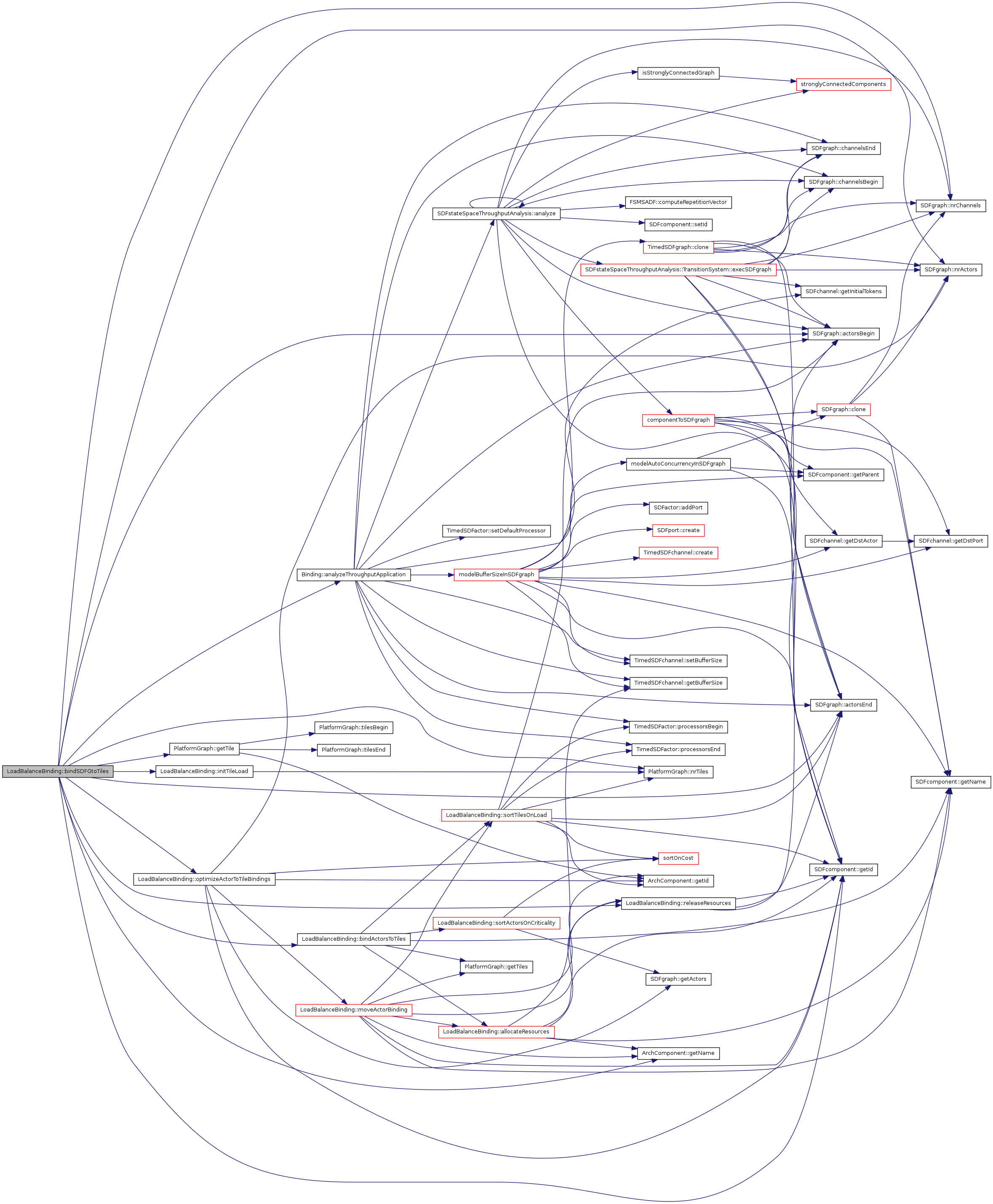
bwChannelsMappedToInConnection () The function returns the bandwidth of channels connected to the actor a which will be mapped to an ingoing connection if the actor is mapped to tile t. Channels of which the other actor is not mapped are ignored.
References actorTileBinding, c, SDFport::getActor(), SDFport::getChannel(), ArchComponent::getId(), SDFcomponent::getId(), TimedSDFchannel::getMinBandwidth(), SDFport::getType(), SDFchannel::oppositePort(), SDFactor::portsBegin(), and SDFactor::portsEnd().
Referenced by sortTilesOnLoad().
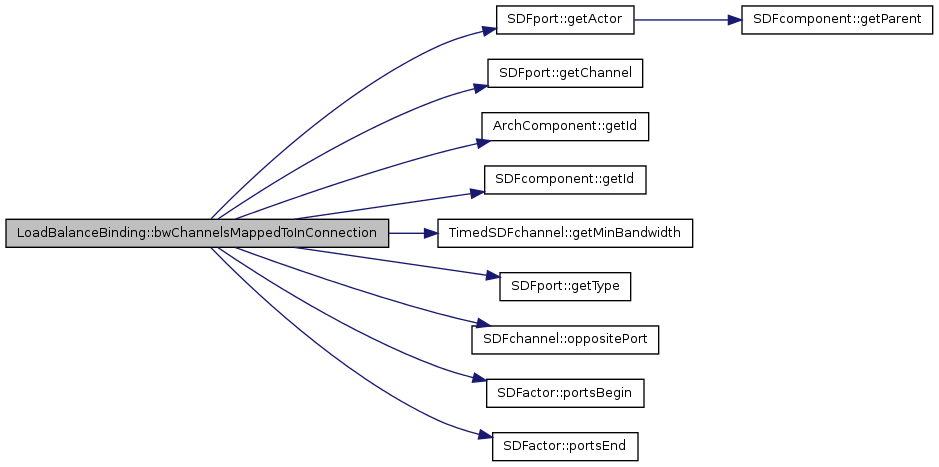
bwChannelsMappedToOutConnection () The function returns the bandwidth of channels connected to the actor a which will be mapped to an outgoing connection if the actor is mapped to tile t. Channels of which the other actor is not mapped are ignored.
References actorTileBinding, c, SDFport::getActor(), SDFport::getChannel(), ArchComponent::getId(), SDFcomponent::getId(), TimedSDFchannel::getMinBandwidth(), SDFport::getType(), SDFchannel::oppositePort(), SDFactor::portsBegin(), and SDFactor::portsEnd().
Referenced by sortTilesOnLoad().
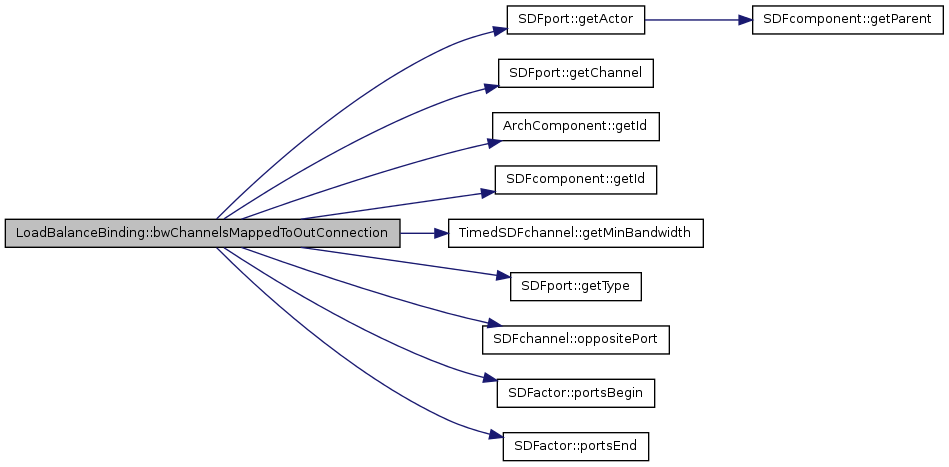
bool LoadBalanceBinding::changeBandwidthAllocation | ( | TimedSDFchannel * | c, | |
double | bw | |||
) | [private] |
changeBandwidthAllocation () The function modifies the bandwidth allocation of a channel. On success, it return true. On failure, it returns false and the channel keeps the original bandwidth assignment.
References actorTileBinding, NetworkInterface::availableInBandwidth(), NetworkInterface::availableOutBandwidth(), CompBindings::find(), NetworkInterface::getBindings(), SDFchannel::getDstActor(), SDFcomponent::getId(), SDFcomponent::getName(), Tile::getNetworkInterface(), SDFchannel::getSrcActor(), ComponentBinding::getValue(), NetworkInterface::outBw, NetworkInterface::releaseConnection(), and NetworkInterface::reserveConnection().
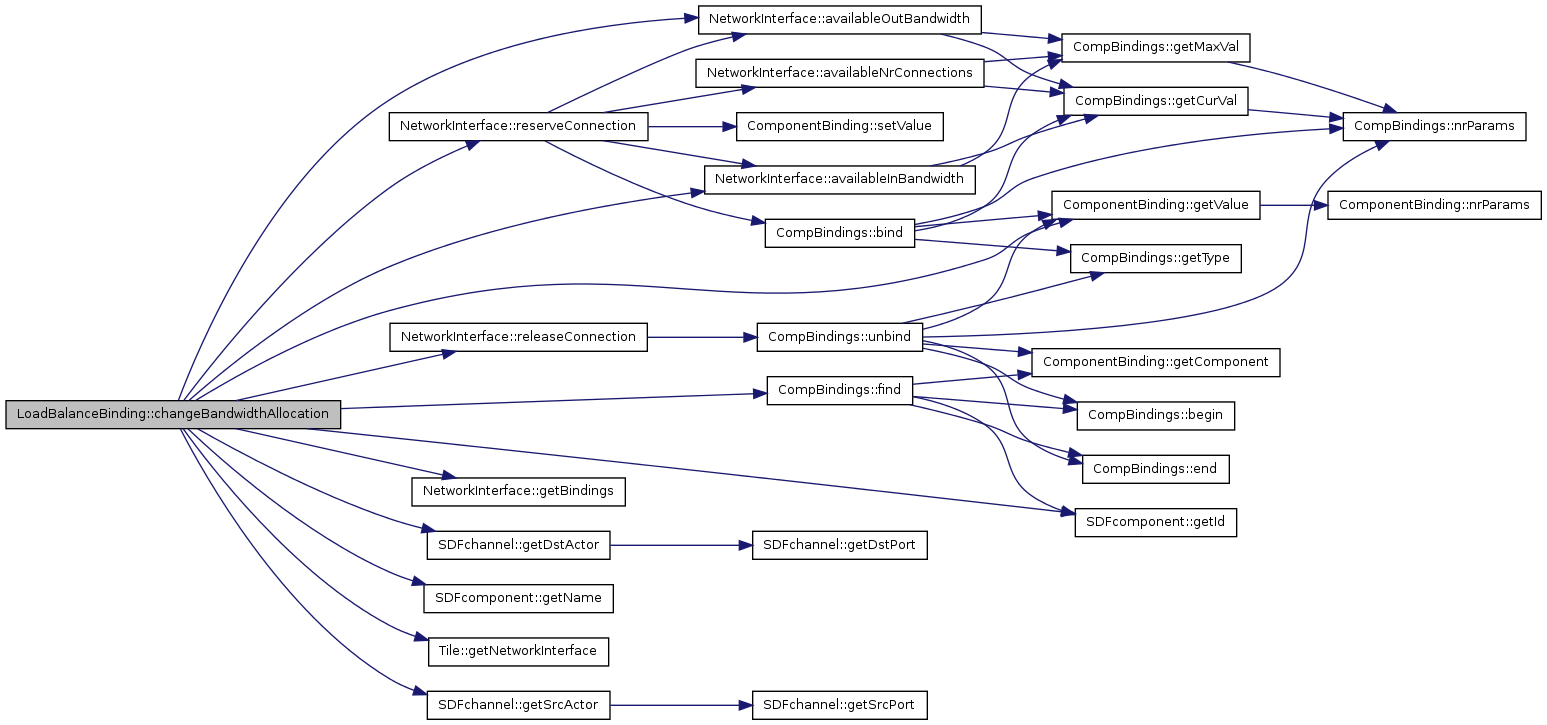
changeSlotAllocation () The function changes the size of the TDMA slot allocation of the tile to the given size. On failure, the original allocation is maintained and false is returned. On success, the function returns true.
References Processor::availableTimewheelSize(), Tile::getProcessor(), Processor::getReservedTimeSlice(), Processor::releaseTimeSlice(), and Processor::reserveTimeSlice().
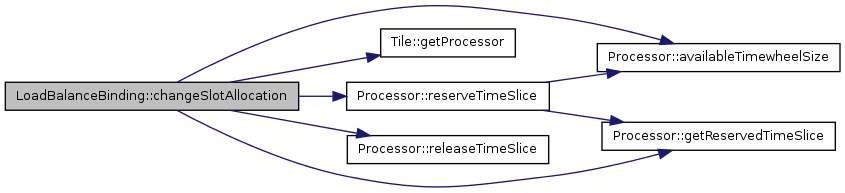
double LoadBalanceBinding::computeLoadOfChannelToConnectionBinding | ( | SDFactor * | a, | |
Tile * | t | |||
) | [private] |
computeLoadOfChannelToConnectionBinding () The function computes the cost of binding actor a to tile t in terms of the latency of the created connections. It computes the sum of the latency of all channels that are bound to a connection by binding actor a to tile t.
References actorTileBinding, Binding::archGraph, c, SDFport::getActor(), SDFport::getChannel(), PlatformGraph::getConnection(), ArchComponent::getId(), SDFcomponent::getId(), Connection::getLatency(), ArchComponent::getParent(), SDFport::getType(), SDFchannel::oppositePort(), SDFactor::portsBegin(), and SDFactor::portsEnd().
Referenced by sortTilesOnLoad().
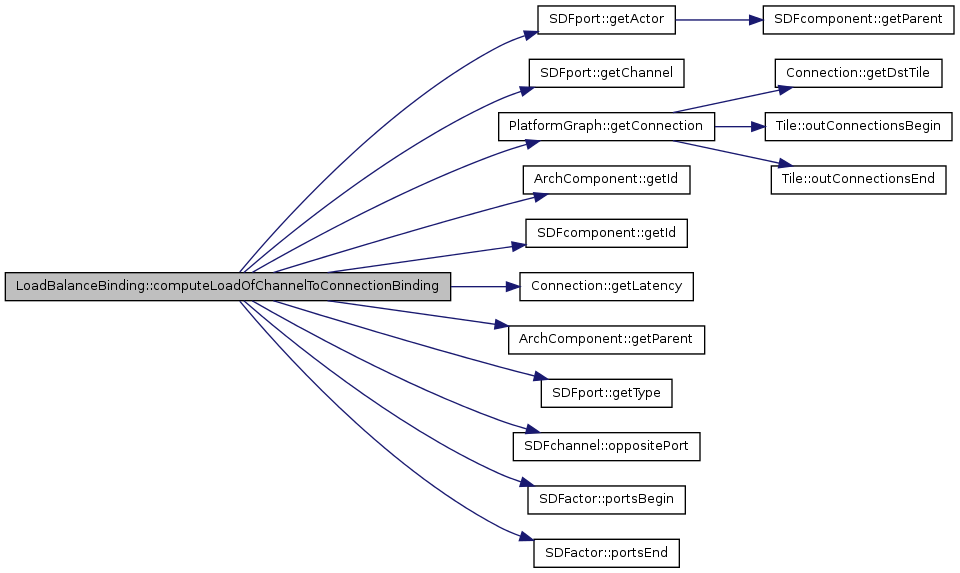
void LoadBalanceBinding::constructStaticOrderSchedules | ( | ) | [private] |
constructStaticOrderSchedules () The function generates a static-order schedule for each processor in the architecture graph. The schedules order the firing of the actors in the application graph. They respects the buffer requirements and mapping of all actors.
References Binding::appGraph, Binding::archGraph, StaticOrderSchedule::changeActorAssociations(), Binding::getFlowType(), ArchComponent::getName(), Tile::getProcessor(), Processor::getSchedule(), BindingAwareSDFG::getScheduleOnTile(), PlatformGraph::getTile(), logMsg(), BindingAwareSDFG::nrTilesInPlatformGraph(), releaseTimeSlices(), reserveTimeSlices(), SDFstateSpaceListScheduler::schedule(), Processor::setSchedule(), PlatformGraph::tilesBegin(), and PlatformGraph::tilesEnd().
Referenced by constructStaticOrderScheduleTiles().
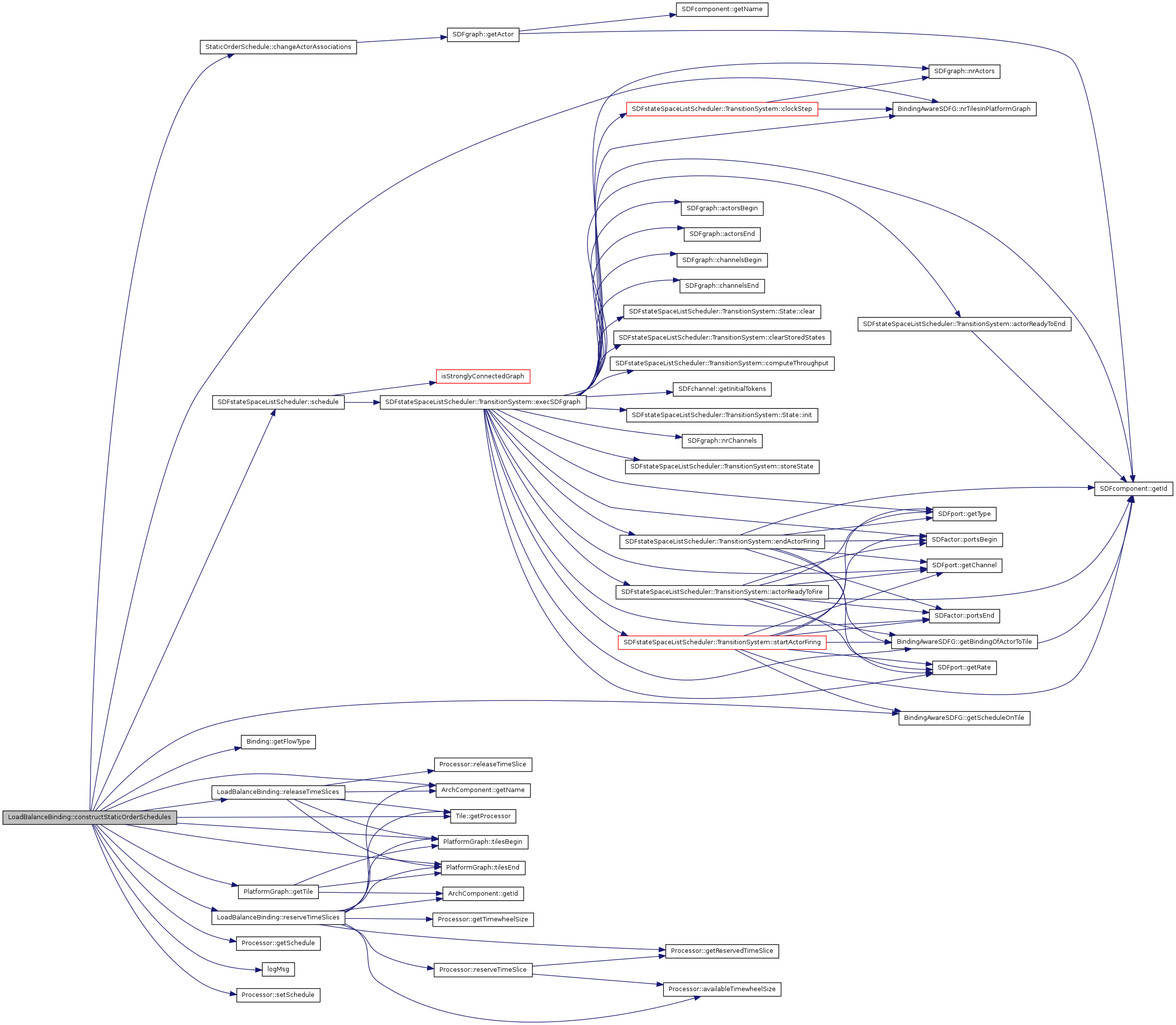
bool LoadBalanceBinding::constructStaticOrderScheduleTiles | ( | ) | [virtual] |
constructStaticOrderScheduleTiles ()
Implements Binding.
References Binding::archGraph, constructStaticOrderSchedules(), and Binding::minimizeStaticOrderSchedules().
Referenced by bind().
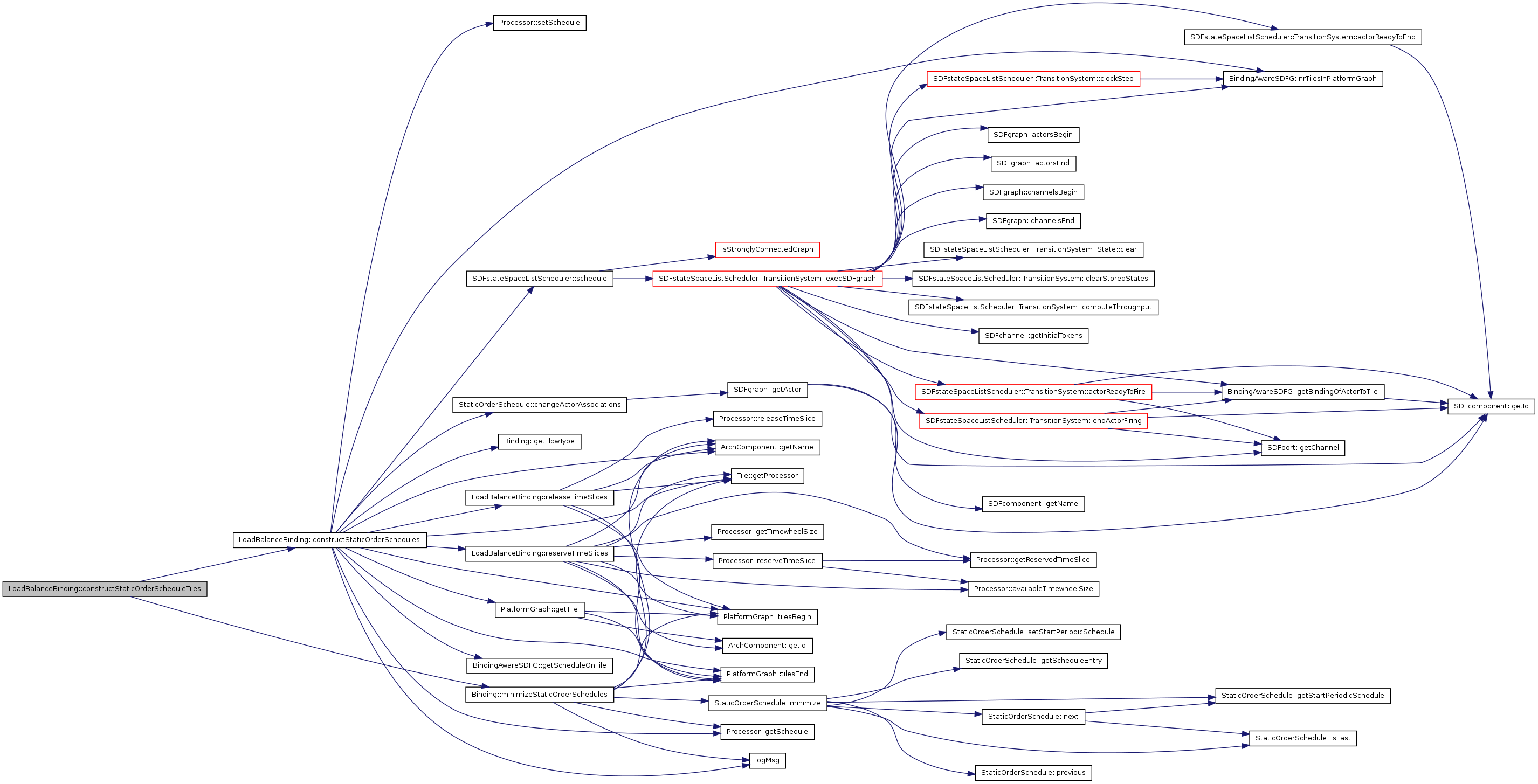
void LoadBalanceBinding::decreaseLoadTile | ( | TimedSDFactor * | a, | |
Tile * | t | |||
) | [private] |
decreaseLoadTile () Remove the load of an actor from a tile. The load is defined as the execution time of the actor per firing times the number of firings required per period.
References actorLoadOnTile(), ASSERT, ArchComponent::getId(), and tileLoad.
Referenced by releaseResources().

void LoadBalanceBinding::estimateMaxCycleMean | ( | ) | [private] |
estimateMaxCycleMean () The function estimates the maximum cycle mean of all actors in the application graph.
References a, SDFgraph::actorsBegin(), SDFgraph::actorsEnd(), Binding::appGraph, c, SDFgraph::channelsBegin(), SDFgraph::channelsEnd(), TimedSDFactor::_Processor::execTime, findSimpleCycles(), SDFchannel::getDstActor(), SDFchannel::getDstPort(), SDFcomponent::getId(), SDFchannel::getInitialTokens(), SDFport::getRate(), SDFchannel::getSrcActor(), maxCycleMean, SDFgraph::nrActors(), TimedSDFactor::nrProcessors(), TimedSDFactor::processorsBegin(), TimedSDFactor::processorsEnd(), and repVec.
Referenced by sortActorsOnCriticality().

void LoadBalanceBinding::getConstantsTileCostFunction | ( | double & | a, | |
double & | b, | |||
double & | c, | |||
double & | d, | |||
double & | e, | |||
double & | f, | |||
double & | g, | |||
double & | k, | |||
double & | l, | |||
double & | m, | |||
double & | n, | |||
double & | o, | |||
double & | p, | |||
double & | q | |||
) |
void LoadBalanceBinding::increaseLoadTile | ( | TimedSDFactor * | a, | |
Tile * | t | |||
) | [private] |
increaseLoadTile () Add the load of an actor to a tile. The load is defined as the execution time of the actor per firing times the number of firings required per period.
References actorLoadOnTile(), ArchComponent::getId(), and tileLoad.
Referenced by allocateResources().

void LoadBalanceBinding::initTileLoad | ( | ) | [private] |
initTileLoad () Initially all tiles are not loaded.
References Binding::archGraph, PlatformGraph::nrTiles(), and tileLoad.
Referenced by bindingCheck(), and bindSDFGtoTiles().

bool LoadBalanceBinding::isActorBound | ( | const SDFactor * | a | ) | const [private] |
isActorBound () The function returns true if an actor is bound to a tile. Otherwise it returns false.
References actorTileBinding, and SDFcomponent::getId().

bool LoadBalanceBinding::isChannelBound | ( | const SDFchannel * | c | ) | const [private] |
isChannelBound () The function returns true if a channel is both the source and destination actor are bound to the a tile. Otherwise it returns false.
References actorTileBinding, SDFchannel::getDstActor(), SDFcomponent::getId(), and SDFchannel::getSrcActor().
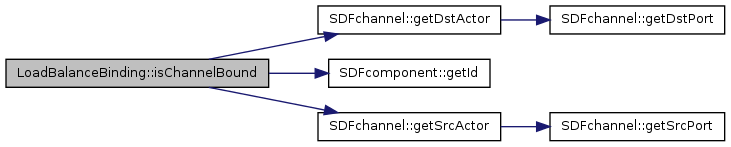
bool LoadBalanceBinding::isChannelBoundToConnection | ( | const SDFchannel * | c | ) | const [private] |
isChannelBoundToConnection () The function returns true if a channel is both the source and destination actor are bound to the different tiles. Otherwise it returns false.
References actorTileBinding, SDFchannel::getDstActor(), SDFcomponent::getId(), and SDFchannel::getSrcActor().
Referenced by allocateResources(), and releaseResources().

bool LoadBalanceBinding::isChannelBoundToTile | ( | const SDFchannel * | c | ) | const [private] |
isChannelBoundToTile () The function returns true if a channel is both the source and destination actor are bound to the same tile. Otherwise it returns false.
References actorTileBinding, SDFchannel::getDstActor(), SDFcomponent::getId(), and SDFchannel::getSrcActor().
Referenced by allocateResources(), releaseResources(), and updateStorageSpaceAllocation().

memLoadChannelsOnTile () The function returns the memory size needed to map all channels connected to actor a when the actor is mapped to tile t. The size of a channel is only considered when the other actor which is connected to the channel is already mapped to a tile.
References actorTileBinding, c, SDFport::getActor(), TimedSDFchannel::getBufferSize(), SDFport::getChannel(), ArchComponent::getId(), SDFcomponent::getId(), TimedSDFchannel::getTokenSize(), SDFport::getType(), SDFchannel::oppositePort(), SDFactor::portsBegin(), and SDFactor::portsEnd().
Referenced by sortTilesOnLoad().
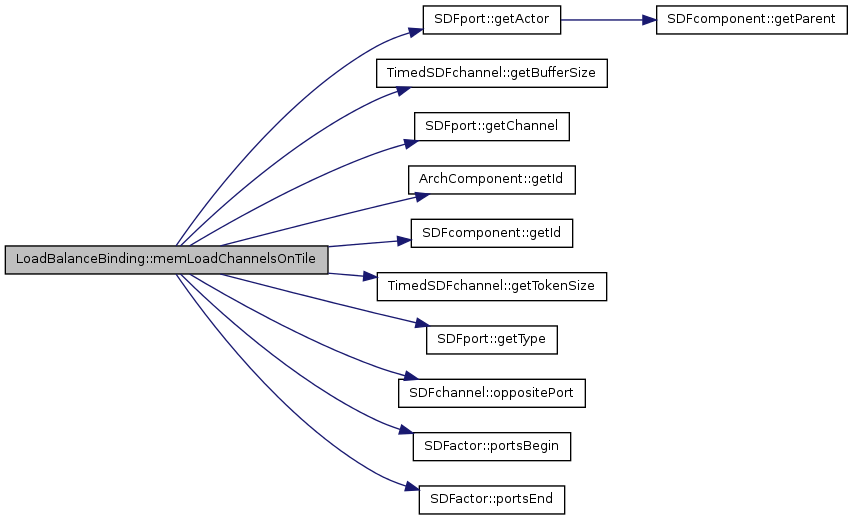
void LoadBalanceBinding::minimizeStorageSpace | ( | ) | [private] |
minimizeStorageSpace () The function computes the minimal buffer allocations needed to meet the throughput constraint. Resource allocations are adjusted to this minimal storage space.
References SDFstateSpaceBindingAwareBufferAnalysis::analyze(), Binding::appGraph, Binding::archGraph, c, _StorageDistributionSet::distributions, SDFgraph::getChannel(), Binding::getFlowType(), TimedSDFgraph::getThroughputConstraint(), TimedSDFchannel::modelsStorageSpace(), _StorageDistributionSet::next, SDFgraph::nrChannels(), _StorageDistributionSet::thr, updateStorageSpaceAllocation(), and CFraction::value().
Referenced by optimizeStorageSpaceAllocations().
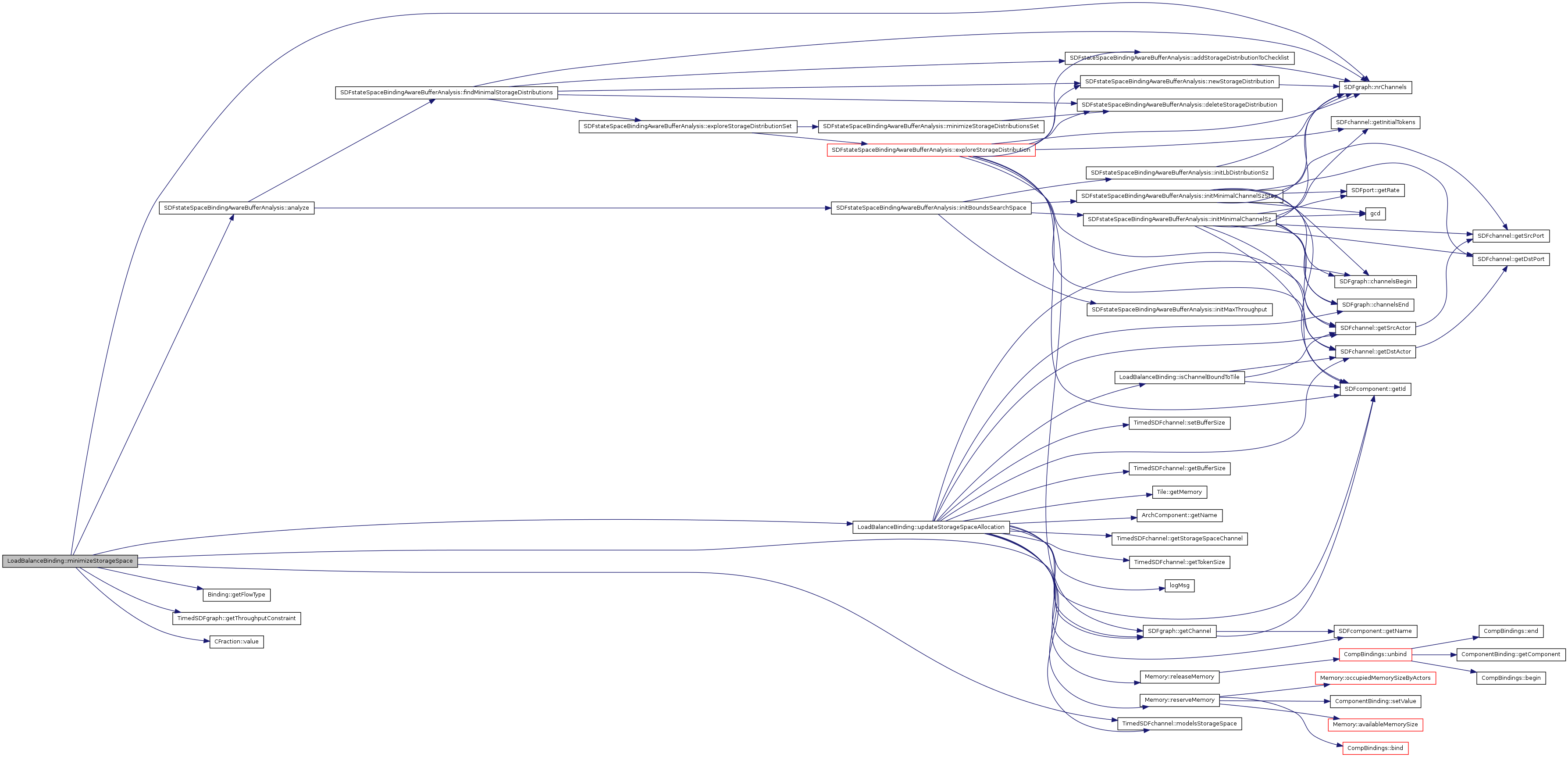
bool LoadBalanceBinding::minimizeTimeSlices | ( | double | step, | |
const double | minStep | |||
) | [private] |
minimizeTimeSlices () The function minimizes the time slice allocation of all used tiles. The objective is to find the minimal time slices with which the throughput constraint is met. The function uses a binary search for this problem.
References Binding::analyzeThroughput(), Binding::appGraph, Binding::archGraph, Processor::availableTimewheelSize(), ArchComponent::getId(), ArchComponent::getName(), Tile::getProcessor(), Processor::getReservedTimeSlice(), TimedSDFgraph::getThroughputConstraint(), Processor::getTimewheelSize(), PlatformGraph::nrTiles(), printTimer(), Processor::reserveTimeSlice(), secondsElapsed(), startTimer(), stopTimer(), tileLoad, PlatformGraph::tilesBegin(), PlatformGraph::tilesEnd(), and CFraction::value().
Referenced by allocateTDMAtimeSlices().
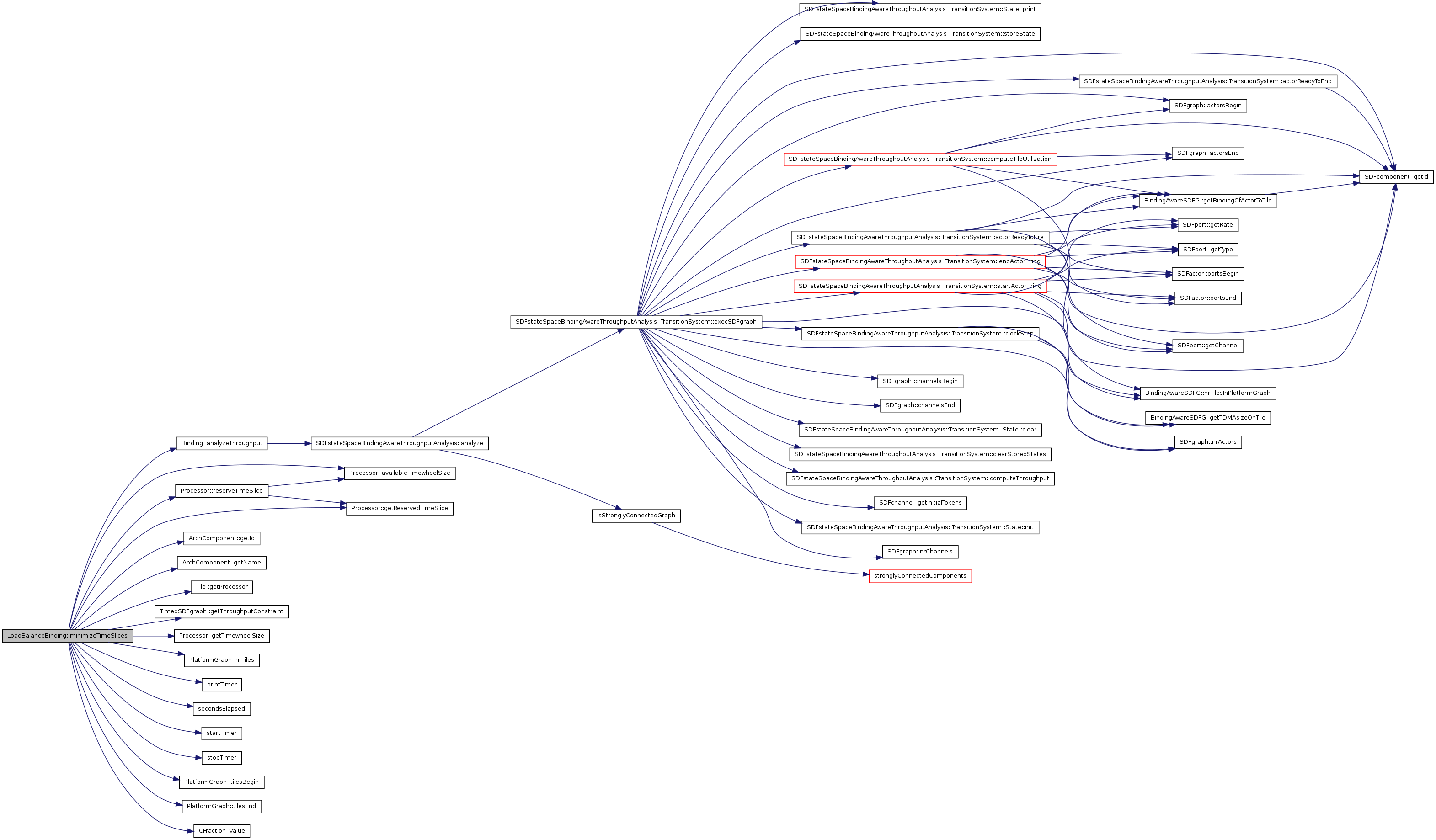
bool LoadBalanceBinding::moveActorBinding | ( | TimedSDFactor * | a, | |
bool | allowExistingTile | |||
) | [private] |
moveActorBinding () The function moves an actor from one processor to another processor. This operation invalidates the schedules of the involved processors.
References actorTileBinding, allocateResources(), Binding::archGraph, cnst_a, cnst_b, cnst_c, cnst_d, cnst_e, cnst_f, cnst_g, cnst_k, cnst_l, cnst_m, cnst_n, cnst_o, cnst_p, cnst_q, ArchComponent::getId(), SDFcomponent::getId(), ArchComponent::getName(), SDFcomponent::getName(), Tile::getProcessor(), Processor::getSchedule(), PlatformGraph::getTiles(), releaseResources(), and sortTilesOnLoad().
Referenced by optimizeActorToTileBindings().
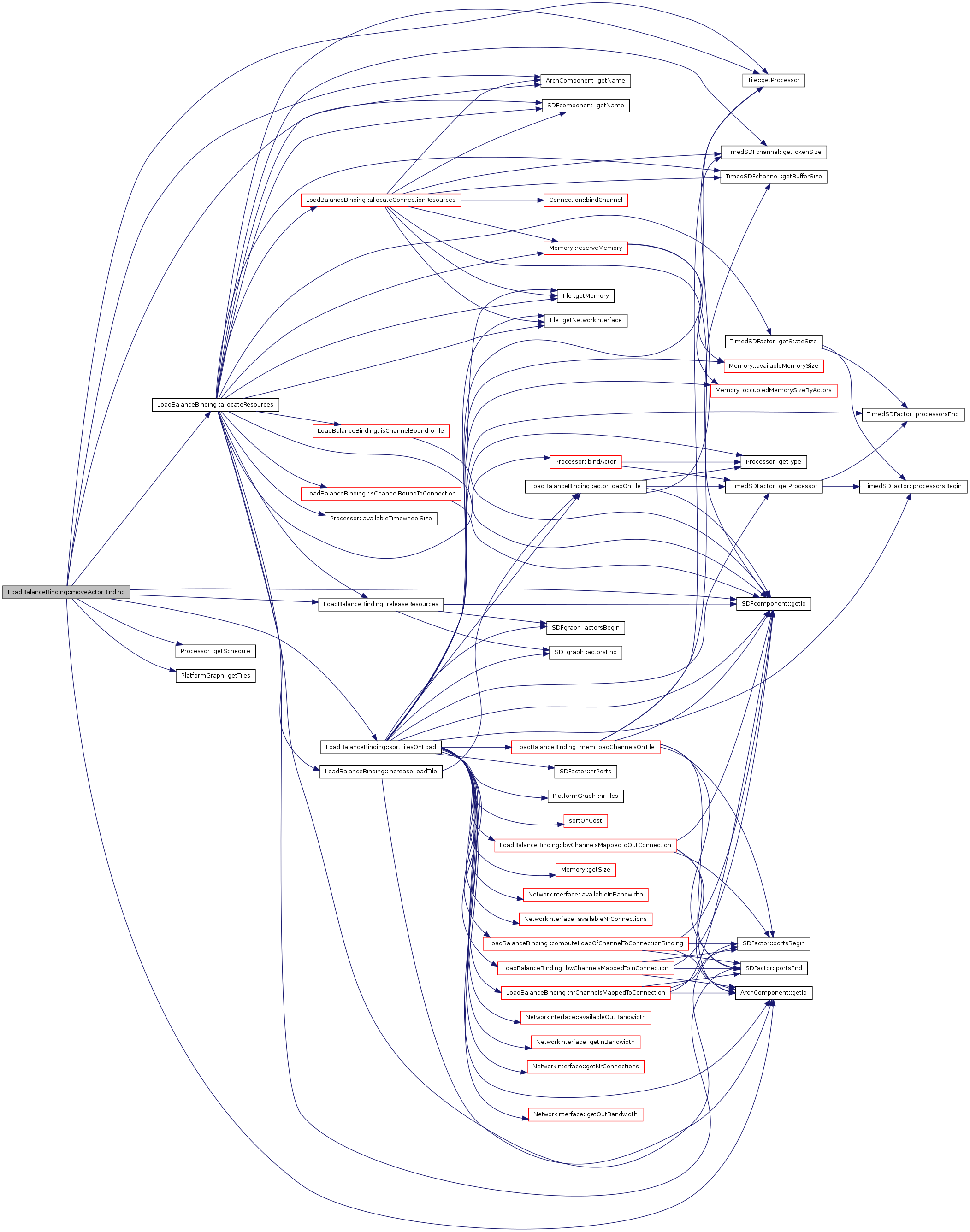
nrChannelsMappedToConnection () The function returns the number of channels connected to the actor a which will be mapped to a connection if the actor is mapped to tile t. Channels of which the other actor is not mapped are ignored.
References actorTileBinding, c, SDFport::getActor(), SDFport::getChannel(), ArchComponent::getId(), SDFcomponent::getId(), SDFchannel::oppositePort(), SDFactor::portsBegin(), and SDFactor::portsEnd().
Referenced by sortTilesOnLoad().
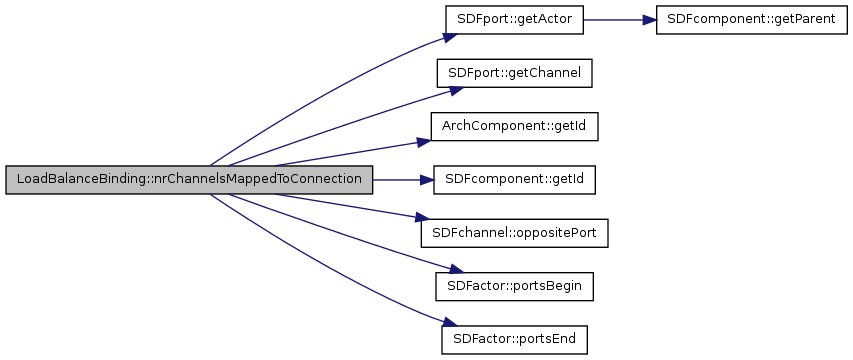
void LoadBalanceBinding::optimizeActorToTileBindings | ( | ) | [private] |
optimizeActorToTileBindings () The function moves actors between tiles to achieve an optimal load balance. It considers both computation and communication cost when moving actors.
References a, actorTileBinding, Binding::appGraph, SDFgraph::getActors(), ArchComponent::getId(), SDFcomponent::getId(), maxCycleMean, moveActorBinding(), SDFgraph::nrActors(), sortOnCost(), and tileLoad.
Referenced by bindSDFGtoTiles().
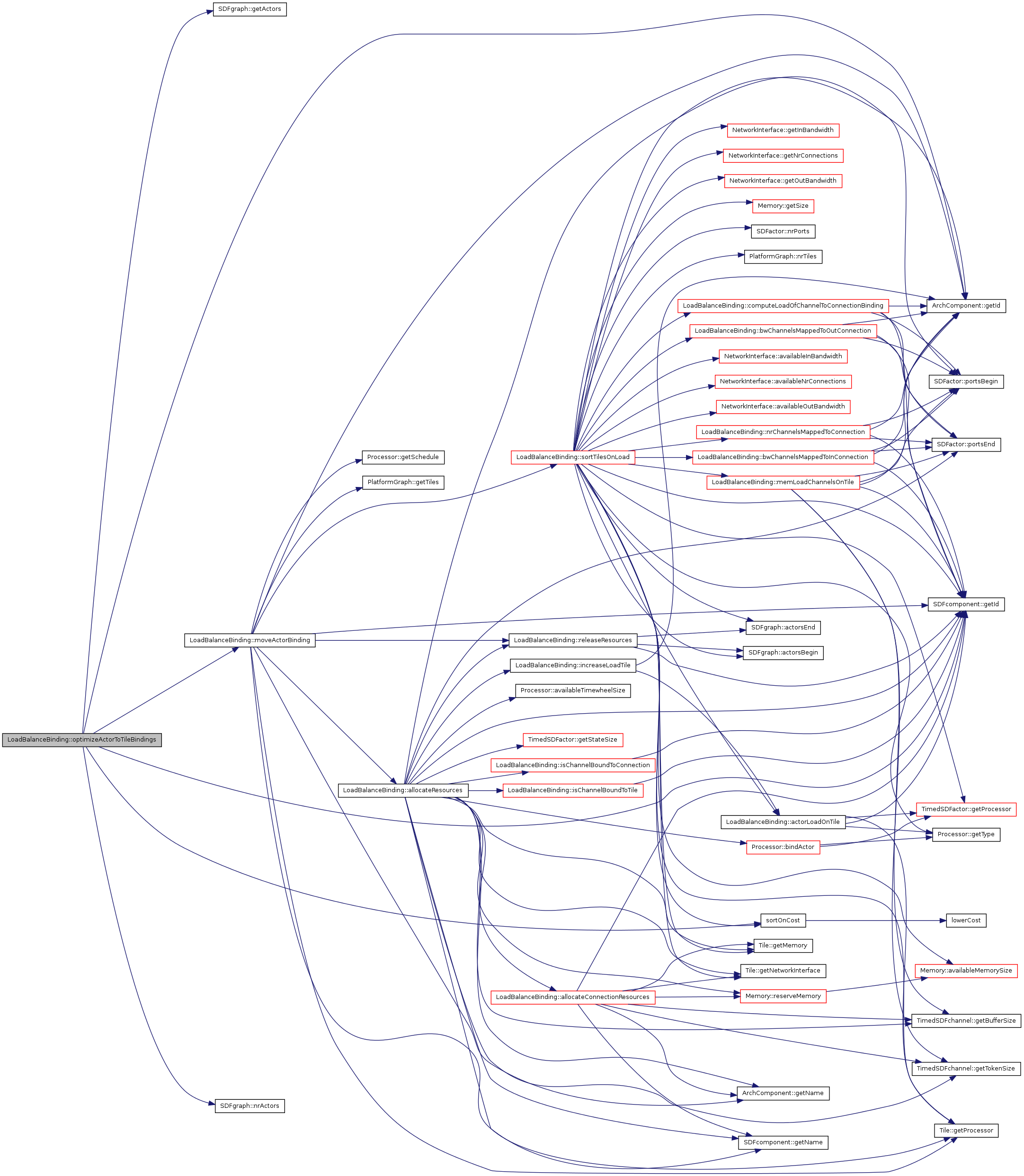
bool LoadBalanceBinding::optimizeStorageSpaceAllocations | ( | ) | [virtual] |
optimizeStorageSpaceAllocations ()
Implements Binding.
References minimizeStorageSpace().
Referenced by bind().
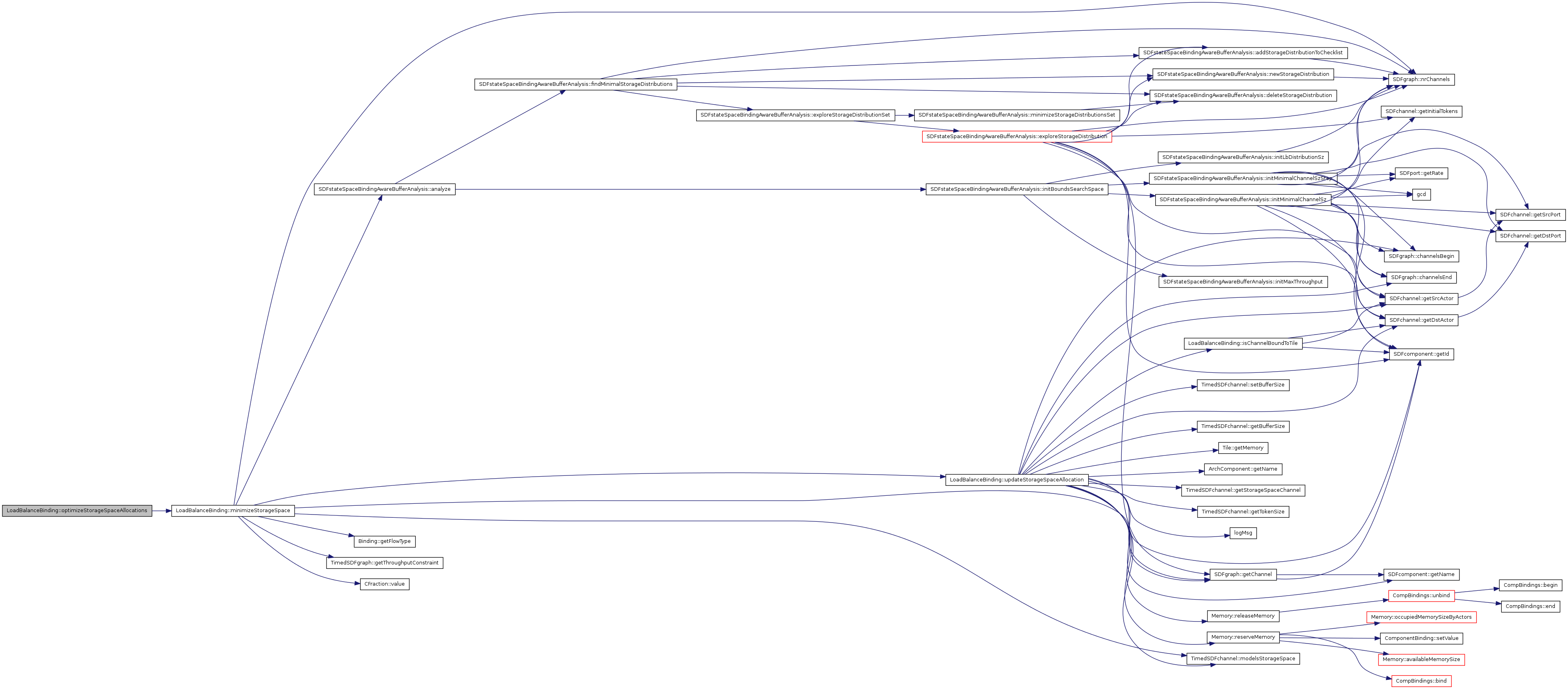
void LoadBalanceBinding::optimizeTimeSlices | ( | ) | [private] |
optimizeTimeSlices () The function performs a binary search to minimize the time slice allocated on each tile.
References Binding::archGraph, ArchComponent::getId(), ArchComponent::getName(), Tile::getProcessor(), Processor::getReservedTimeSlice(), Processor::getTimewheelSize(), PlatformGraph::nrTiles(), Processor::reserveTimeSlice(), tileLoad, PlatformGraph::tilesBegin(), and PlatformGraph::tilesEnd().
Referenced by allocateTDMAtimeSlices(), and optimizeTimeSlices().
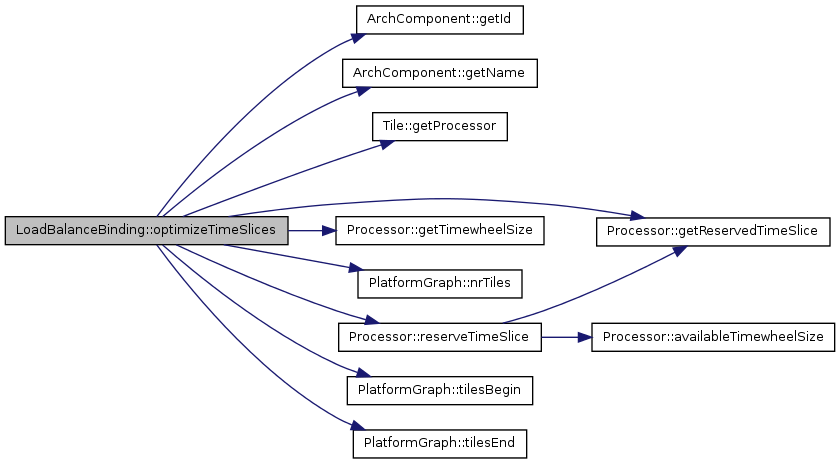
bool LoadBalanceBinding::optimizeTimeSlices | ( | vector< CSize > | minSlice, | |
vector< CSize > | maxSlice | |||
) | [private] |
optimizeTimeSlices () The function performs a binary search to minimize the time slice allocated on each tile.
References Binding::analyzeThroughput(), Binding::appGraph, Binding::archGraph, ArchComponent::getId(), ArchComponent::getName(), Tile::getProcessor(), Processor::getReservedTimeSlice(), TimedSDFgraph::getThroughputConstraint(), Processor::getTimewheelSize(), PlatformGraph::nrTiles(), optimizeTimeSlices(), printTimer(), Processor::reserveTimeSlice(), startTimer(), stopTimer(), tileLoad, PlatformGraph::tilesBegin(), PlatformGraph::tilesEnd(), and CFraction::value().
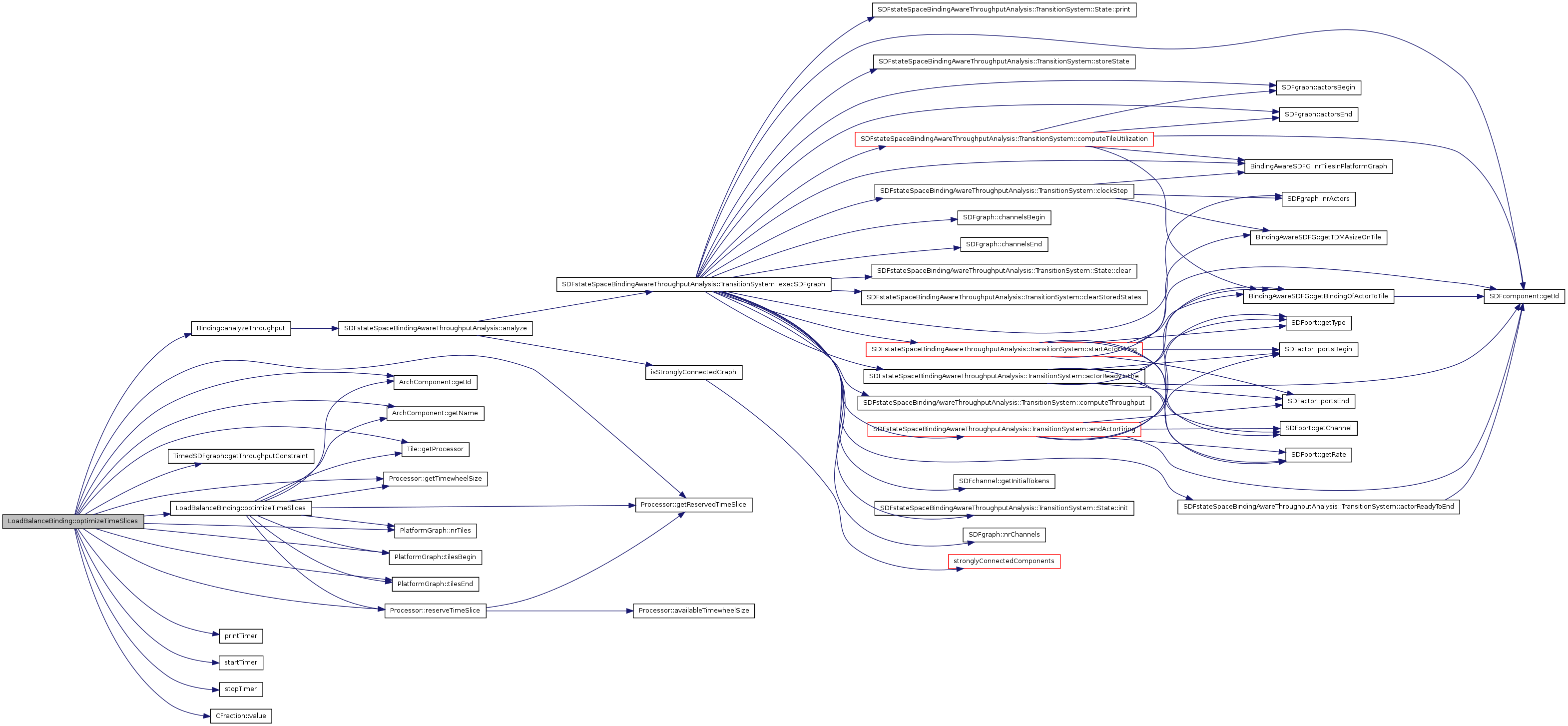
void LoadBalanceBinding::reconstructStaticOrderSchedules | ( | ) | [private] |
reconstructStaticOrderSchedules () The function generates a static-order schedule for each processor in the architecture graph. The schedules order the firing of the actors in the application graph. They respects the buffer requirements and mapping of all actors.
References Binding::appGraph, Binding::archGraph, StaticOrderSchedule::changeActorAssociations(), Binding::getFlowType(), ArchComponent::getName(), Tile::getProcessor(), Processor::getSchedule(), BindingAwareSDFG::getScheduleOnTile(), PlatformGraph::getTile(), logMsg(), BindingAwareSDFG::nrTilesInPlatformGraph(), SDFstateSpaceListScheduler::schedule(), Processor::setSchedule(), PlatformGraph::tilesBegin(), and PlatformGraph::tilesEnd().
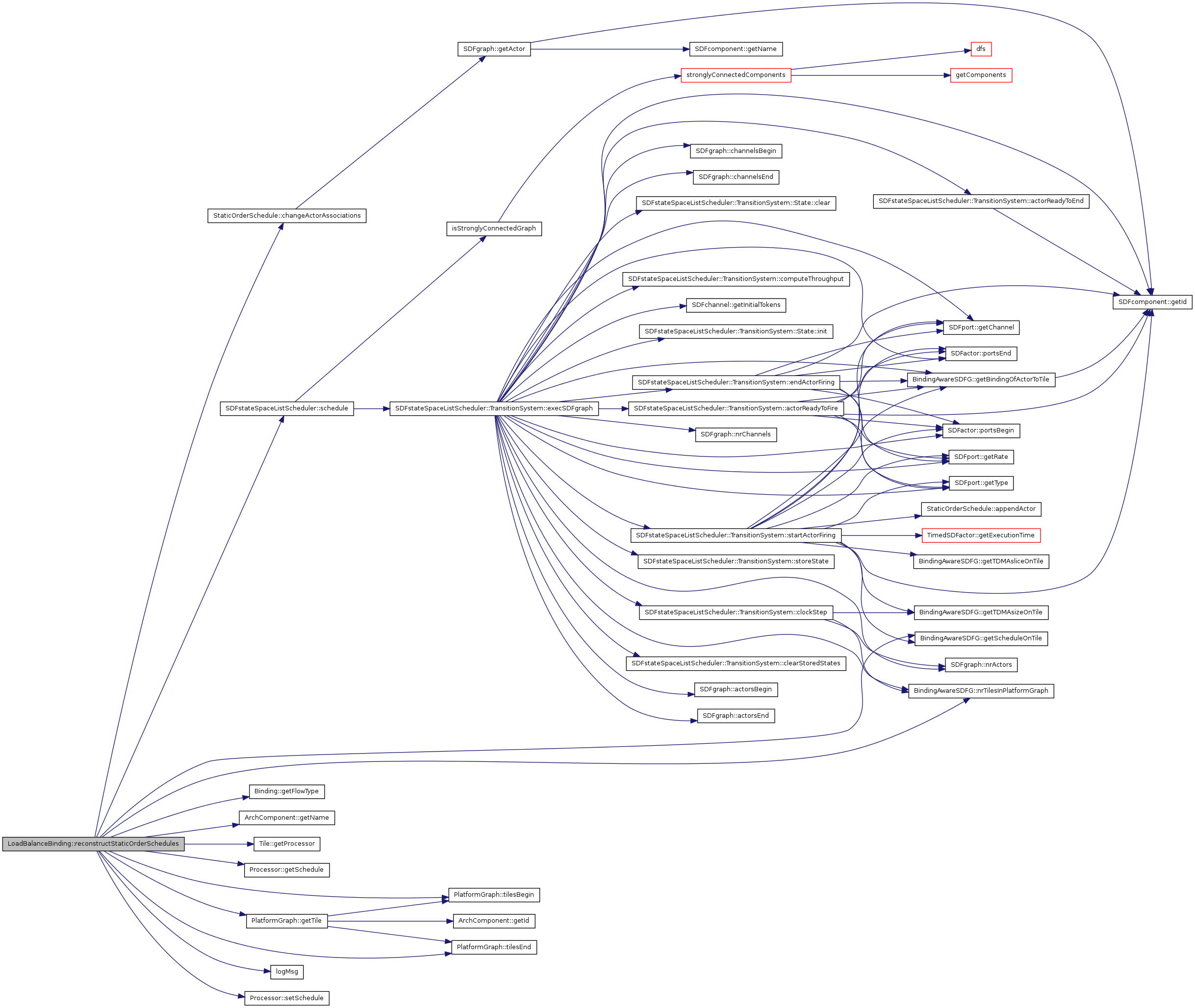
void LoadBalanceBinding::releaseConnectionResources | ( | TimedSDFchannel * | c | ) | [private] |
releaseConnectionResources () The function releases all connection resources allocated by a channel.
References actorTileBinding, Binding::archGraph, PlatformGraph::getConnections(), SDFchannel::getDstActor(), SDFcomponent::getId(), Tile::getMemory(), ArchComponent::getName(), SDFcomponent::getName(), Tile::getNetworkInterface(), SDFchannel::getSrcActor(), NetworkInterface::releaseConnection(), Memory::releaseMemory(), and Connection::unbindChannel().
Referenced by releaseResources().
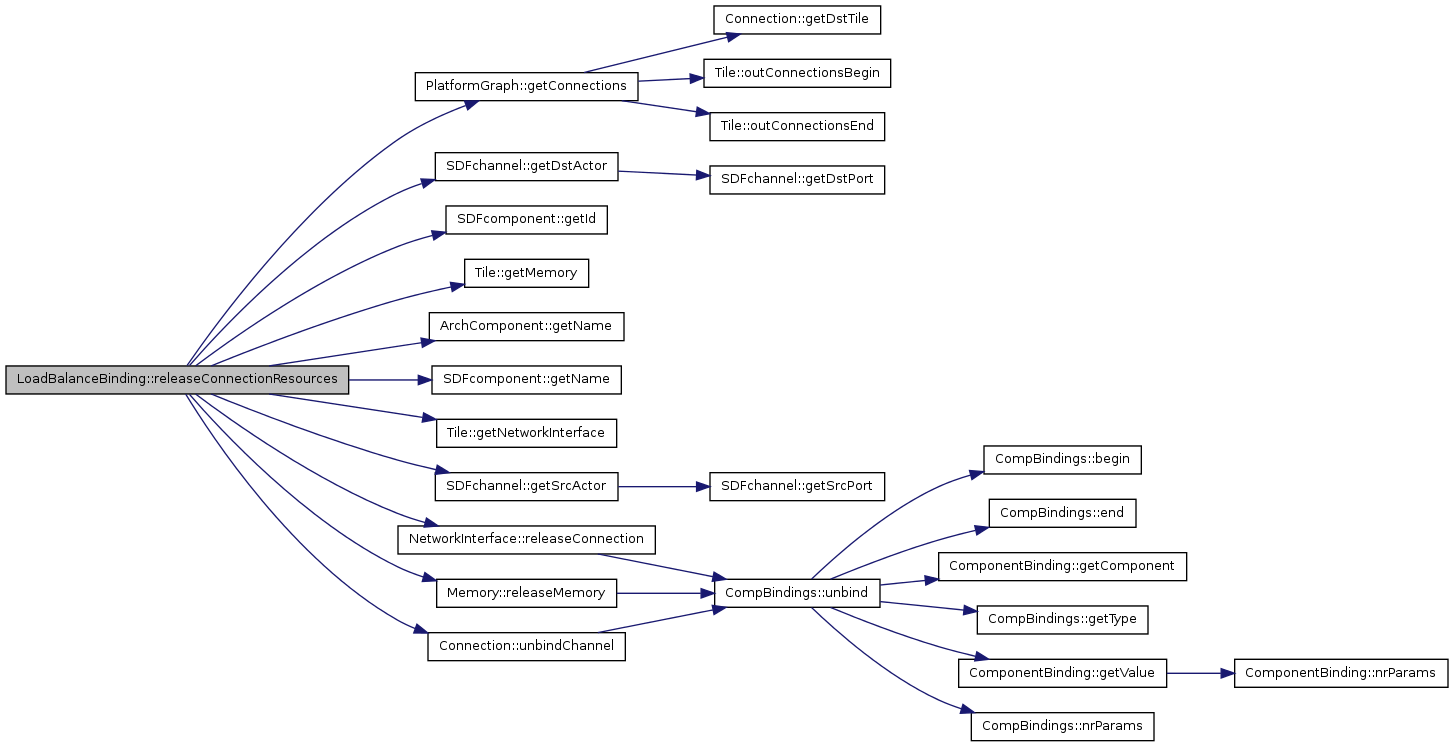
void LoadBalanceBinding::releaseResources | ( | TimedSDFactor * | a, | |
Tile * | t | |||
) | [private] |
releaseResources () The function releases all resources allocated by an actor on a tile t. This includes possible connections to other actors.
References actorTileBinding, decreaseLoadTile(), SDFport::getChannel(), SDFcomponent::getId(), Tile::getMemory(), ArchComponent::getName(), SDFcomponent::getName(), Tile::getProcessor(), isChannelBoundToConnection(), isChannelBoundToTile(), SDFactor::portsBegin(), SDFactor::portsEnd(), releaseConnectionResources(), Memory::releaseMemory(), Processor::releaseTimeSlice(), and Processor::unbindActor().
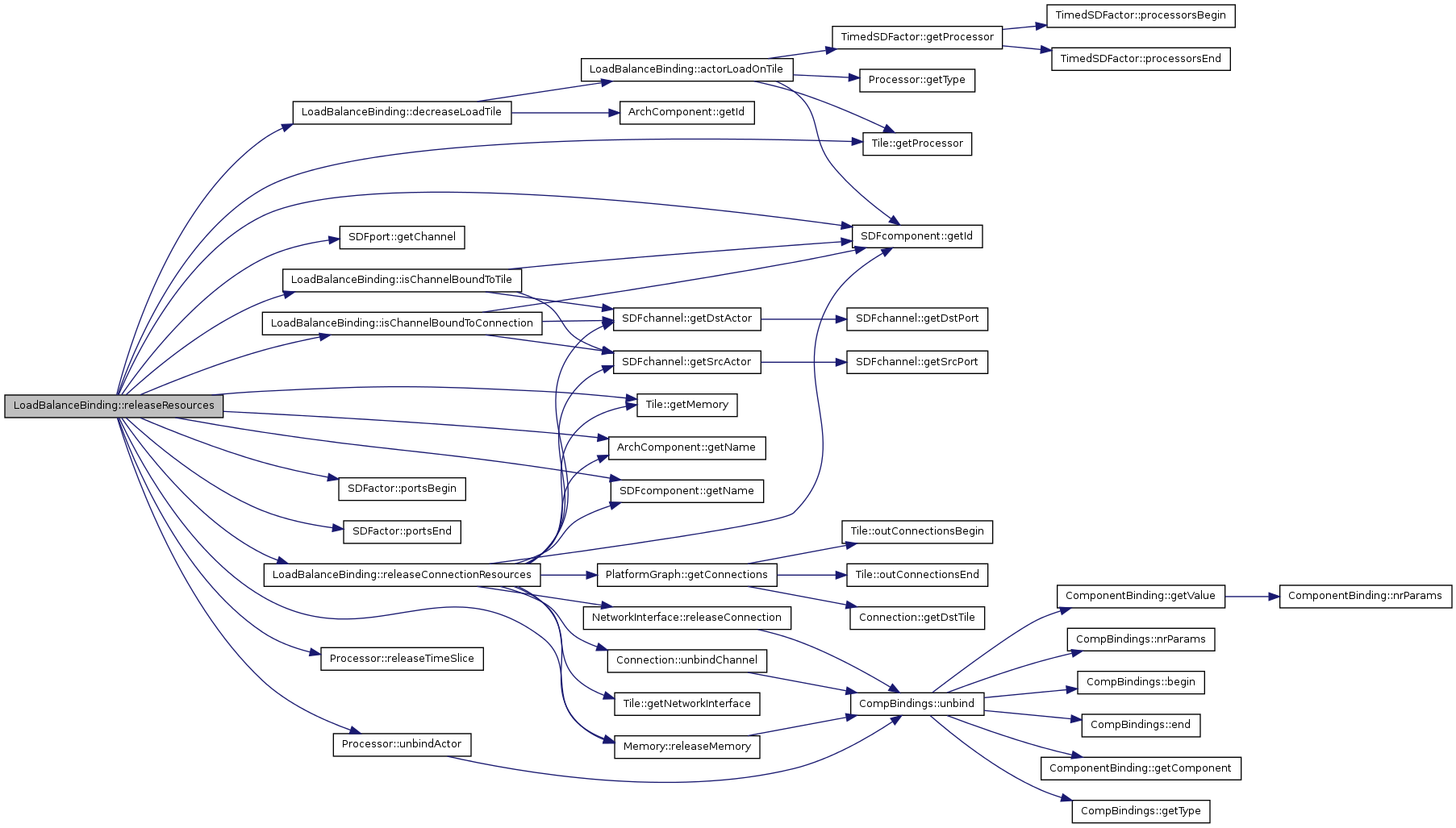
void LoadBalanceBinding::releaseResources | ( | ) | [private, virtual] |
releaseResources () The function releases all resources allocated by a application.
Implements Binding.
References a, SDFgraph::actorsBegin(), SDFgraph::actorsEnd(), actorTileBinding, Binding::appGraph, and SDFcomponent::getId().
Referenced by allocateResources(), allocateTDMAtimeSlices(), bindSDFGtoTiles(), and moveActorBinding().
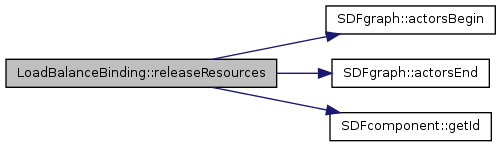
void LoadBalanceBinding::releaseTimeSlices | ( | ) | [private] |
releaseTimeSlices () The function releases the complete TDMA time slice it has allocated on each tile.
References Binding::archGraph, ArchComponent::getName(), Tile::getProcessor(), Processor::releaseTimeSlice(), PlatformGraph::tilesBegin(), and PlatformGraph::tilesEnd().
Referenced by constructStaticOrderSchedules().
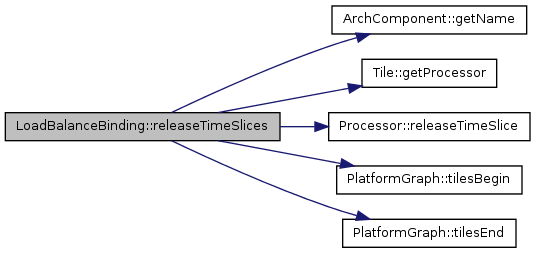
void LoadBalanceBinding::reserveTimeSlices | ( | double | fraction | ) | [private] |
reserveTimeSlices () The function reserves a TDMA time slice of fraction of the available wheel size on each tile which contains actors of the application graph.
References Binding::archGraph, ASSERT, Processor::availableTimewheelSize(), ArchComponent::getId(), ArchComponent::getName(), Tile::getProcessor(), Processor::getReservedTimeSlice(), Processor::getTimewheelSize(), Processor::reserveTimeSlice(), tileLoad, PlatformGraph::tilesBegin(), and PlatformGraph::tilesEnd().
Referenced by allocateTDMAtimeSlices(), bindingCheck(), and constructStaticOrderSchedules().
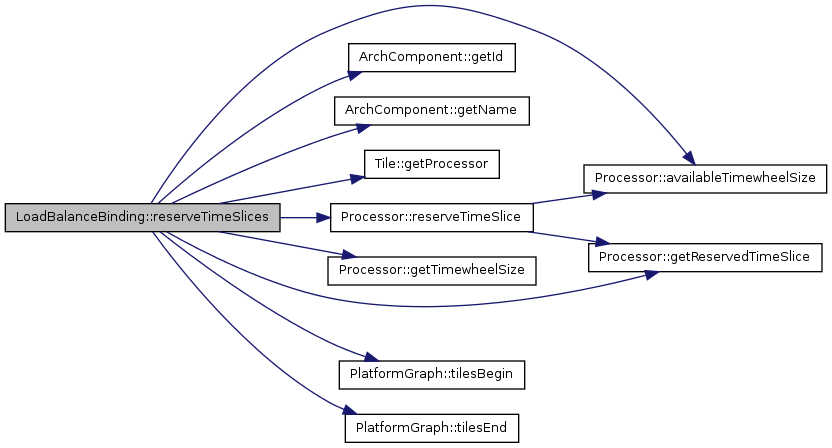
void LoadBalanceBinding::setAppGraph | ( | TimedSDFgraph * | g | ) | [virtual] |
setAppGraph () Set the application graph. This function is overloaded from the base class.
Implements Binding.
References actorTileBinding, Binding::appGraph, FSMSADF::computeRepetitionVector(), maxCycleMean, SDFgraph::nrActors(), and repVec.
Referenced by bindApplicationGraphsToArchitectureGraph().

void LoadBalanceBinding::setConstantsTileCostFunction | ( | double | a, | |
double | b, | |||
double | c, | |||
double | d, | |||
double | e, | |||
double | f, | |||
double | g, | |||
double | k, | |||
double | l, | |||
double | m, | |||
double | n, | |||
double | o, | |||
double | p, | |||
double | q | |||
) |
setConstantsTileCostFunction () The function sets all constants used in the tile cost function.
References cnst_a, cnst_b, cnst_c, cnst_d, cnst_e, cnst_f, cnst_g, cnst_k, cnst_l, cnst_m, cnst_n, cnst_o, cnst_p, and cnst_q.
Referenced by bindApplicationGraphsToArchitectureGraph(), and LoadBalanceBinding().
SDFactors LoadBalanceBinding::sortActorsOnCriticality | ( | ) | [private] |
sortActorsOnCriticality () The function computes for each actor the maximum cycle mean of all simple cycles it is part of. The cycle mean is defined as the sum of the execution times of the actors (in one period) divided by the normalized initial tokens on the edges of the cycle. The function orders the actors according to their maximum cycle mean (high to low).
References Binding::appGraph, estimateMaxCycleMean(), SDFgraph::getActors(), maxCycleMean, and sortOnCost().
Referenced by bindActorsToTiles().
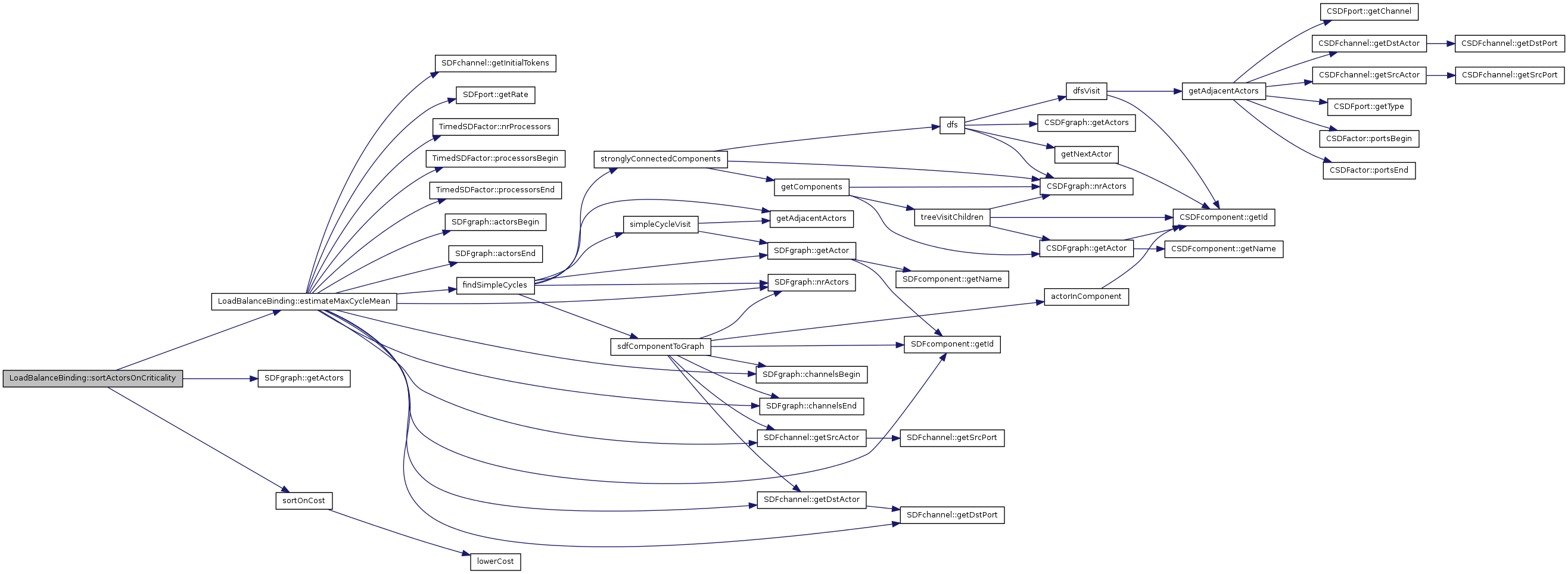
void LoadBalanceBinding::sortTilesOnCommunicationOverhead | ( | TimedSDFactor * | a, | |
Tiles & | tiles | |||
) | [private] |
sortTilesOnCommunicationOverhead () The function takes as input a list of tiles ordered based on the processor load and an actor when can be mapped to all tiles in the list. The functions considers reordering the tiles based on the expected communication overhead (connection delay + timewheel synchronization). When the communication overhead of mapping actor a to tile t1 exceeds the gain of mapping actor a onto a different tile t2, the tiles t1 and t2 are reordered.
References actorTileBinding, Binding::appGraph, Binding::archGraph, c, SDFport::getActor(), SDFport::getChannel(), PlatformGraph::getConnection(), TimedSDFactor::getExecutionTime(), SDFcomponent::getId(), ArchComponent::getId(), Connection::getLatency(), TimedSDFchannel::getMinBandwidth(), TimedSDFactor::getProcessor(), Tile::getProcessor(), SDFport::getRate(), TimedSDFchannel::getTokenSize(), SDFport::getType(), Processor::getType(), SDFgraph::nrActors(), PlatformGraph::nrTiles(), SDFchannel::oppositePort(), SDFactor::portsBegin(), SDFactor::portsEnd(), repVec, sortOnCost(), and tileLoad.
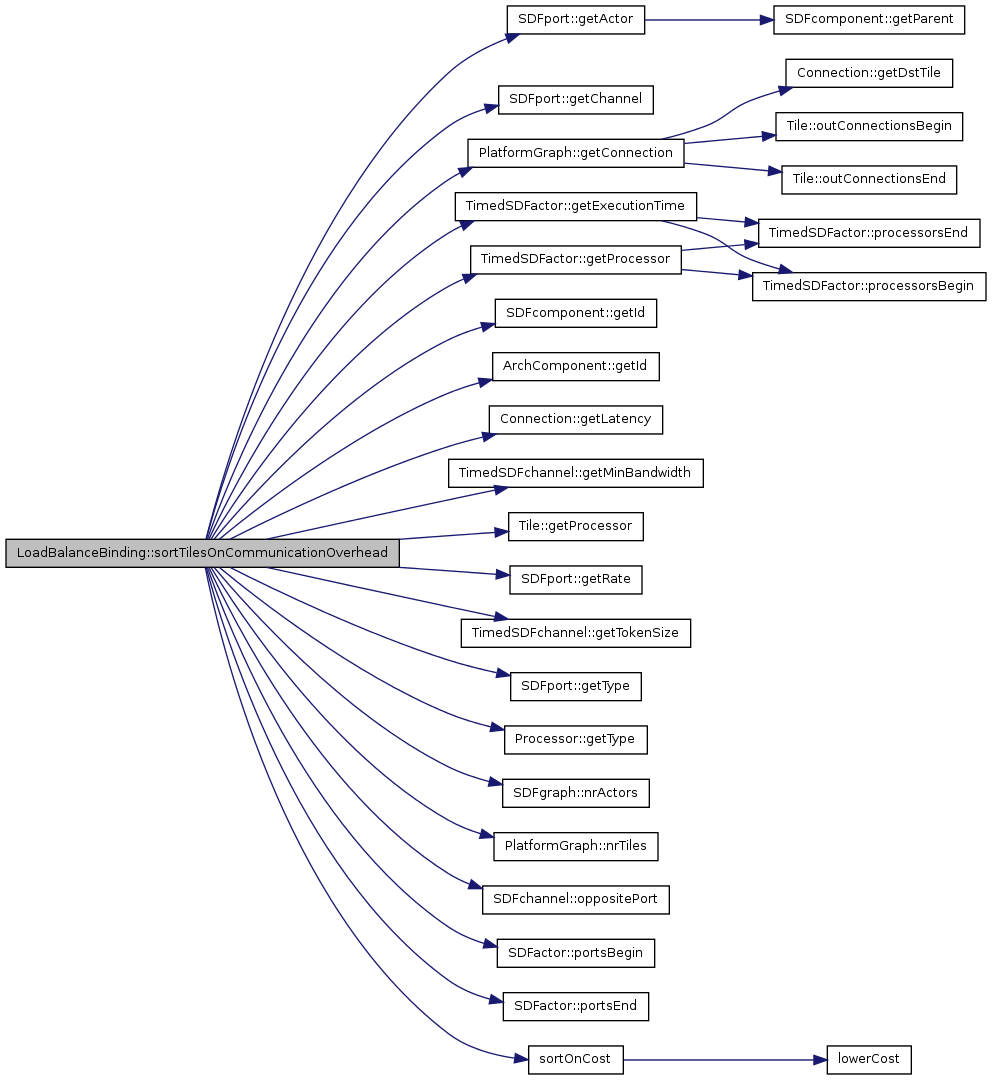
void LoadBalanceBinding::sortTilesOnLoad | ( | TimedSDFactor * | a, | |
Tiles & | tiles, | |||
double | const_a, | |||
double | const_b, | |||
double | const_c, | |||
double | const_d, | |||
double | const_e, | |||
double | const_f, | |||
double | const_g, | |||
double | const_k, | |||
double | const_l, | |||
double | const_m, | |||
double | const_n, | |||
double | const_o, | |||
double | const_p, | |||
double | const_q | |||
) | [private] |
sortTilesOnLoad () The function sorts the list of tiles from a low to a high load to which actor a can be mapped.
Cost of a tile is determined by:
- the processor load measured in execution time of the mapped actors per iteration of the graph,
- the memory requirements of the mapped actors,
- the memory requirements of the mapped communication channels,
- the bandwidth requirements of the mapped communication channels,
- the number of mapped communication channels,
- the number of additional connections required when mapping the actor to the tile.
When computing the cost of a tile, while considering the mapping of an actor to the tile, the actor is considered to be mapped to the tile. It is only checked wether the processor type inside the tile is supported by the actor.
The cost of a tile t is defined as:
cost(t) = a*ProcLoad^k + b*(MemAct+MemCh)^l + c*Bw^m + d*NrConn^n + e*NrNewConn^o + g*latencyConn^q
The constants a, b, c, d, e are used to scale the various properties determining the cost wrt each other. The constants k, l, m, n, o are used to increase cost for heavily loaded tiles.
References actorLoadOnTile(), SDFgraph::actorsBegin(), SDFgraph::actorsEnd(), actorTileBinding, Binding::appGraph, Binding::archGraph, NetworkInterface::availableInBandwidth(), Memory::availableMemorySize(), NetworkInterface::availableNrConnections(), NetworkInterface::availableOutBandwidth(), bwChannelsMappedToInConnection(), bwChannelsMappedToOutConnection(), computeLoadOfChannelToConnectionBinding(), ArchComponent::getId(), SDFcomponent::getId(), NetworkInterface::getInBandwidth(), Tile::getMemory(), Tile::getNetworkInterface(), NetworkInterface::getNrConnections(), NetworkInterface::getOutBandwidth(), TimedSDFactor::getProcessor(), Tile::getProcessor(), Memory::getSize(), Processor::getType(), memLoadChannelsOnTile(), nrChannelsMappedToConnection(), SDFactor::nrPorts(), PlatformGraph::nrTiles(), Memory::occupiedMemorySizeByActors(), TimedSDFactor::processorsBegin(), TimedSDFactor::processorsEnd(), repVec, and sortOnCost().
Referenced by bindActorsToTiles(), and moveActorBinding().
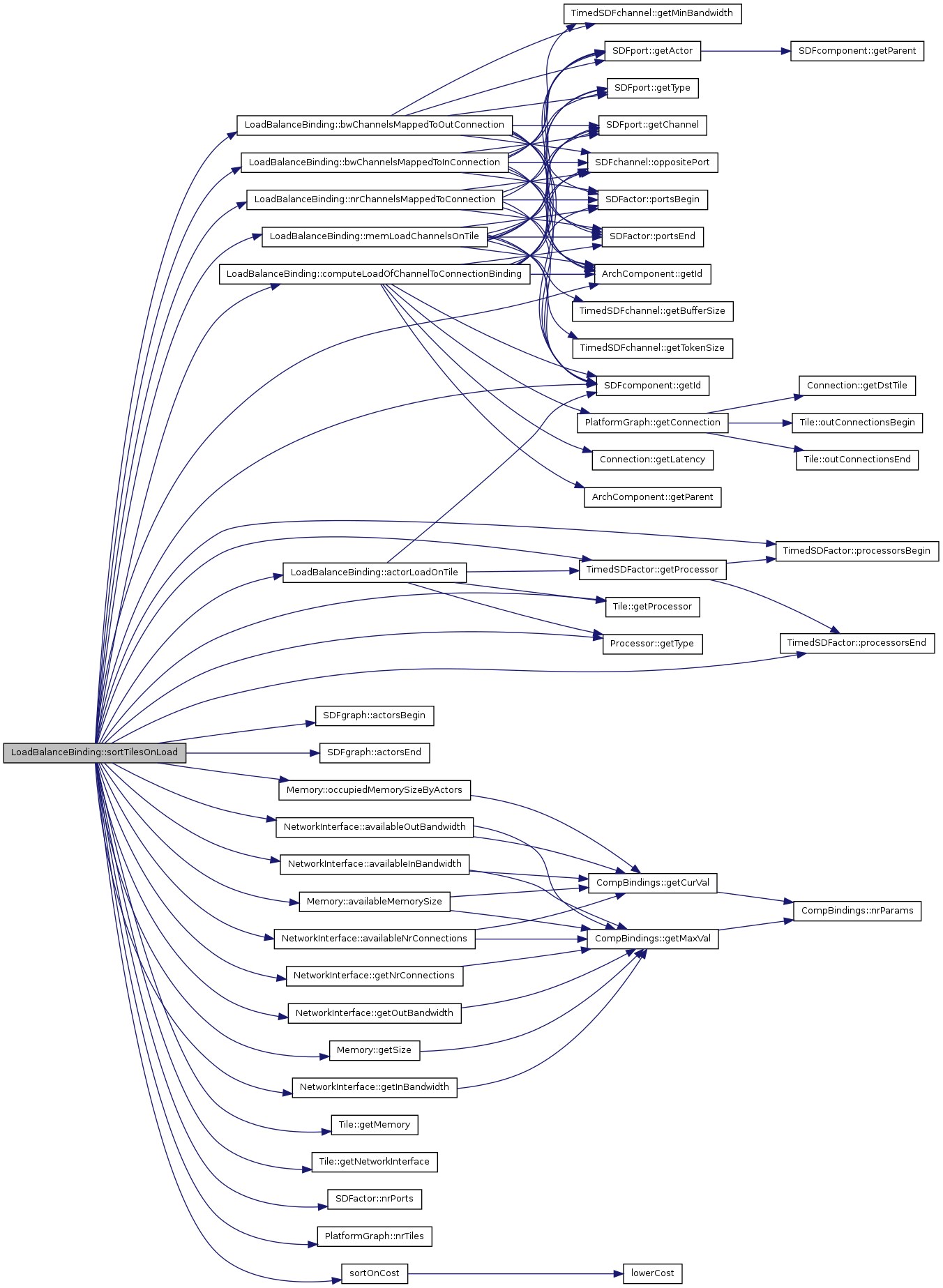
void LoadBalanceBinding::updateStorageSpaceAllocation | ( | TimedSDFgraph * | mappedAppGraph, | |
StorageDistribution * | d | |||
) | [private] |
updateStorageSpaceAllocation () The function changes the storage space allocation of every channel marked as storage space in the mappedAppGraph into the storage space indicated by the storage distribution d.
References actorTileBinding, Binding::appGraph, c, SDFgraph::channelsBegin(), SDFgraph::channelsEnd(), TimedSDFchannel::_BufferSize::dst, TimedSDFchannel::getBufferSize(), SDFgraph::getChannel(), SDFchannel::getDstActor(), SDFcomponent::getId(), Tile::getMemory(), ArchComponent::getName(), SDFcomponent::getName(), SDFchannel::getSrcActor(), TimedSDFchannel::getStorageSpaceChannel(), TimedSDFchannel::getTokenSize(), isChannelBoundToTile(), logMsg(), TimedSDFchannel::_BufferSize::mem, TimedSDFchannel::modelsStorageSpace(), Memory::releaseMemory(), Memory::reserveMemory(), TimedSDFchannel::setBufferSize(), _StorageDistribution::sp, TimedSDFchannel::_BufferSize::src, and TimedSDFchannel::_BufferSize::sz.
Referenced by minimizeStorageSpace().
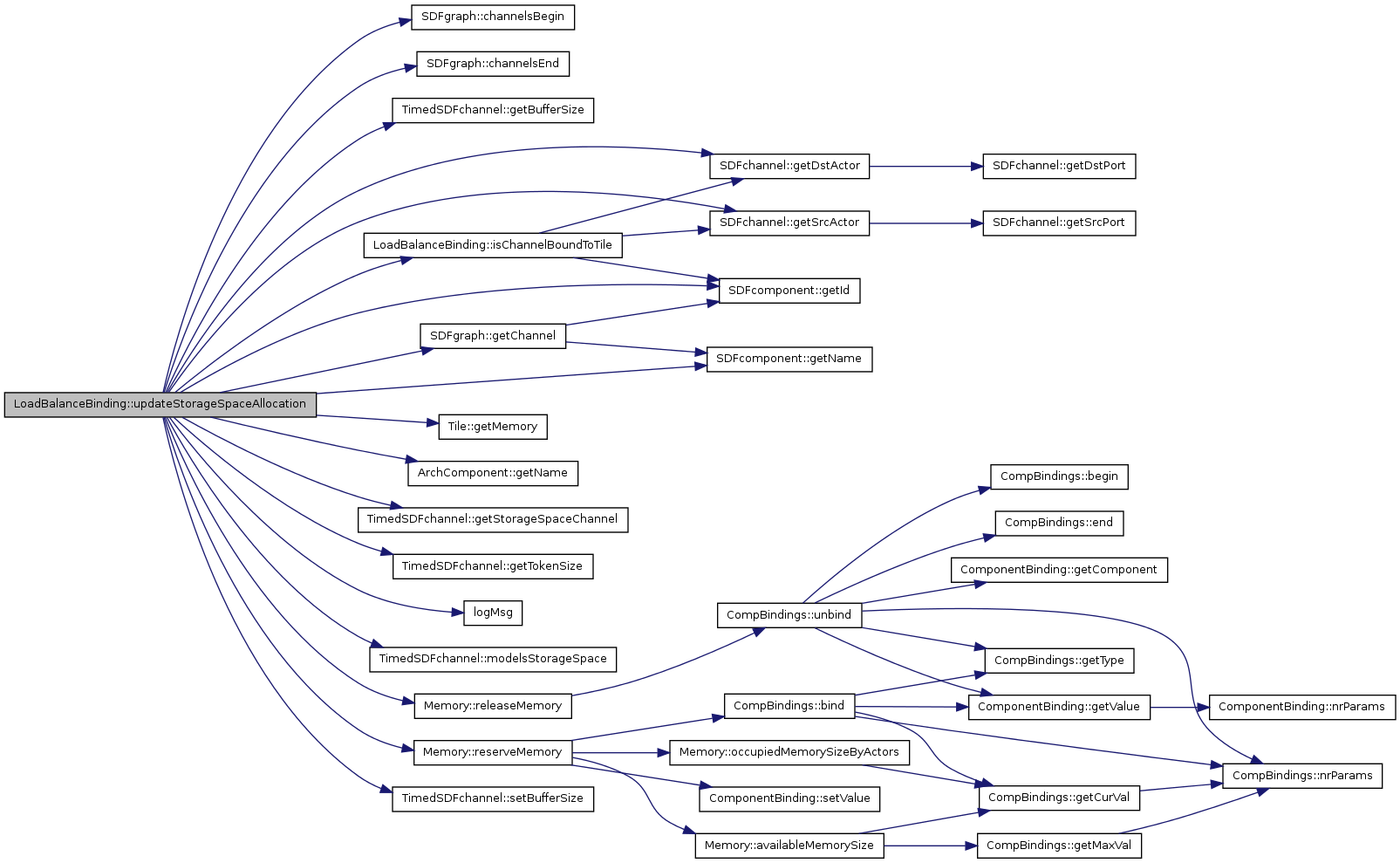
Member Data Documentation
Tiles LoadBalanceBinding::actorTileBinding [private] |
Referenced by allocateConnectionResources(), allocateResources(), allocateTDMAtimeSlices(), bindingCheck(), bindSDFGtoTiles(), bwChannelsMappedToInConnection(), bwChannelsMappedToOutConnection(), changeBandwidthAllocation(), computeLoadOfChannelToConnectionBinding(), isActorBound(), isChannelBound(), isChannelBoundToConnection(), isChannelBoundToTile(), memLoadChannelsOnTile(), moveActorBinding(), nrChannelsMappedToConnection(), optimizeActorToTileBindings(), releaseConnectionResources(), releaseResources(), setAppGraph(), sortTilesOnCommunicationOverhead(), sortTilesOnLoad(), and updateStorageSpaceAllocation().
vector<Tiles> LoadBalanceBinding::actorTileBindingOptions [private] |
double LoadBalanceBinding::cnst_a [private] |
double LoadBalanceBinding::cnst_b [private] |
double LoadBalanceBinding::cnst_c [private] |
double LoadBalanceBinding::cnst_d [private] |
double LoadBalanceBinding::cnst_e [private] |
double LoadBalanceBinding::cnst_f [private] |
double LoadBalanceBinding::cnst_g [private] |
double LoadBalanceBinding::cnst_k [private] |
double LoadBalanceBinding::cnst_l [private] |
double LoadBalanceBinding::cnst_m [private] |
double LoadBalanceBinding::cnst_n [private] |
double LoadBalanceBinding::cnst_o [private] |
double LoadBalanceBinding::cnst_p [private] |
double LoadBalanceBinding::cnst_q [private] |
double* LoadBalanceBinding::maxCycleMean [private] |
RepetitionVector LoadBalanceBinding::repVec [private] |
double* LoadBalanceBinding::tileLoad [private] |
The documentation for this class was generated from the following files: